How to use JavaScript regex over multiple lines?
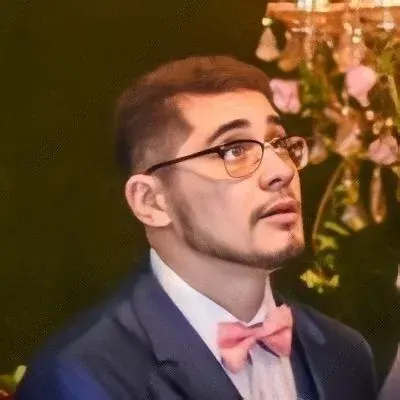
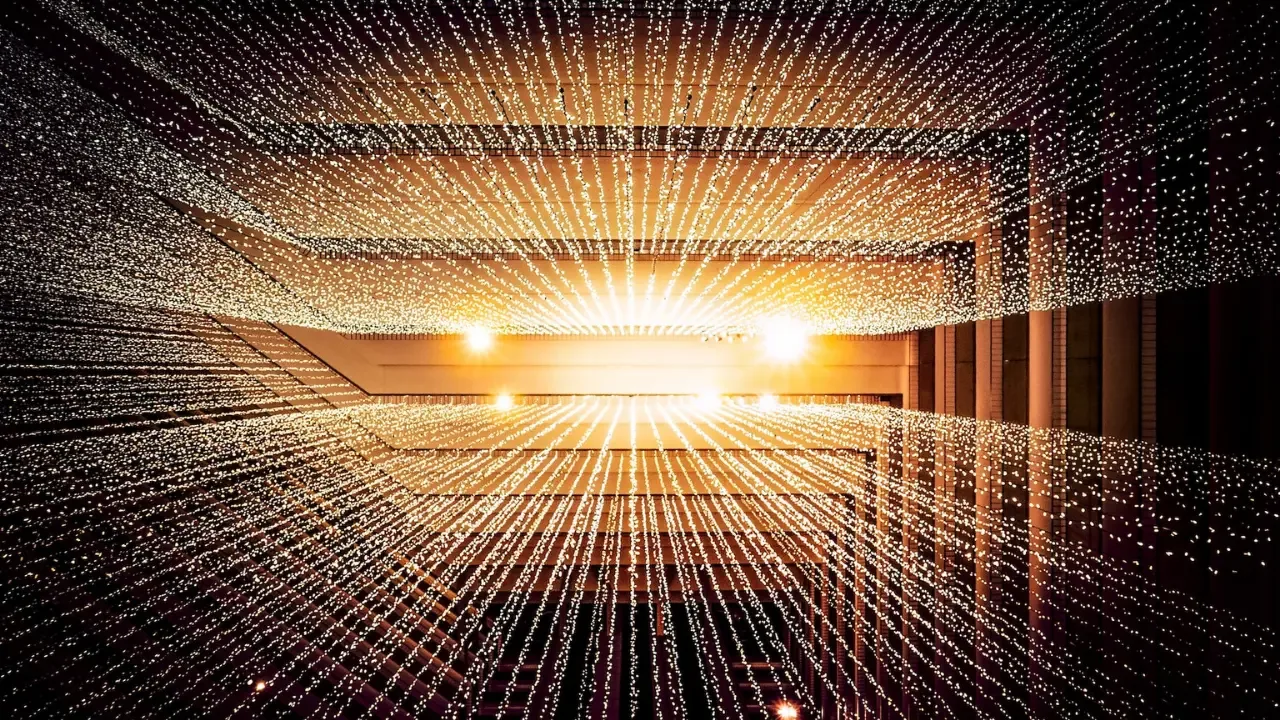
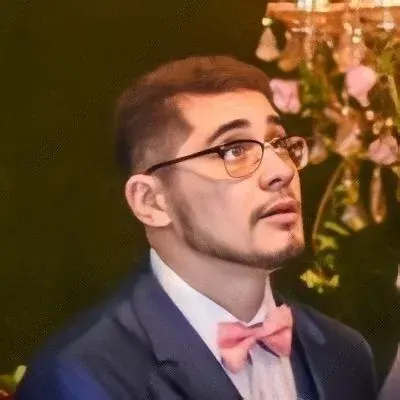
How to Use JavaScript Regex Over Multiple Lines? 💻🔍
Are you struggling to use JavaScript regex over multiple lines? 🤔 Don't worry, you're not alone! Many developers face this issue when trying to match patterns that span across newline characters. 📝
Let's dive into the problem, discover some common solutions, and find the best one for you! 🚀
The Problem: Matching Patterns Over Multiple Lines 🧩
Consider the following code snippet:
var ss = "<pre>aaaa\nbbb\nccc</pre>ddd";
var arr = ss.match(/<pre.*?<\/pre>/gm);
alert(arr); // null
In this example, we want to match the <pre>
block, even though it spans over newline characters. 😟 However, using the m
flag alone doesn't solve our problem.
Discovering Solutions: Unveiling the Best Strategy 🌟
Before we discuss the solution, let's explore a closely related article we found: this amazing post. 📚 It's always great to gather knowledge from different sources!
Now, here's the solution:
var ss = "<pre>aaaa\nbbb\nccc</pre>ddd";
var arr = ss.match(/<pre[\s\S]*?<\/pre>/gm);
alert(arr); // <pre>...<\/pre> 🙂
By using the [\s\S]*?
construct, we match any character (including newline characters) between the <pre>
and </pre>
tags. This ensures that our regex works over multiple lines! 🎉
Seeking Simplicity: A Less Cryptic Approach? 🙋♀️🤷♂️
You might be wondering if there's a less cryptic way to achieve this. We understand your concern!
Although the [\s\S]*?
solution is effective, there's an alternative we found in a duplicate question on Stack Overflow. It proposes using [^]
as a "multiline dot." Interesting, right? 🤔
var ss = "<pre>aaaa\nbbb\nccc</pre>ddd";
var arr = ss.match(/<pre[^]*?<\/pre>/gm);
alert(arr); // <pre>...<\/pre> 🎉
Note that this alternative might not be as well-known or widely used as the [\s\S]*?
approach. Choose the one that works best for you! 🤩
Call-To-Action: Share Your Thoughts and Insights! 💬✨
Now that you have an understanding of how to use JavaScript regex over multiple lines, it's time to share your thoughts and insights! 💭💡
Have you encountered any other challenges when working with regex in JavaScript? Do you prefer the [\s\S]*?
or [^]
approach? Let us know in the comments below! Let's learn and grow together as a community. 🌟👥
📢 Stay tuned for more exciting tech content and useful guides! And don't forget to follow us on Twitter for quick tips and updates. Happy coding! 💻😄