How to update React Context from inside a child component?
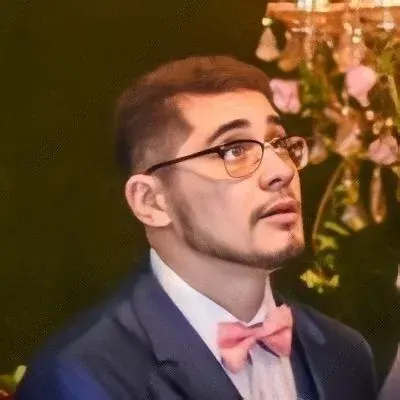
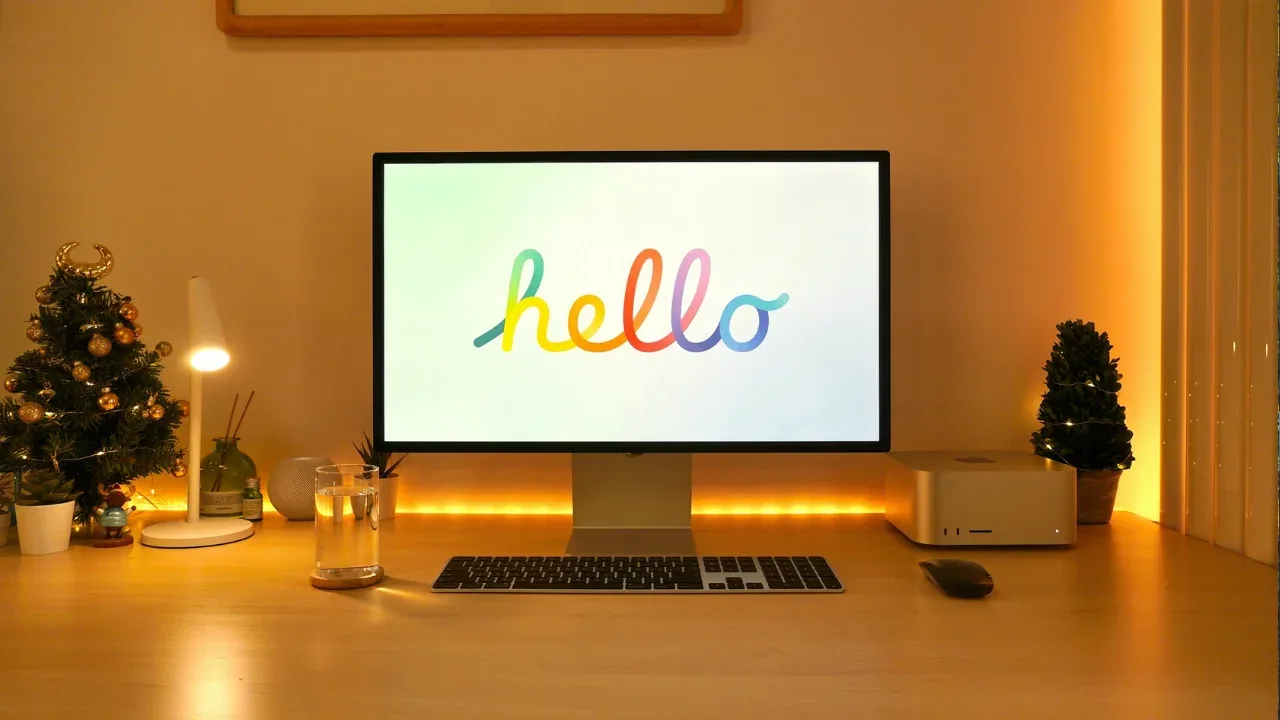
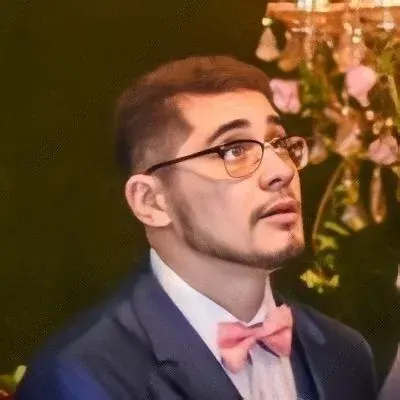
How to update React Context from inside a child component? 💻🔄
Are you having trouble updating the React Context from inside a child component? Don't worry, we've got you covered! 🤝 In this guide, we'll walk you through a simple and effective solution to this common problem. Let's dive in! 💡
Understanding the problem 🤔
So, you have a LanguageProvider
component that sets the initial language in the context, and a MyPage
component which renders a LanguageSwitcher
component. The goal is to update the language in the context from within the LanguageSwitcher
. However, you're unsure how to achieve this. Let's explore the solution together! 🚀
The Solution: Updating the Context 🛠️
To update the context from inside a child component, you'll need to follow these steps:
Import the
Context
andConsumer
from yourLanguageProvider
component into theLanguageSwitcher
component.
import { Context } from '../LanguageProvider'; // Update the path to your LanguageProvider component
Inside the
updateLanguage
method of theLanguageSwitcher
, access thelangConfig
property from the context and update it accordingly.
updateLanguage() {
const { langConfig } = this.context;
langConfig = 'jp'; // Update the language to 'jp' or any other desired value
}
Lastly, wrap your desired content inside the
Consumer
component, passing thecontext
as a prop.
render() {
return (
<Context.Consumer>
{context => (
<button onClick={this.updateLanguage}>Change Language</button>
)}
</Context.Consumer>
);
}
That's it! You've successfully updated the context from within the LanguageSwitcher
component. 🎉
Bringing it all together 🌟
Let's recap the steps we took to update the context from inside a child component:
Imported the
Context
andConsumer
from theLanguageProvider
component.Accessed the
langConfig
property from the context and updated it inside theupdateLanguage
method.Wrapped the desired content in the
Consumer
component, passing the context as a prop.
Now, you can confidently update the language in your React Context from within the LanguageSwitcher
component. 💪
Share your success and keep learning! 📣📚
Don't forget to share your success story in the comments below! We love hearing about how our guides help developers overcome obstacles. 👏
Remember, learning is an ongoing journey, and we're here to support you every step of the way! If you have any further questions or need assistance with any other React-related topics, feel free to reach out. Happy coding! 😄✨