How to send FormData objects with Ajax-requests in jQuery?
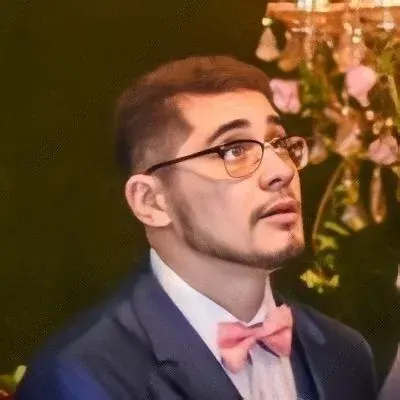
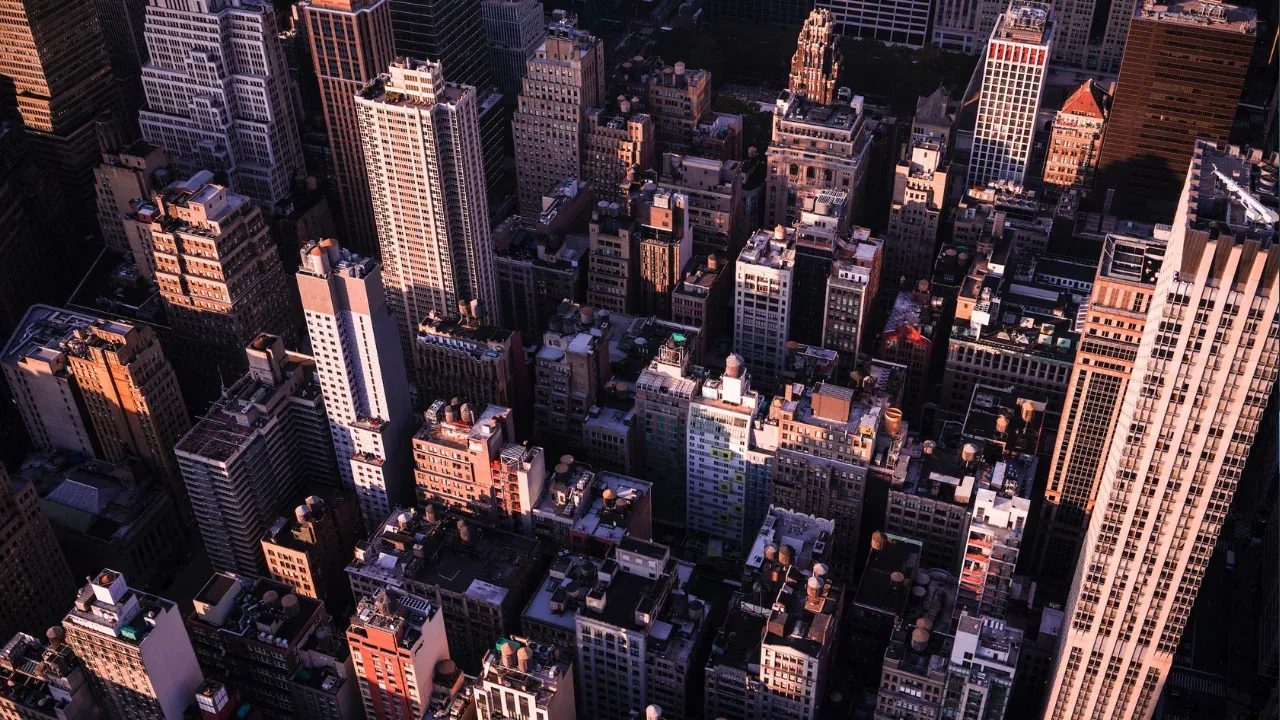
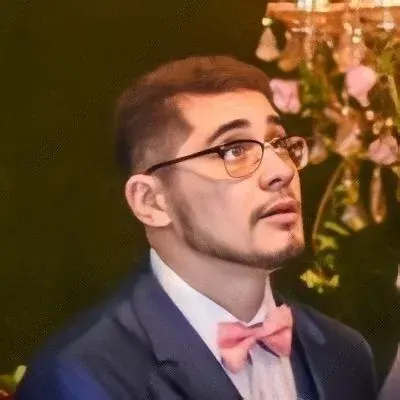
Sending FormData Objects with Ajax-requests in jQuery
๐ค Are you looking for a way to send FormData objects with Ajax-requests in jQuery? Look no further! In this blog post, we'll address this common issue and provide you with easy solutions. Let's dive right in! ๐ช
Understanding the Issue
The XMLHttpRequest Level 2 standard introduced the FormData interface, which allows you to append File objects to Ajax requests. This new feature is great because it eliminates the need for the old "hidden-iframe-trick" approach. However, when it comes to using jQuery, things can get a bit tricky. ๐
The Incorrect Approach
Before we explore the correct solution, let's discuss an approach that may seem intuitive but won't work. If you try the following code snippet, you'll encounter an "Illegal invocation" error. Let's take a look: ๐ซ
var fd = new FormData();
fd.append('file', input.files[0]);
$.post('http://example.com/script.php', fd, handler);
The error occurs because jQuery expects a simple key-value object to represent form field names and values. Unfortunately, the FormData instance we're passing in is incompatible with this expectation.
The Correct Solution
But fear not! There's a simple workaround to make this functionality work with jQuery. ๐ To send FormData objects with Ajax-requests using jQuery, follow these steps:
Create a FormData instance and append the desired data to it. ๐ค
var data = new FormData();
data.append('file', $('#file')[0].files[0]);
Use the
$.ajax()
method to make the Ajax request. Set theurl
to the desired destination and thedata
property to the FormData object. Additionally, make sure to setprocessData
tofalse
to prevent jQuery from converting the data. Finally, specify thetype
asPOST
. ๐
$.ajax({
url: 'http://example.com/script.php',
data: data,
processData: false,
type: 'POST',
success: function (response) {
// Handle the success response here
}
});
By following these steps, you'll be able to send FormData objects successfully using Ajax-requests in jQuery. ๐
Testing the Solution
To make sure you've implemented the solution correctly, you can test it with the provided demo code. The HTML and JavaScript snippets below demonstrate the desired behavior: ๐งช
<form>
<input type="file" id="file" name="file">
<input type="submit">
</form>
$('form').submit(function (e) {
var data = new FormData();
data.append('file', $('#file')[0].files[0]);
$.ajax({
url: 'http://hacheck.tel.fer.hr/xml.pl',
data: data,
processData: false,
type: 'POST',
success: function (response) {
alert(response);
}
});
e.preventDefault();
});
If everything works as expected, you should see a successful HTTP request with the "multipart/form-data" content-type. ๐
Engage with Us
We hope this blog post has helped you overcome the challenge of sending FormData objects with Ajax-requests in jQuery. If you have any further questions or insights, feel free to leave a comment or engage with us on our website or social media channels. We'd love to hear from you! Let's make web development easier together. ๐