How to set an iframe src attribute from a variable in AngularJS
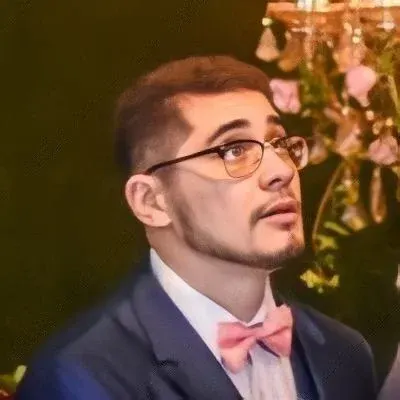
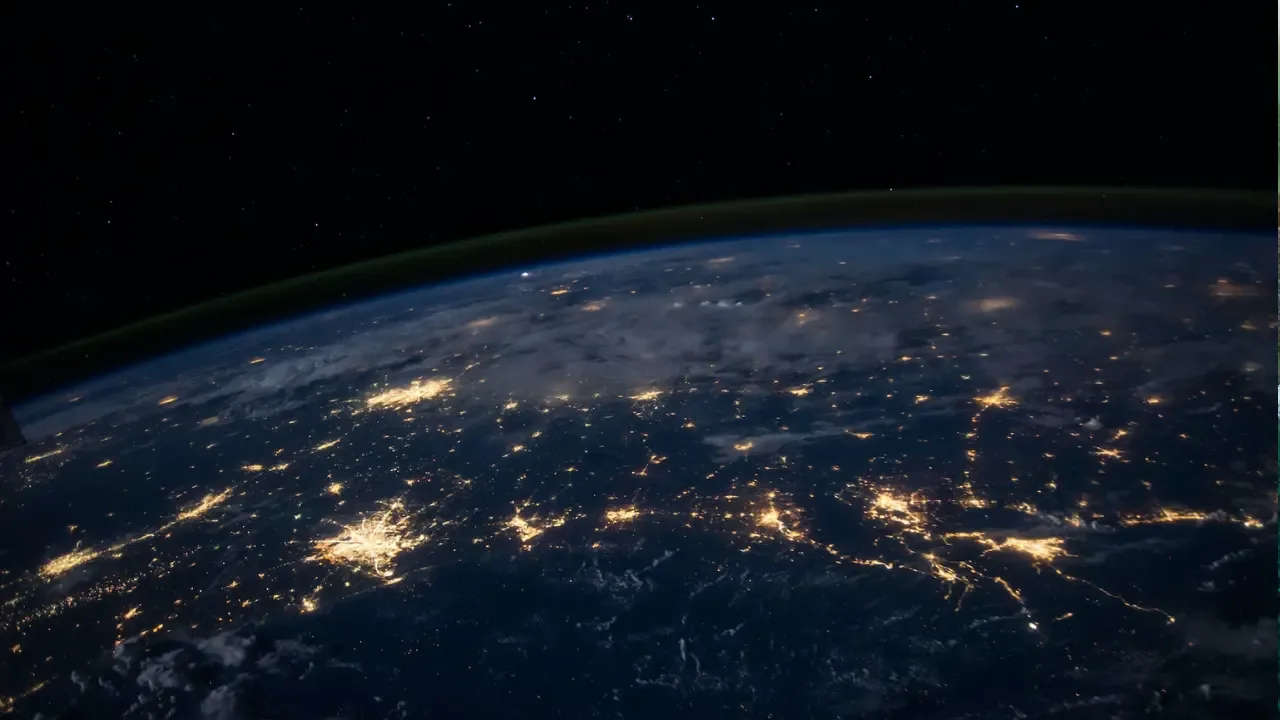
How to set an iframe src attribute from a variable in AngularJS 😎
So, you're trying to set the src
attribute of an iframe from a variable in AngularJS, but it's not working as expected. Don't worry, I've got you covered! In this blog post, I'll walk you through the common issues and provide easy solutions to get it working seamlessly. Let's dive in! 💪
The problem 😕
Based on the given context, you have an iframe element where you want to set the src
attribute dynamically from a variable, currentProject.url
. However, when you try to do it, the iframe remains blank.
Solution 1: Trust the source URL with $sce.trustAsResourceUrl() 🧐
To solve this issue, you can use AngularJS's $sce service to trust the source URL, which is necessary for iframe src attributes.
First, inject the
$sce
dependency into theAppCtrl
controller if you haven't done it already.Inside the controller, modify the
setProject()
function as follows:
$scope.setProject = function (id) {
$scope.currentProject = $scope.projects[id];
$scope.currentProjectUrl = $sce.trustAsResourceUrl($scope.currentProject.url);
console.log($scope.currentProject);
console.log($scope.currentProjectUrl);
}
Here, we use $sce.trustAsResourceUrl()
to trust the URL stored in currentProject.url
and assign it to the currentProjectUrl
variable.
In your HTML, update the
ng-src
attribute of the iframe element to use thecurrentProjectUrl
variable:
<iframe ng-src="{{ currentProjectUrl }}">
Something wrong...
</iframe>
Explanation 🤓
When setting an iframe's src
attribute from a variable in AngularJS, you need to trust the source URL using $sce.trustAsResourceUrl()
. This is a security measure to prevent Cross-Site Scripting (XSS) attacks. By trusting the URL, AngularJS allows the iframe to load its content.
Update 1: TrustedValueHolderType 😮
It seems like you've encountered the TrustedValueHolderType
when using $sce.trustAsResourceUrl()
inside the ng-src
attribute. This is because $sce.trustAsResourceUrl()
returns a wrapper object, not the actual URL. To get the URL, you can use .toString()
on the trusted URL holder object.
<iframe ng-src="{{ currentProjectUrl.toString() }}">
Something wrong...
</iframe>
Update 2: Security warning when passing trusted URL 😬
If you're getting a security warning even after trusting the URL, make sure that the trusted URL is properly passed into the src
attribute. Double-check the value of currentProjectUrl
and ensure that it's correctly assigned.
Conclusion 🎉
Setting the src
attribute of an iframe from a variable in AngularJS can be achieved by trusting the URL with $sce.trustAsResourceUrl()
. By following the steps mentioned above, you'll be able to dynamically load iframe content without any issues.
Don't hesitate to leave a comment if you have any questions or suggestions. Happy coding! 😊
Want to learn more? 📚
If you're interested in AngularJS and want to dive deeper into its functionalities, check out our AngularJS Beginner's Guide for an in-depth journey into the framework. 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
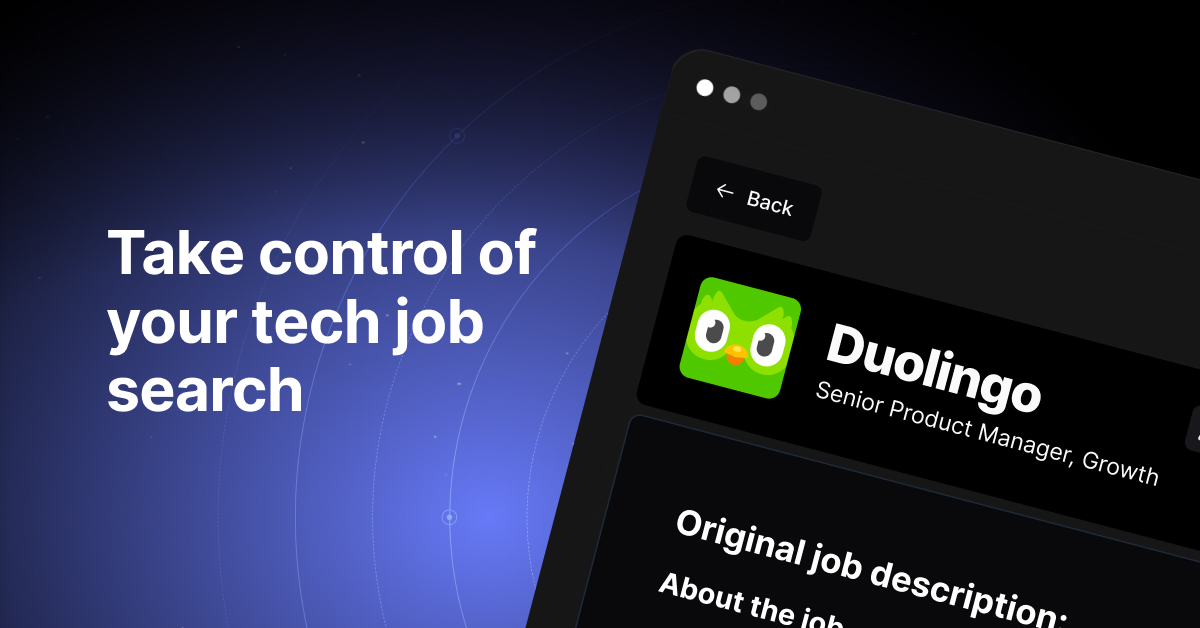