$http.get(...).success is not a function
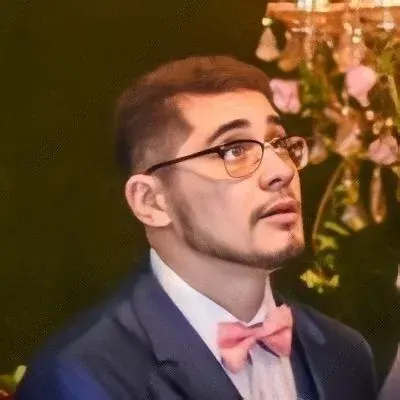
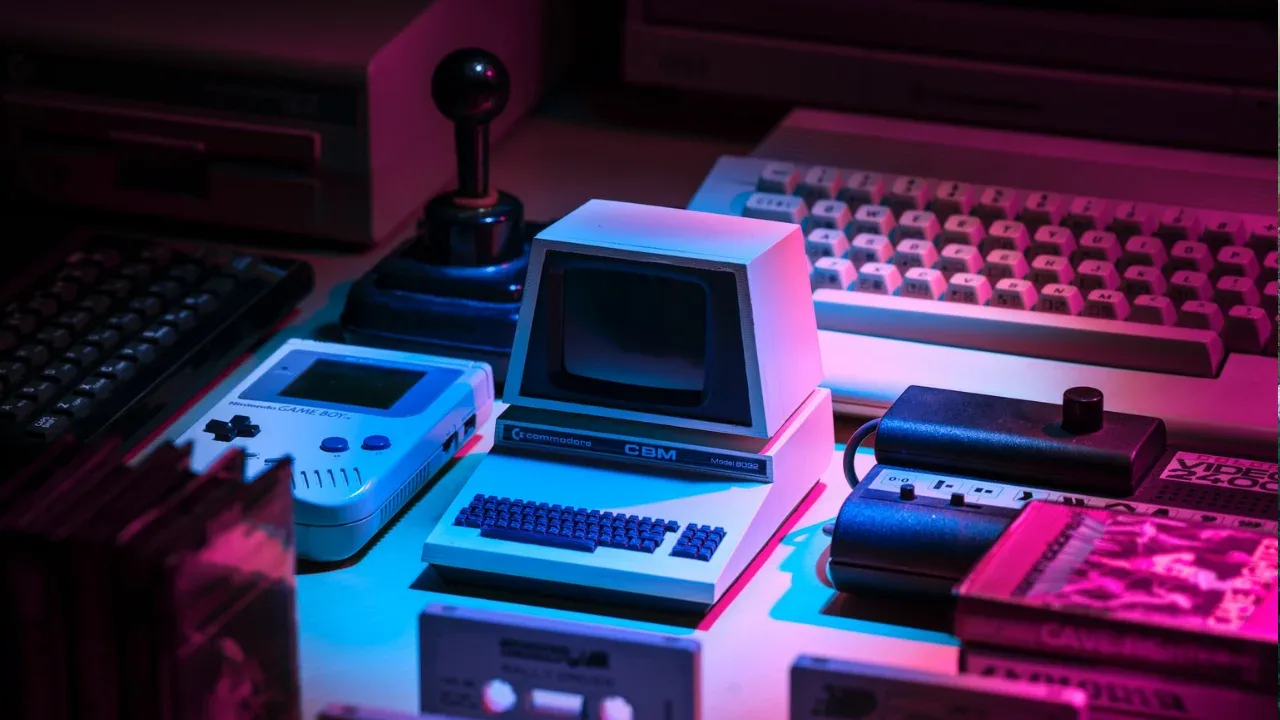
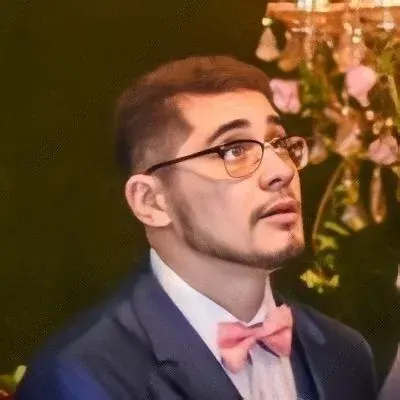
💻📝👋 Hey there tech enthusiasts! Have you ever encountered the error "TypeError: $http.get(...).success is not a function" while working with AngularJS and making an HTTP GET request? 😱 Don't worry, you're not alone, and I'm here to help you fix it! Let's dive in and find a solution together. 🤝
First, let's take a closer look at your code example:
app.controller('MainCtrl', function ($scope, $http){
$http.get('api/url-api')
.success(function (data, status, headers, config){
}
}
The error message suggests that the .success
function is not recognized, which is the root cause of the issue. This is because the .success
function has been deprecated since AngularJS version 1.6, and it was completely removed in AngularJS version 1.7 and above. 😢
But fret not, my friend! AngularJS provides an alternative method called .then
which handles both success and error responses. 💪 Here's how you can modify your code to fix the error:
app.controller('MainCtrl', function ($scope, $http){
$http.get('api/url-api')
.then(function (response){
// Handle the success response here
var data = response.data;
var status = response.status;
var headers = response.headers;
var config = response.config;
})
.catch(function (error){
// Handle the error response here
});
}
In the updated code snippet, we replaced .success
with .then
and added a .catch
block to handle any error that might occur during the HTTP request. 😎
Now, when you run this code, you should be able to successfully handle both the success and error cases. 🎉
🔥 Pro Tip: If you're using AngularJS version 1.6 or later, it's highly recommended to migrate to the more recent versions of Angular (Angular 2+ or Angular.js) to leverage the latest features and improvements. It will ensure better long-term support for your application. Just a heads up! 😉
That's it, folks! You've successfully resolved the "TypeError: $http.get(...).success is not a function" error. Give yourself a pat on the back! 👏
Feel free to let me know if you have any other questions or issues. I'm here to save your day with tech solutions! 😄🌟
Before you go, don't forget to share this blog post with your fellow developers who might be facing the same error. Sharing is caring! 🚀✨