How to use FormData for AJAX file upload?
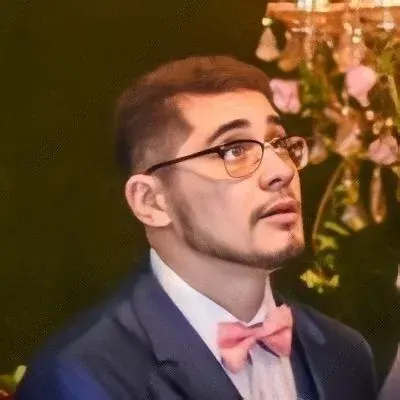
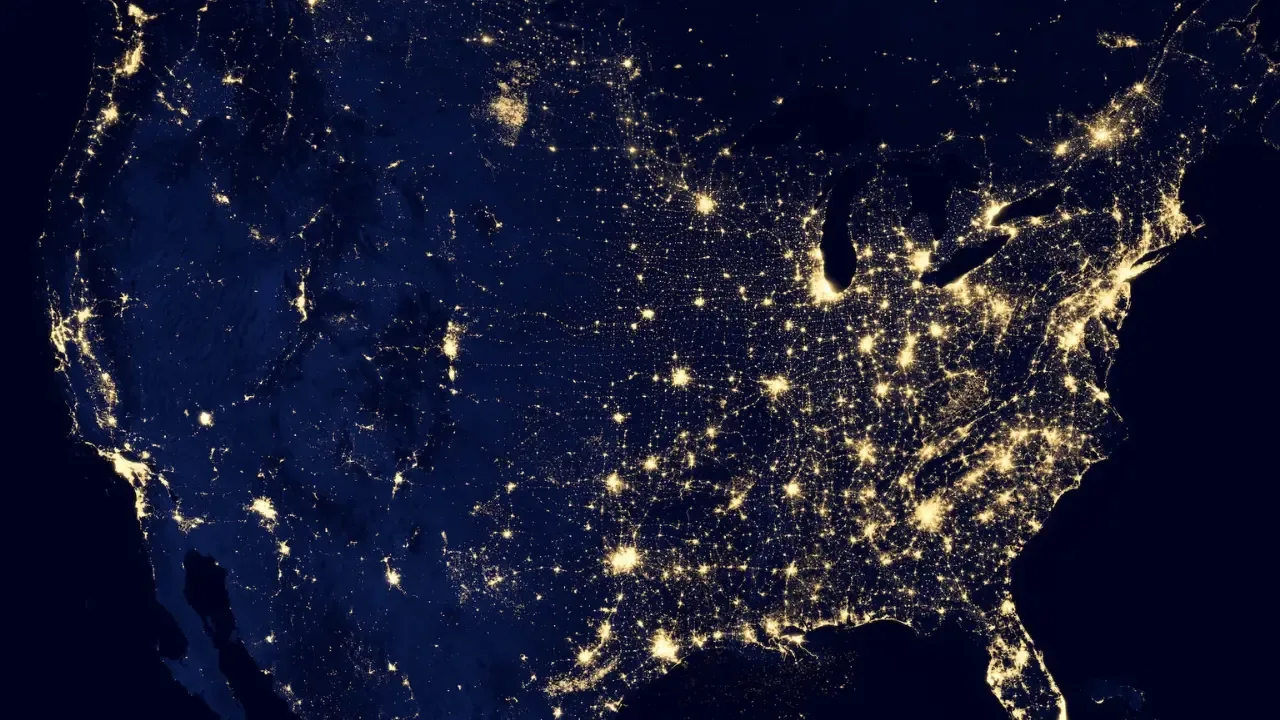
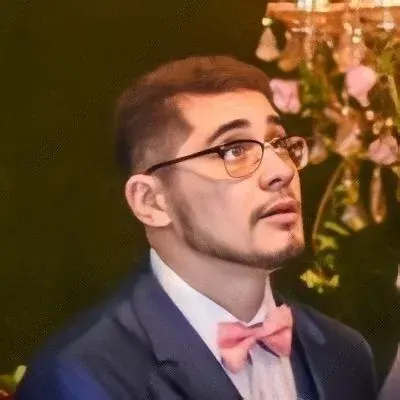
How to use FormData for AJAX file upload? πΎπ€
Have you ever needed to upload a file using AJAX, but didn't know how to handle it? This can be a common issue, especially when it comes to dynamically generated forms. But fear not! In this guide, we'll show you how to use FormData to successfully upload files using AJAX. π
The Problem
Let's start by taking a look at your code. You have a dynamically generated form and you want to upload a file using AJAX. However, you're not sure how to handle the file input. Don't worry, we've got you covered! π
The Solution
To handle file uploads using AJAX, we can make use of the FormData object. FormData is a powerful feature that allows you to effortlessly send and retrieve data from a form, including files. π
In your JavaScript code, you'll need to make a few modifications to successfully upload the file. Let's break it down step by step:
Capture the form submission event using the
.submit()
function in jQuery:
$('.wpc_contact').submit(function(event){
// Your code goes here
});
Get the form name and serialize the form data:
var formname = $('.wpc_contact').attr('name');
var form = $('.wpc_contact').serialize();
Create a new FormData object and pass in the form element:
var FormData = new FormData($('.wpc_contact')[0]);
Make an AJAX request with the FormData object:
$.ajax({
url: 'your_upload_url',
data: FormData,
type: 'POST',
processData: false,
contentType: false,
success: function(data){
// Handle the success response
}
});
That's it! By following these steps, you'll be able to upload files using AJAX with ease. π
The Call to Action
Now that you know how to use FormData for AJAX file uploads, it's time to put your knowledge into action! Try implementing this solution in your own project and see the magic happen. If you have any questions or need further assistance, feel free to leave a comment below. We're here to help! πͺ
So go ahead, take the leap, and start uploading those files like a pro! ππ