How to update nested state properties in React
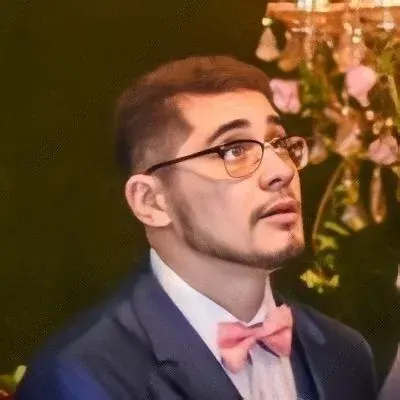
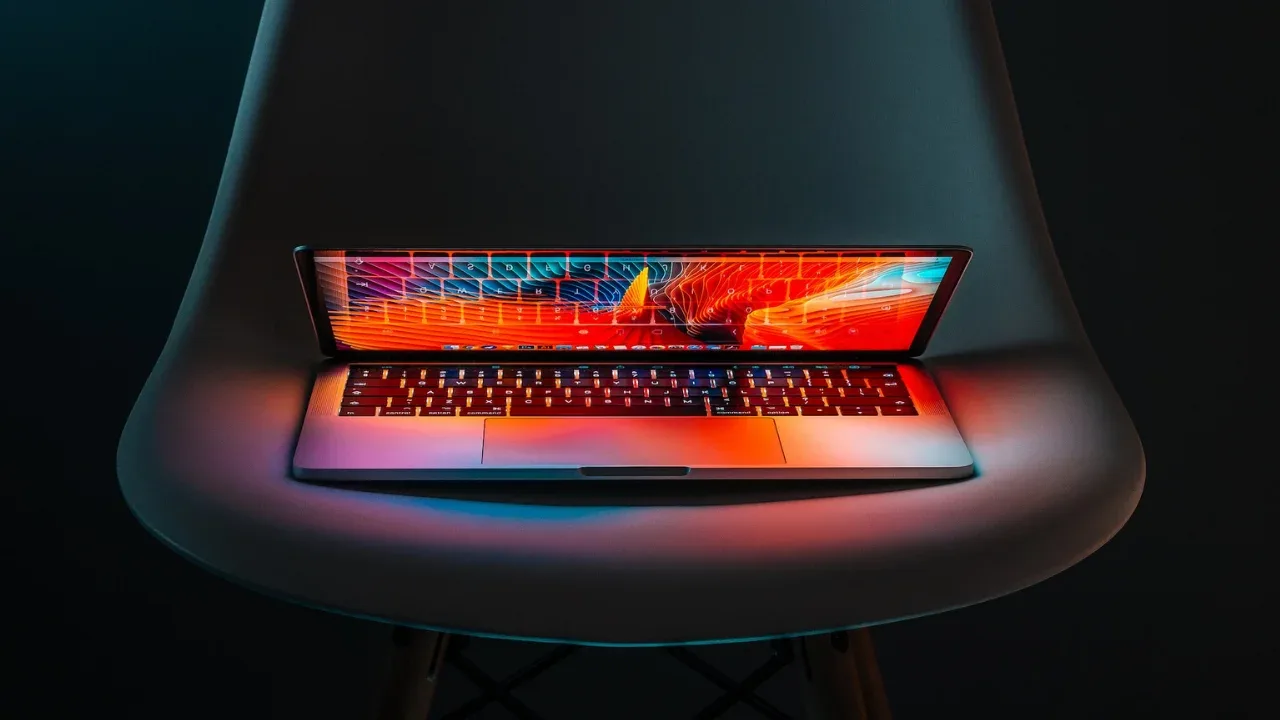
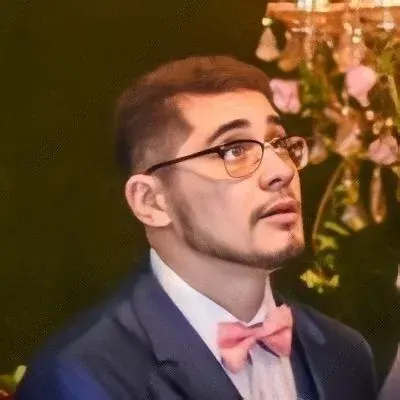
Updating Nested State Properties in React: A Complete Guide 📝🚀
Have you ever found yourself struggling to update nested state properties in React? 🤔 Fear not! In this blog post, we will tackle this common issue head-on and provide you with easy solutions to ensure your React applications run smoothly. So, let's dive right in! 💪😎
Understanding the Problem 🤔
The problem arises when we try to directly update a nested state property using the setState
method. As mentioned in the context, attempting to update the desired nested property like this this.setState({ someProperty.flag: false });
will not work. 😞
Why the Direct Approach Fails ❌
In JavaScript, you cannot directly assign a value to a nested property using dot notation within an object literal. This limitation applies to React's setState
method as well. Instead, we need to follow a different approach to update nested state properties. 🔄
The Correct Approach ✅
To update nested state properties in React, we must ensure that we don't mutate the existing state directly. Instead, we create a copy of the nested property, update the necessary values, and then set the new state using setState
. Let's walk through a step-by-step example to make it crystal clear. 🔍👀
// Step 1: Create a copy of the parent object
const updatedProperty = { ...this.state.someProperty };
// Step 2: Update the nested property
updatedProperty.flag = false;
// Step 3: Set the new state using setState
this.setState({ someProperty: updatedProperty });
In the example above, we followed a safe approach to update the flag
property within the someProperty
object. By creating a copy of the parent object, we avoid mutating the original state and adhere to React's immutability principles. 🔄✨
A Simplified Solution using the Updater Function 🎉
React provides us with an alternative solution to update nested state properties using the updater function inside the setState
method. This approach is particularly handy when dealing with asynchronous state updates, ensuring we always have the latest state. 😄🔄
// Using the updater function
this.setState((prevState) => {
// Step 1: Create a copy of the parent object from the previous state
const updatedProperty = { ...prevState.someProperty };
// Step 2: Update the nested property
updatedProperty.flag = false;
// Step 3: Return the updated state
return { someProperty: updatedProperty };
});
By accessing the previous state using prevState
, we ensure that we always have the most up-to-date state when updating nested properties using the updater function. This approach safeguards against any potential race conditions or unexpected behavior. 🏎️🔒
Conclusion and Call-to-Action 🎬💡
Updating nested state properties in React doesn't have to be a headache anymore! By following the correct approaches outlined in this guide, you can confidently handle nested state updates in your React applications. Remember, always create a copy of the parent object and update the nested property accordingly to maintain React's immutability principles.
If you found this guide helpful, why not share it with your fellow developers or React enthusiasts? 👥🔗 Spread the knowledge and empower others to tackle nested state updates like pros! If you have any further questions or tips, feel free to leave a comment below and join the discussion. Let's level up our React game together! 🚀💪
Happy coding! 👩💻👨💻