How to send a message to a particular client with socket.io
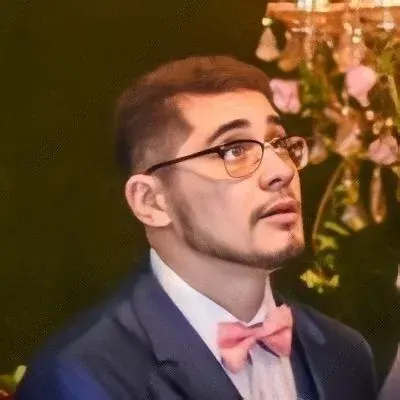
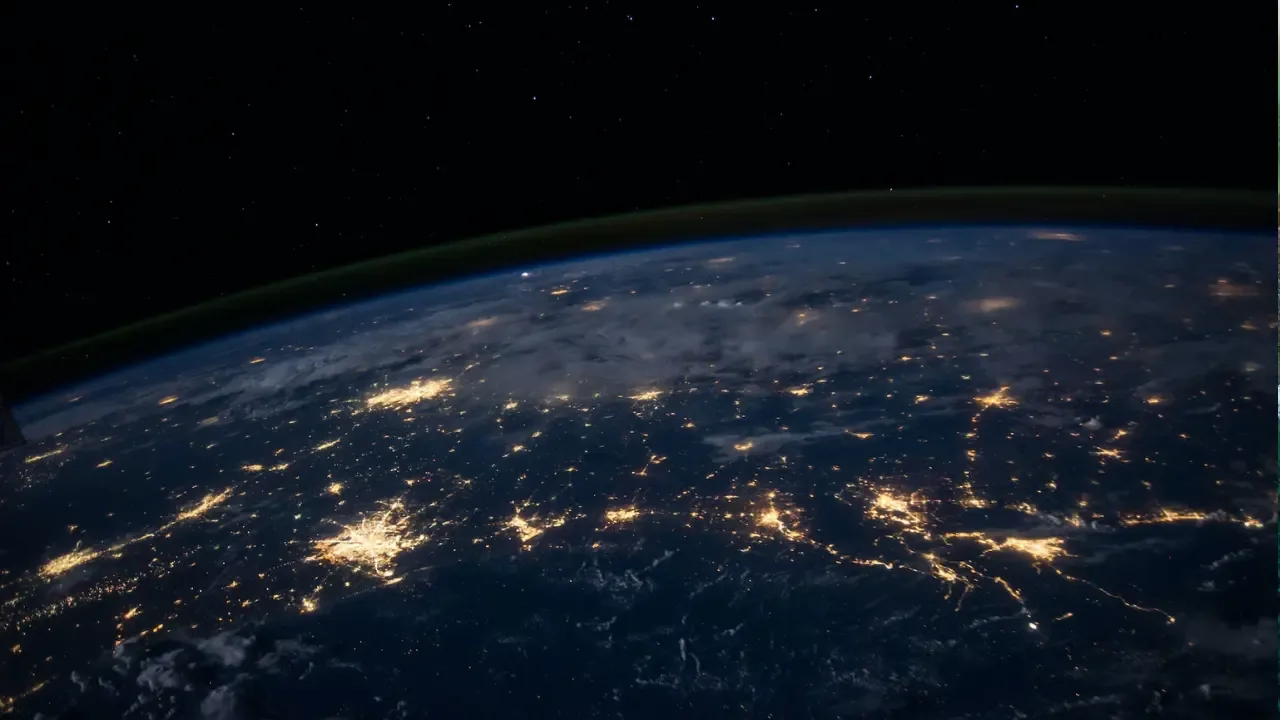
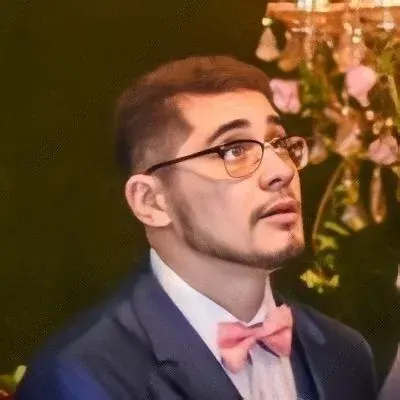
👋 Hey there, tech enthusiasts! Are you ready to level up your socket.io game and unlock the secret to sending private messages to specific clients? 📩💼
So you've already mastered the art of sending messages locally and broadcasting them to all connected clients using the socket.broadcast.emit()
function. 🎉 But now you find yourself wondering how to establish some one-on-one communication, a private chat between just two people. 🤔
Fret not! I'm here to guide you through the process step-by-step, so grab your coding gear and let's dive in! 💻🤓
The Challenge:
You want to send a message to a particular client using socket.io, in order to enable a private chat feature between two individuals. 🗣️💬
The Solution:
To send a private message to a specific client, you need to have the unique identifier of that client's socket. This identifier is usually stored on the server-side, allowing you to target specific clients. Here's how you can do it:
Establish a connection:
Make sure you have socket.io installed and running in your project.
Create a server-side script using Node.js and socket.io, where clients can connect to the server.
Clients should emit an event to the server when they connect, allowing the server to store their socket ID.
Store client socket IDs:
On the server-side, create a data structure (E.g., an object or a map) to store the socket IDs of connected clients.
When a client connects, store its socket ID in this data structure for future reference.
Send a private message:
With the client socket IDs stored, you can send a private message by emitting an event directly to the desired client.
On the server-side, use
io.to(clientSocketID).emit(eventName, data)
to send the private message to the specific client, whereclientSocketID
represents the socket ID of the intended recipient.
Handle the private message on the client-side:
On the client-side, listen for the event you defined in step 3 (
eventName
) and handle the private message accordingly.Voila! You've successfully sent a private message to a particular client using socket.io! 🎊
Example Code:
To make things crystal clear, let's take a look at a simplified example:
Server-side code:
const connectedClients = {};
io.on('connection', (socket) => {
// Store the socket ID of each connected client
connectedClients[socket.id] = socket;
// Handle private message event from the client
socket.on('privateMessage', (data) => {
const recipientSocket = connectedClients[data.recipientSocketId];
if (recipientSocket) {
recipientSocket.emit('privateMessage', {
from: socket.id,
message: data.message,
});
}
});
});
Client-side code:
const socket = io();
// Emit private message to server
function sendPrivateMessage(recipientSocketId, message) {
socket.emit('privateMessage', {
recipientSocketId,
message,
});
}
// Handle private message from server
socket.on('privateMessage', (data) => {
console.log(`Private message from ${data.from}: ${data.message}`);
});
Conclusion:
With this guide, you're now equipped with the knowledge to send private messages to specific clients using socket.io! 🚀💬 Why not add a touch of personalization to your applications and create engaging one-on-one chat features?
So go ahead, experiment, and build amazing real-time communication experiences! And don't forget to share your awesome creations with us in the comments below. Happy coding! 😄👏
If you found this guide helpful, make sure to follow us for more exciting tech tutorials and stay up-to-date with the latest developments in the tech world. 😎🌐