How to sort an array of integers correctly
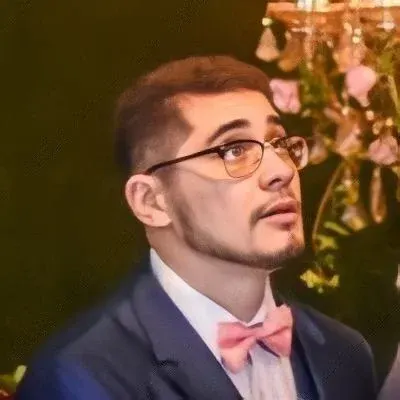
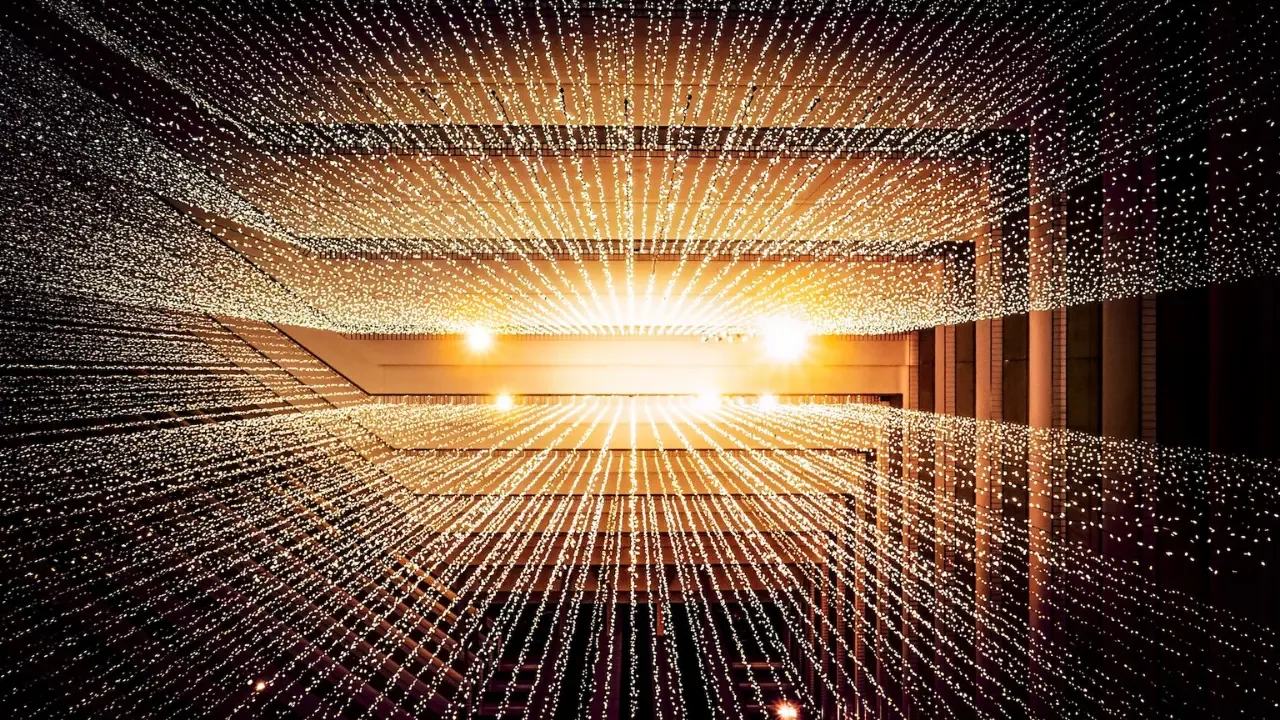
📝 How to Sort an Array of Integers Correctly
So you want to sort an array of integers, huh? It sounds like a straightforward task, but as you may have already experienced, it can be trickier than expected. Don't worry, though! I'm here to help you out. Let's dive into this conundrum and find an easy solution. 💪
🔍 The Problem: Sorting an Array of Integers Gone Wrong
The issue you encountered when sorting your array is quite common. By default, JavaScript's sort()
function sorts values as strings, not integers. This means that it considers the comparison based on the Unicode code points of the characters. 😮
For instance, when you sort the array [140000, 104, 99]
, you expect the output to be [99, 104, 140000]
. However, JavaScript sorts it as [104, 140000, 99]
. Bummer, right?
Let's now explore some easy solutions to correctly sort an array of integers. 🔄
💡 Solution: Custom Sorting Function
To sort an array of integers correctly, you need to provide a custom sorting function as an argument to the sort()
method. This custom function tells JavaScript how to compare the values as integers, rather than as strings.
Here's an example of how you can implement this solution:
var numArray = [140000, 104, 99];
numArray.sort(function(a, b) {
return a - b;
});
console.log(numArray); // Output: [99, 104, 140000]
In the above code, the custom sorting function calculates the difference between a
and b
. This instructs JavaScript to sort the array in ascending order by comparing the integer values directly. Amazing, right? 😄
📣 Time to Take Action: Engage and Share Your Thoughts
Now that you know how to correctly sort an array of integers, what do you think about it? Have you faced any other challenges while working with JavaScript arrays? I'd love to hear about your experiences and any questions you may have!
Leave a comment below and let's spark a conversation. Don't forget to share this blog post with your friends who might find it helpful. Together, we can spread the knowledge and make programming a breeze for everyone! ✨
Happy sorting! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
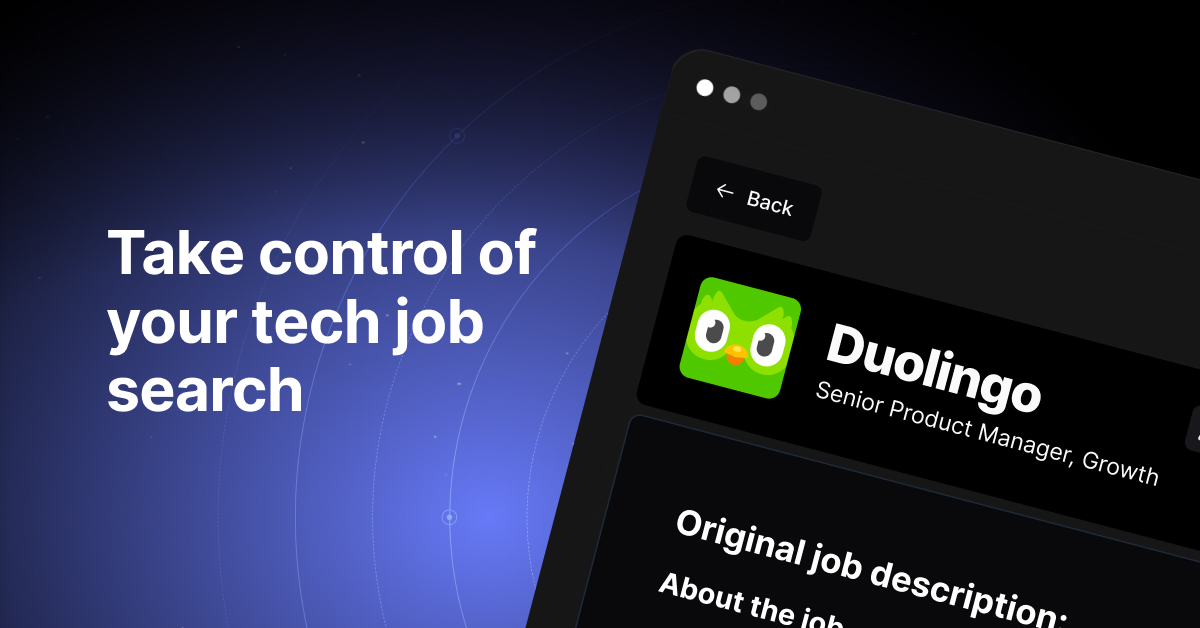