How to search in array of object in mongodb
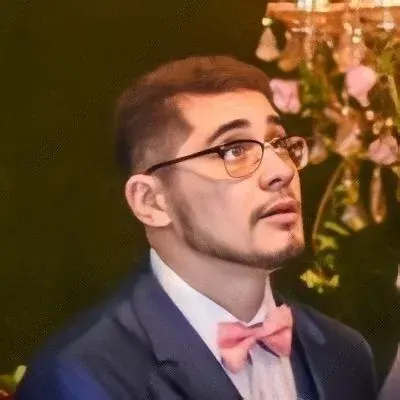
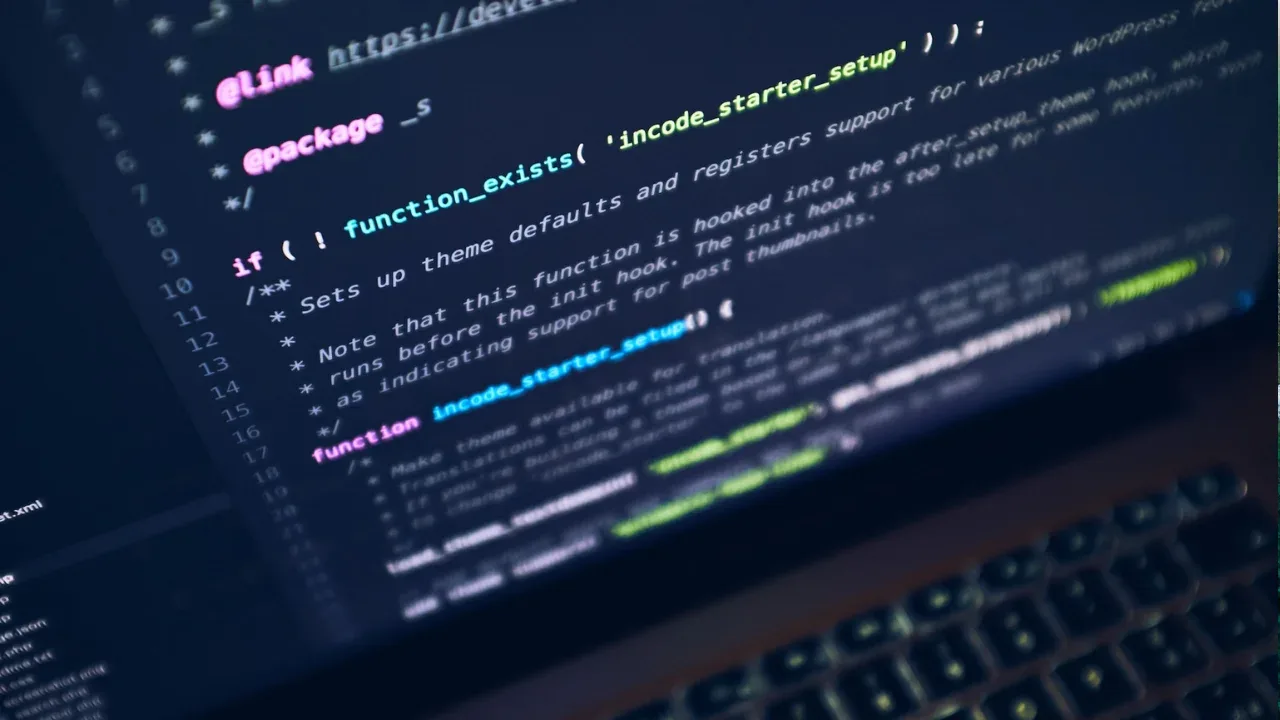
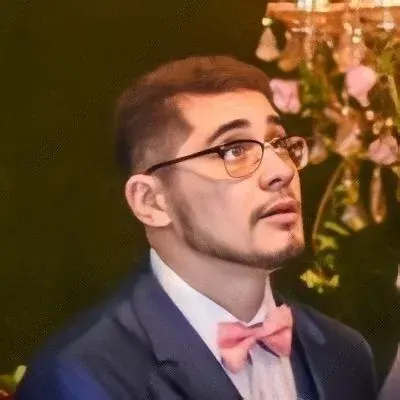
Searching in Array of Objects in MongoDB: Finding the Right Person 🕵️♂️
So you're searching for the person who won the 'National Medal' in 1975 in an array of objects in MongoDB? Look no further! In this blog post, I'll guide you through the process of searching for a specific object in an array using MongoDB queries. 💪
The Context:
Let's start by understanding the structure of your MongoDB document. In this case, we have a collection named 'users', and each user's data is stored as an object. The 'awards' field is an array of objects, with each object representing an award received by a user.
{
"_id": 1,
"name": {
"first": "John",
"last": "Backus"
},
"birth": "Dec 03, 1924",
"death": "Mar 17, 2007",
"contribs": ["Fortran", "ALGOL", "Backus-Naur Form", "FP"],
"awards": [
{
"award": "National Medal",
"year": 1975,
"by": "NSF"
},
{
"award": "Turing Award",
"year": 1977,
"by": "ACM"
}
]
}
Now, let's dive into the solution for finding the right person who won the 'National Medal' in 1975. 🔍
The Query:
To achieve this, we can use MongoDB's powerful query operators. In this case, we'll use the $elemMatch
operator to match objects within the 'awards' array based on multiple conditions.
db.users.find({
awards: {
$elemMatch: {
award: "National Medal",
year: 1975
}
}
})
The $elemMatch
operator ensures that both the 'award' and 'year' fields of the same object from the 'awards' array match the specified values. This way, we'll only get the person who won the 'National Medal' in 1975. 🏅🕺
The Result:
By running the above query, MongoDB will return the person who meets our search criteria. In this case, John Backus is the one we're looking for! 👏
Want more control? Use Projections! 🖥️
If you want to retrieve only specific fields of the matching document, you can use projections. Here's an example:
db.users.find(
{
awards: {
$elemMatch: {
award: "National Medal",
year: 1975
}
}
},
{
"name.first": 1,
"name.last": 1,
_id: 0
}
)
In this case, we're only fetching the first name and last name of the person who won the award, and excluding the _id
field from the result.
Conclusion: ✨
Searching within an array of objects in MongoDB might seem challenging at first, but with the $elemMatch
operator, it becomes a breeze! We've successfully found the person who won the 'National Medal' in 1975, and even learned how to use projections to have more control over the returned data.
Now it's your turn! Put this knowledge into practice and explore the vast possibilities that MongoDB offers. 🚀
If you have any more questions or face any challenges, feel free to leave a comment below. Happy searching! 💙