How to use componentWillMount() in React Hooks?
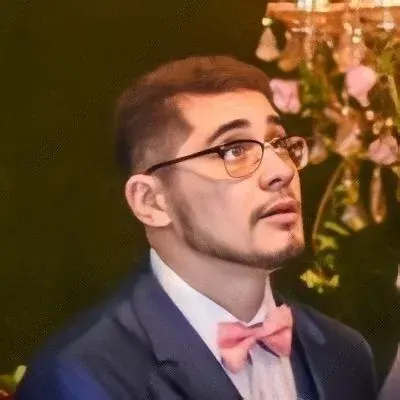
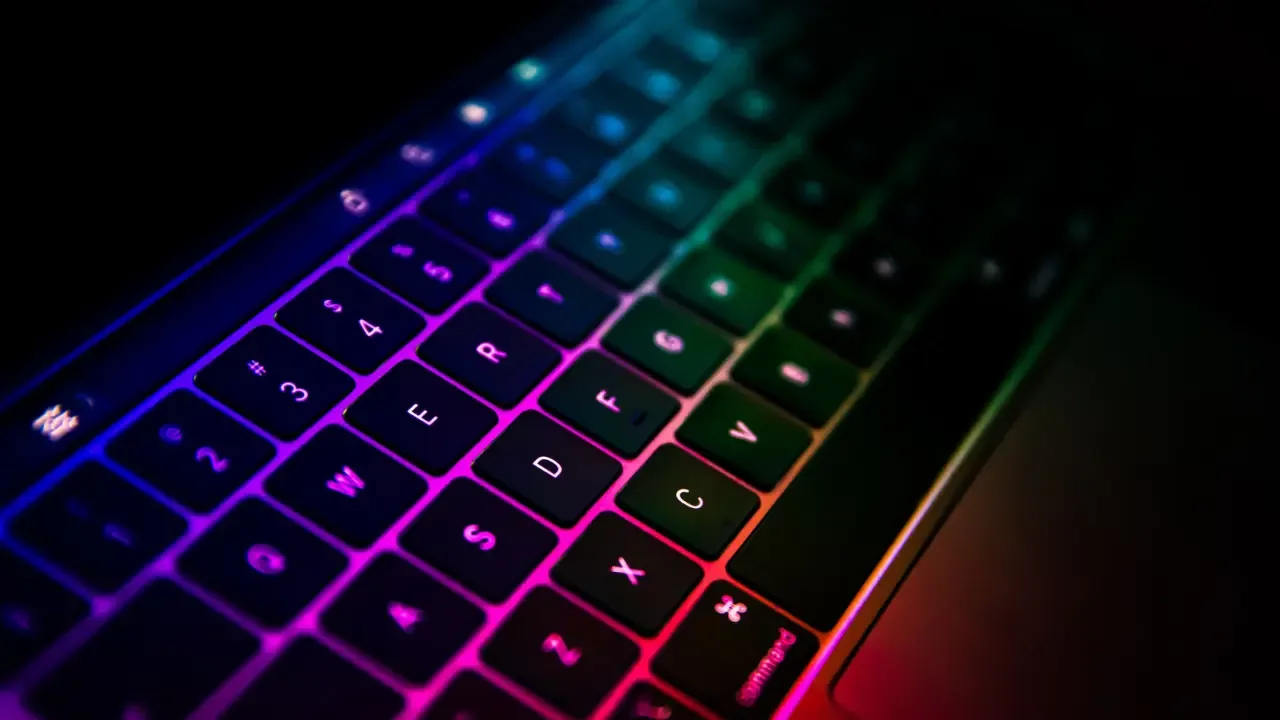
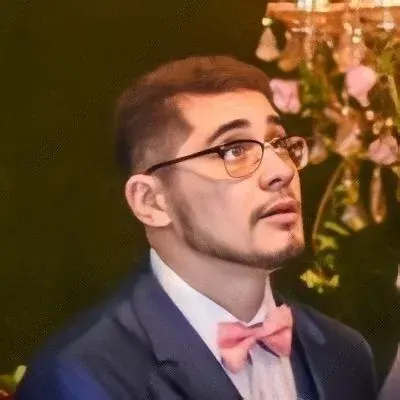
How to Use componentWillMount()
in React Hooks?
If you're familiar with React class lifecycle methods, you may have come across the componentWillMount()
method. But when it comes to React Hooks, you may wonder how to achieve the same functionality. In this blog post, we will discuss how to use componentWillMount()
in React Hooks and provide easy solutions to common issues you may encounter. So, let's dive in! 💪
Before we begin, it's important to note that the componentWillMount()
method is not available in React Hooks. It was part of the traditional class component lifecycle and is not supported in functional components. However, we can achieve similar functionality using the useEffect()
Hook.
The equivalent of componentWillMount()
in React Hooks
As mentioned in the official React documentation, we can think of useEffect()
as a combination of componentDidMount()
, componentDidUpdate()
, and componentWillUnmount()
lifecycle methods. So, to replicate the behavior of componentWillMount()
, we need to set up an effect that runs once, similar to how componentWillMount()
is triggered before the initial render.
To achieve this, we need to pass an empty dependency array as the second argument to the useEffect()
Hook. This ensures that the effect runs only once, mimicking the behavior of componentWillMount()
.
Here's an example of how to use componentWillMount()
in React Hooks:
import React, { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
// code to be executed before the initial render
// similar to the behavior of componentWillMount()
}, []);
// rest of the component code
}
By passing an empty dependency array, the effect will only run once when the component is mounted.
Common Issues and Solutions
Issue: Multiple renders
If you notice that your effect is running multiple times despite passing an empty dependency array, it might be due to some state or prop changes triggering a re-render. In such cases, you need to analyze your component and identify any dependencies that should be included in the dependency array.
Solution: Update the dependency array to include the necessary dependencies that affect the behavior of the effect. This ensures that the effect runs whenever those dependencies change, avoiding unnecessary re-renders.
Issue: Cleanup
In componentWillUnmount()
, you might have used it to clean up any resources or subscriptions to prevent memory leaks. With Hooks, we can achieve the same effect using the return
statement within the effect.
Solution: Return a cleanup function inside the effect, just like you would do in componentWillUnmount()
. This function will be executed when the component is unmounted or before a re-render if the effect's dependencies change.
import React, { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
// code to be executed before the initial render
return () => {
// cleanup code
// similar to the behavior of componentWillUnmount()
};
}, []);
// rest of the component code
}
Let's Hooks Things Up!
Though componentWillMount()
may not exist in React Hooks, we can achieve its functionality using the useEffect()
Hook with an empty dependency array. By addressing common issues and providing alternative solutions, we hope this guide has helped you understand how to effectively use componentWillMount()
in React Hooks.
Now it's your turn! 🚀 How do you handle component initialization logic in your React Hooks? Let us know in the comments below. Happy hooking! 💙