How to scroll to an element?
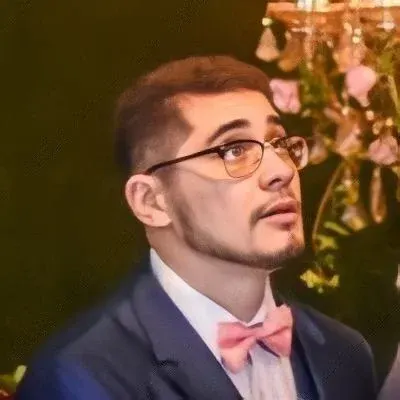
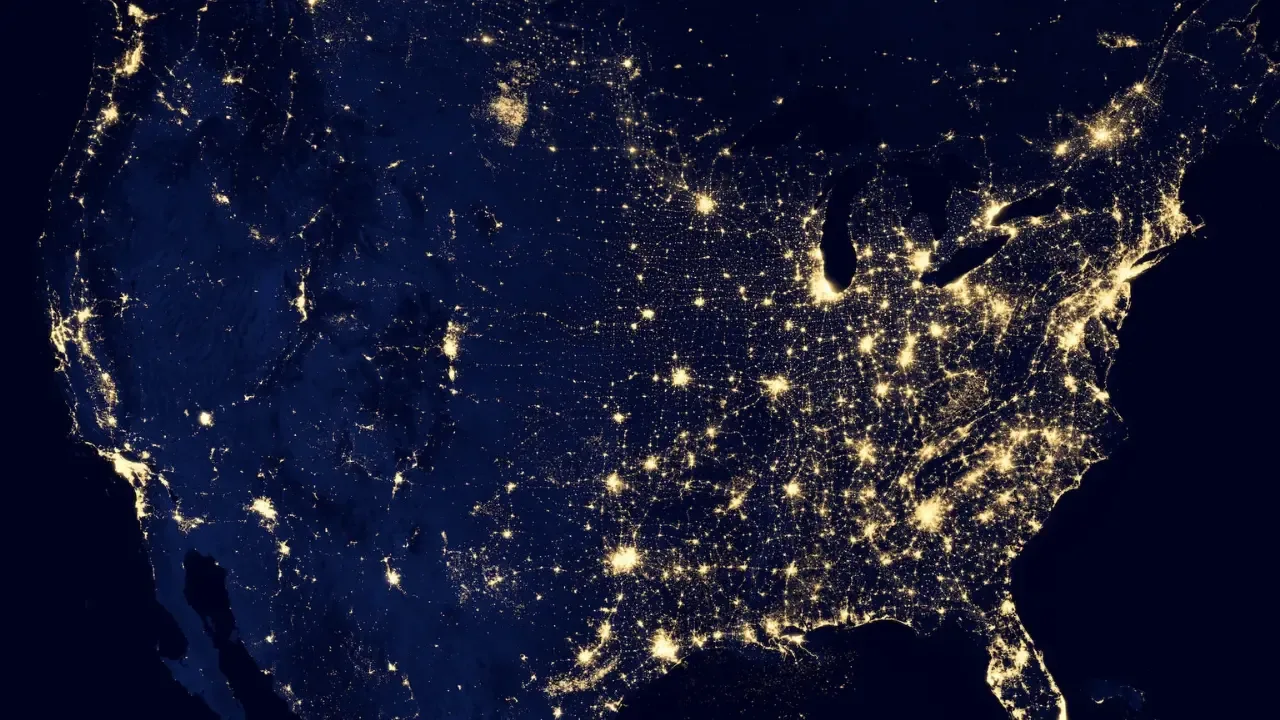
How to Scroll to an Element: A Complete Guide
Have you ever come across a situation where you want to automatically scroll to a specific element on your web page? Maybe you have a chat widget that loads new messages as you scroll up, but you want the scroll position to always focus on the last index element from the previous array. In this guide, we'll explore common issues you might face when scrolling to an element and provide easy solutions to help you achieve your desired result.
The Problem: Fixed Slider Positioning
Let's consider the scenario mentioned above. You have a chat widget with a slider that stays fixed at the top while messages load. The challenge is to make the slider focus on the last index element from the previous array every time new messages are loaded. You've already figured out how to create dynamic refs by passing an index, but you need to know what kind of scroll function to use to accomplish this.
The Solution: scrollTo()
To achieve the desired scroll functionality, we can utilize the scrollTo()
function. This function allows you to smoothly scroll to a specific element on your webpage. Here's how you can implement it:
handleScrollToElement(event) {
const testNode = ReactDOM.findDOMNode(this.refs.test);
if (some_logic) {
window.scrollTo({
top: testNode.offsetTop, // Scroll to the top position of the 'test' element
behavior: 'smooth', // Enable smooth scrolling effect
});
}
}
render() {
return (
<div>
<div ref="test"></div>
</div>
);
}
In the above code snippet, we first find the DOM Node for the element with the ref test
using ReactDOM.findDOMNode()
. Then, within the handleScrollToElement()
function, we use the window.scrollTo()
method to scroll to the top position of the test
element. The smooth
behavior option adds a smooth scrolling effect for a better user experience.
💡 Pro Tip: Use offsetHeight to Scroll to the Bottom
If you want to scroll to the bottom of an element instead of the top, you can make a small adjustment to the code. Instead of using offsetTop
, you can use offsetHeight
of the element to automatically scroll to the bottom position. Here's an example:
handleScrollToBottom(event) {
const testNode = ReactDOM.findDOMNode(this.refs.test);
if (some_logic) {
window.scrollTo({
top: testNode.offsetTop + testNode.offsetHeight, // Scroll to the bottom position of 'test' element
behavior: 'smooth', // Enable smooth scrolling effect
});
}
}
You can see here that we added testNode.offsetHeight
to the top
property of window.scrollTo()
. This ensures that the scroll position will be adjusted to the bottom position of the test
element.
Test it Out!
Now, try implementing the provided code snippets into your project and see how the scroll functionality works. You should be able to effortlessly scroll to the desired element, whether it's at the top or bottom of the page.
Share Your Experience!
We hope this guide has helped you overcome the issue of scrolling to an element on your web page. If you have any questions or face any difficulties during implementation, feel free to reach out in the comments section. Share your experience and let us know if there are any other topics you'd like us to cover!
Happy scrolling! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
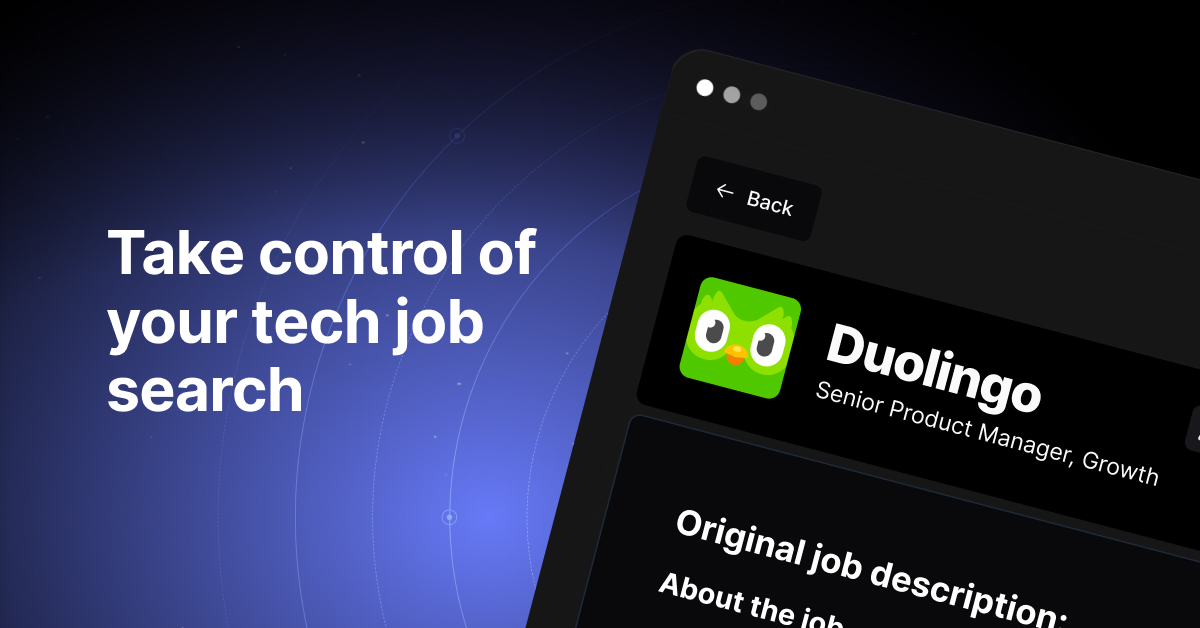