How to store objects in HTML5 localStorage/sessionStorage
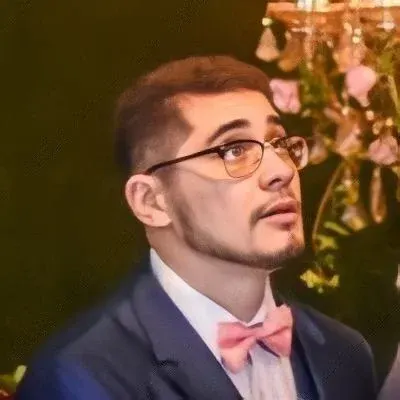
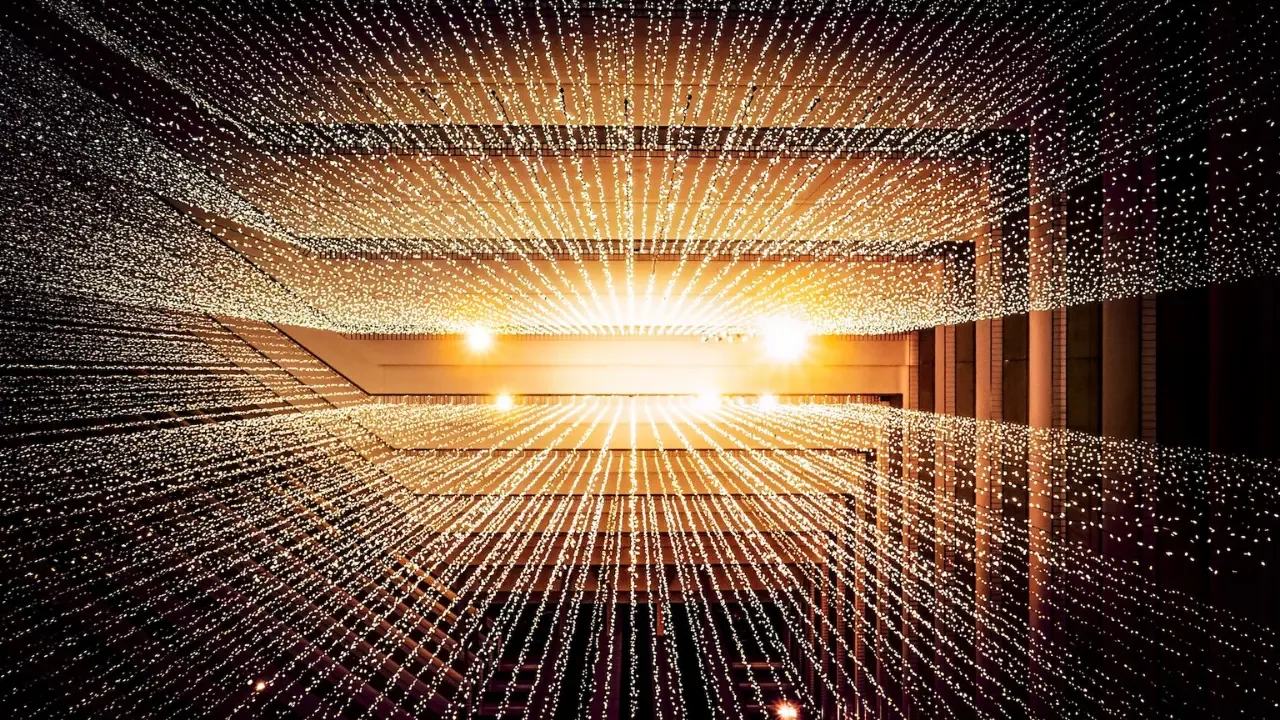
How to Store Objects in HTML5 localStorage/sessionStorage
šš» Hey there! Are you trying to store a JavaScript object in HTML5 localStorage
but it's being converted to a string instead? Don't worry, you're not alone. Many developers have faced this issue, but luckily there is an easy workaround. In this guide, I'll explain why this happens and provide you with a simple solution.
Understanding the Problem
When you try to store an object in localStorage
, the setItem
method converts the object to a string before saving it. That's why when you retrieve the object using getItem
, it returns a string representation of the object instead of the actual object.
Let's take a look at the code you shared:
var testObject = { 'one': 1, 'two': 2, 'three': 3 };
console.log('typeof testObject: ' + typeof testObject);
console.log('testObject properties:');
for (var prop in testObject) {
console.log(' ' + prop + ': ' + testObject[prop]);
}
// Put the object into storage
localStorage.setItem('testObject', testObject);
// Retrieve the object from storage
var retrievedObject = localStorage.getItem('testObject');
console.log('typeof retrievedObject: ' + typeof retrievedObject);
console.log('Value of retrievedObject: ' + retrievedObject);
The console output shows:
typeof testObject: object
testObject properties:
one: 1
two: 2
three: 3
typeof retrievedObject: string
Value of retrievedObject: [object Object]
As you can see, the testObject
is of type object
, but when retrieved from localStorage
, it becomes a string representation of [object Object]
.
The Workaround
To store objects in localStorage
or sessionStorage
, we need to serialize the object into a string and then deserialize it back into an object when retrieving it.
One way to do this is by using JSON.stringify() to convert the object to a string before storing it, and JSON.parse() to convert the string back to an object when retrieving it.
Here's an updated version of your code with the workaround:
var testObject = { 'one': 1, 'two': 2, 'three': 3 };
// Serialize the object and store it
localStorage.setItem('testObject', JSON.stringify(testObject));
// Retrieve the serialized object from storage and deserialize it
var retrievedObject = JSON.parse(localStorage.getItem('testObject'));
console.log('typeof retrievedObject: ' + typeof retrievedObject);
console.log('Value of retrievedObject: ', retrievedObject);
After making these changes, you should see the following console output:
typeof retrievedObject: object
Value of retrievedObject: { one: 1, two: 2, three: 3 }
Voila! Now you can store and retrieve objects from localStorage
without any issues.
Conclusion
Storing objects in HTML5 localStorage
or sessionStorage
may seem tricky at first, but with the workaround of serializing and deserializing the object using JSON, it becomes super easy. Remember to use JSON.stringify()
when storing the object, and JSON.parse()
when retrieving it.
š Now that you know how to solve this common issue, go ahead and experiment with storing and retrieving different objects in localStorage
. If you have any questions or other cool tips, feel free to share them in the comments below! Happy coding! š©āš»šØāš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
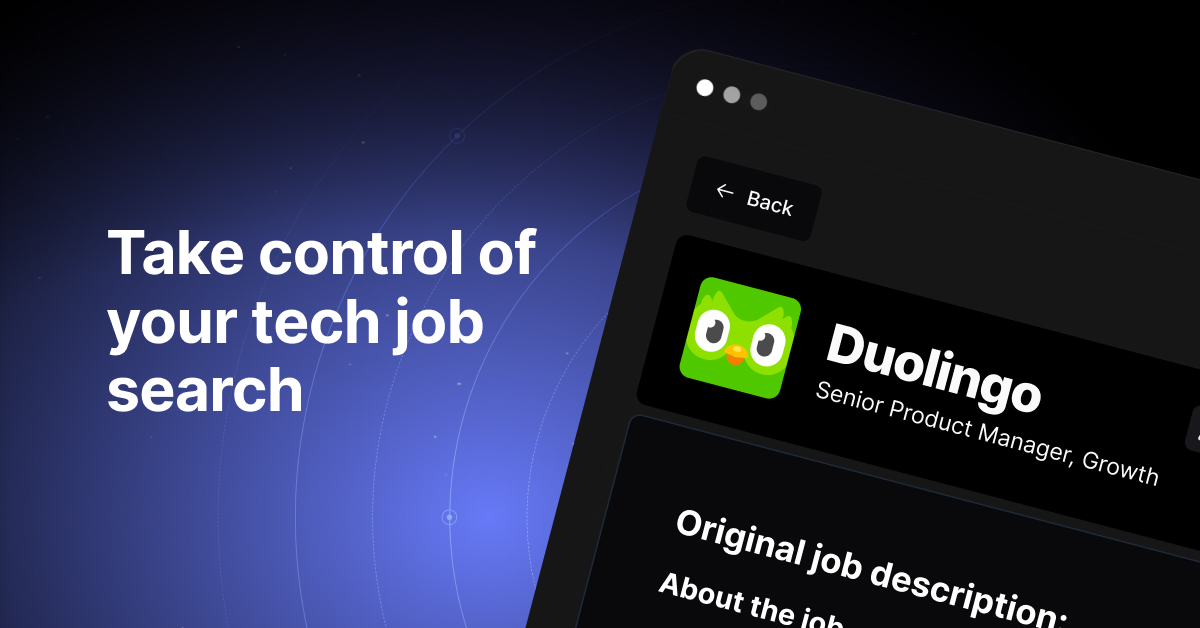