How to test if a string is JSON or not?
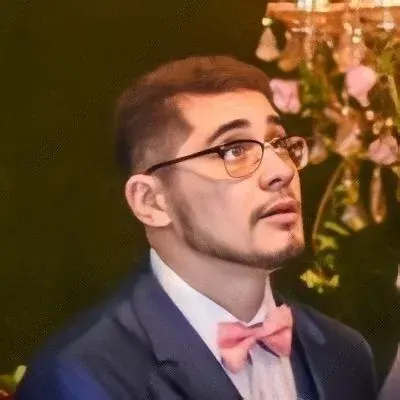
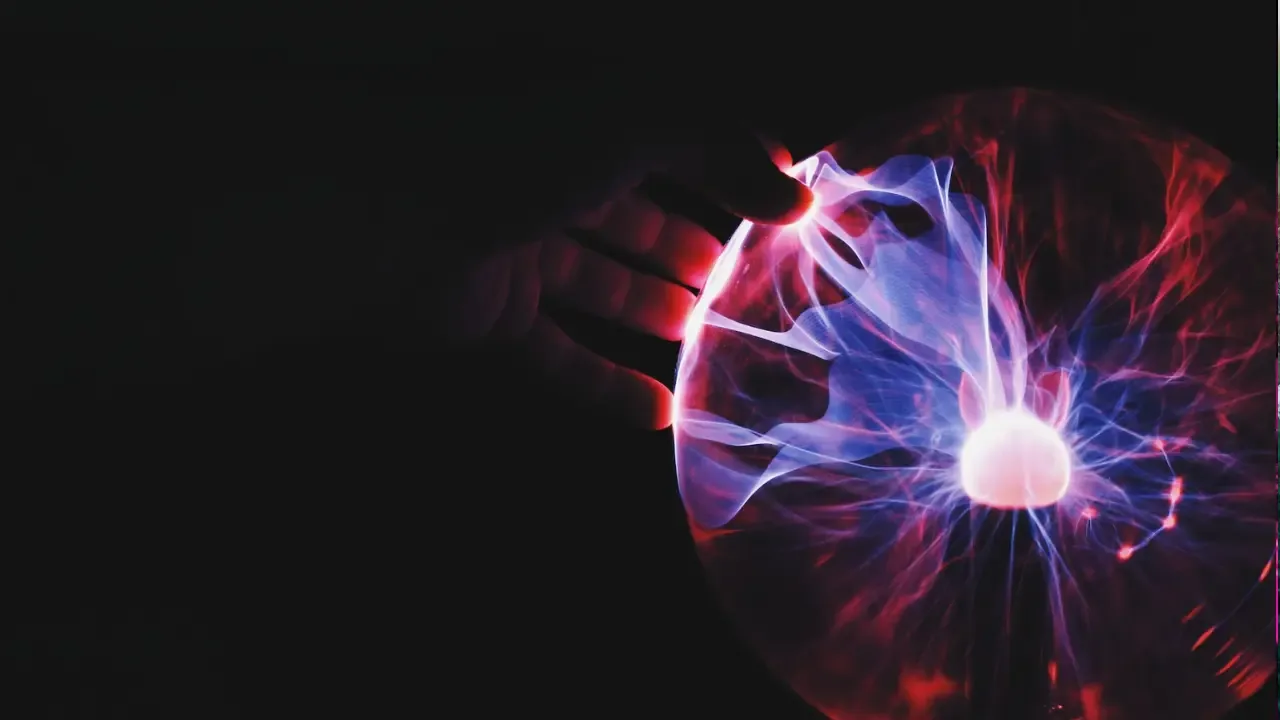
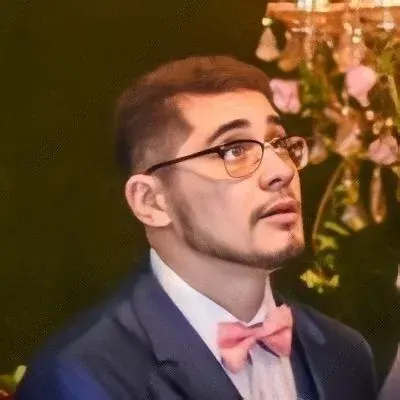
How to Test if a String is JSON or Not?
So you have a problem. You're making an AJAX call to your server, and it could potentially return two different types of data: a JSON string with useful information, or an error message string generated by the mysql_error()
function in PHP. Now you're scratching your head, wondering how to test whether the returned data is a JSON string or an error message. Fear not, my friend! I've got you covered with a handy solution.
The Challenge
Let's break down the problem a bit. You want to determine if a given string is in valid JSON format. But how do you go about that?
The Solution
To tackle this challenge, we'll create our own custom function called isJSON()
that will do the job for us. Here's an example implementation:
function isJSON(data) {
try {
JSON.parse(data);
} catch (e) {
return false;
}
return true;
}
Let's dissect this function a bit. We start by using JSON.parse()
to try and parse the given data
string as JSON. If parsing succeeds, it means that the string is in valid JSON format, and we return true
. If an exception is thrown during the parsing process, it means that the string is not valid JSON, and we catch the error, returning false
.
Now that we have our trusty isJSON()
function, we can use it to solve our original problem:
if (isJSON(data)){
// do some data stuff
} else {
// report the error
alert(data);
}
In the code above, we first check if the data
string is valid JSON using isJSON()
. If it is, we can safely assume that it contains useful data and proceed accordingly. If it's not valid JSON, we treat it as an error message and alert it to the user.
Example Scenario
Let's say you make an AJAX call, and the server responds with the following data:
var data = '{"username": "john_doe", "age": 25}';
If you use the isJSON()
function on this data
string, it will return true
since it's a valid JSON string. You can then process the data and access the username
and age
properties.
Now, let's consider another scenario where the server responds with an error message:
var data = 'Database connection failed.';
This time, the isJSON()
function will return false
since the string is not valid JSON. In the else
block of your code, you can handle the error by alerting the error message to the user.
Call-to-Action
So now you know how to test if a string is JSON or not! It's time to put your newfound knowledge to the test and start implementing it in your own projects. Remember, the isJSON()
function is your friend when dealing with potentially JSON data, giving you more control and stability in your applications.
If you found this blog post helpful or have any questions, let me know in the comments below. I'd love to hear your thoughts and experiences with testing JSON strings. Happy coding! 🚀