How to set focus on an input field after rendering?
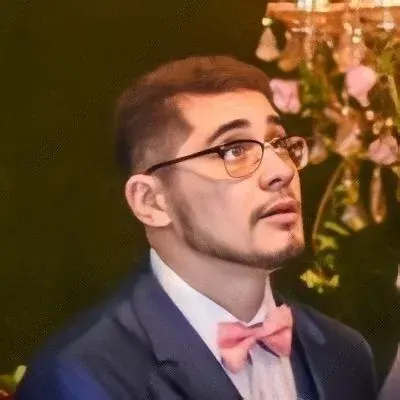
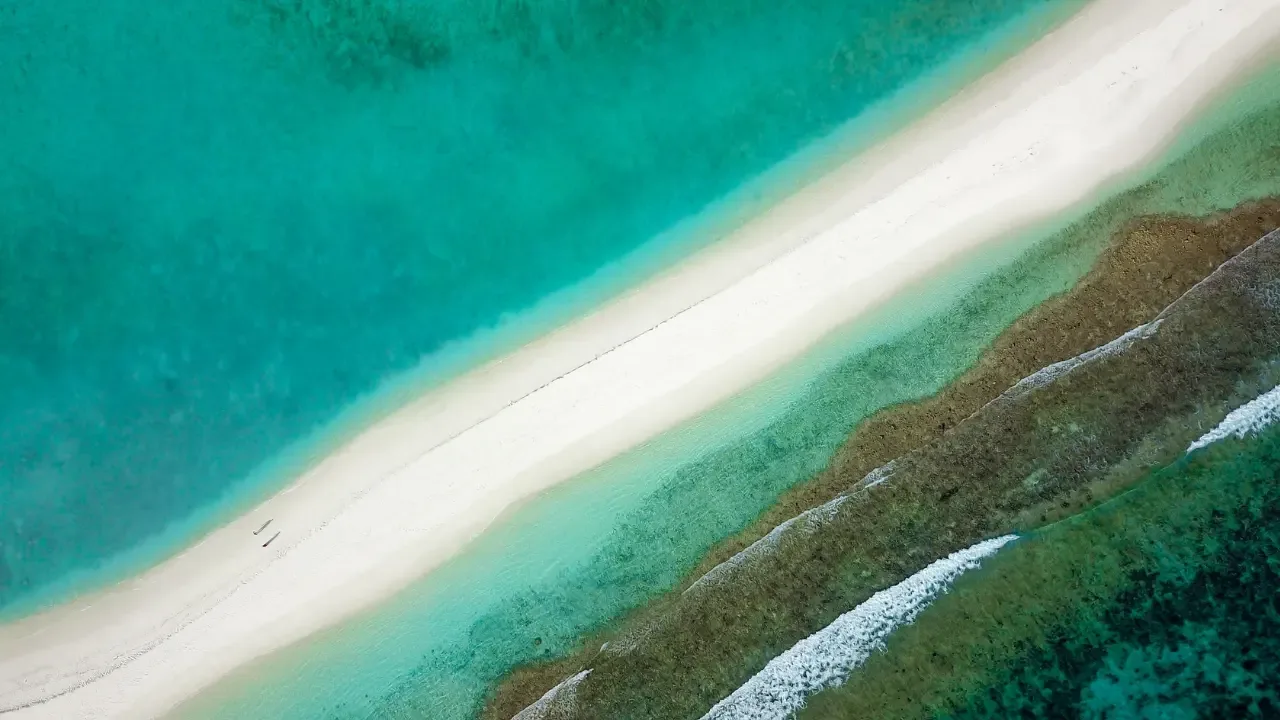
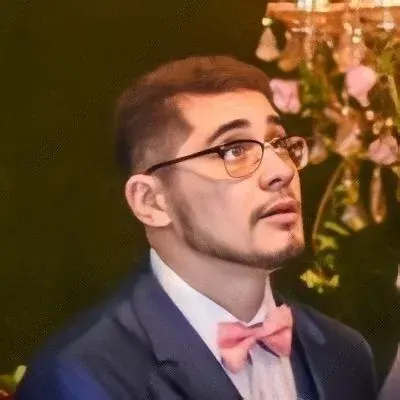
🔍 How to Set Focus on an Input Field After Rendering? 🎯
So, you want to set focus on a specific input field after your component is rendered in React. You've come to the right place! 🙌
The suggested approach is to use "refs" to achieve this. In your render function, you can set the ref attribute on the desired input field like this:
<input ref="nameInput" />
Now comes the important part - actually setting the focus. After your component is rendered, you'll need to call the focus()
method on the ref using the getInputDOMNode()
function.
componentDidMount() {
this.refs.nameInput.getInputDOMNode().focus();
}
But, where should you call this? It's a common confusion, my friend! You might have tried a few places, but couldn't get it to work. Let's find a suitable spot together. 🧐
💡 Solution:
To make this work, you should call the .focus()
method in the componentDidMount()
lifecycle method of your component. This is the best place to perform tasks after the component has been rendered.
Here's an example of how you can do it:
class YourComponent extends React.Component {
componentDidMount() {
this.refs.nameInput.getInputDOMNode().focus();
}
render() {
return (
<form>
<label>
Name:
<input ref="nameInput" />
</label>
<button>Submit</button>
</form>
);
}
}
Now, when your component is rendered, you'll see that the "Name" input field is automatically focused for a seamless user experience. 🎉
That's it! You've successfully tackled the challenge of setting focus on an input field after rendering in React. Pretty cool, right? 😎
If you still have any questions, feel free to leave a comment below. Don't forget to share this post with your fellow React enthusiasts, because sharing is caring! 👥💙
Happy coding! ✨