How to save an HTML5 Canvas as an image on a server?
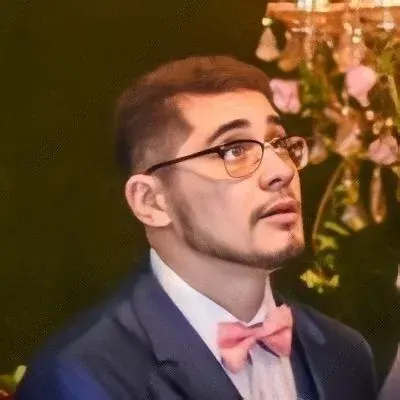
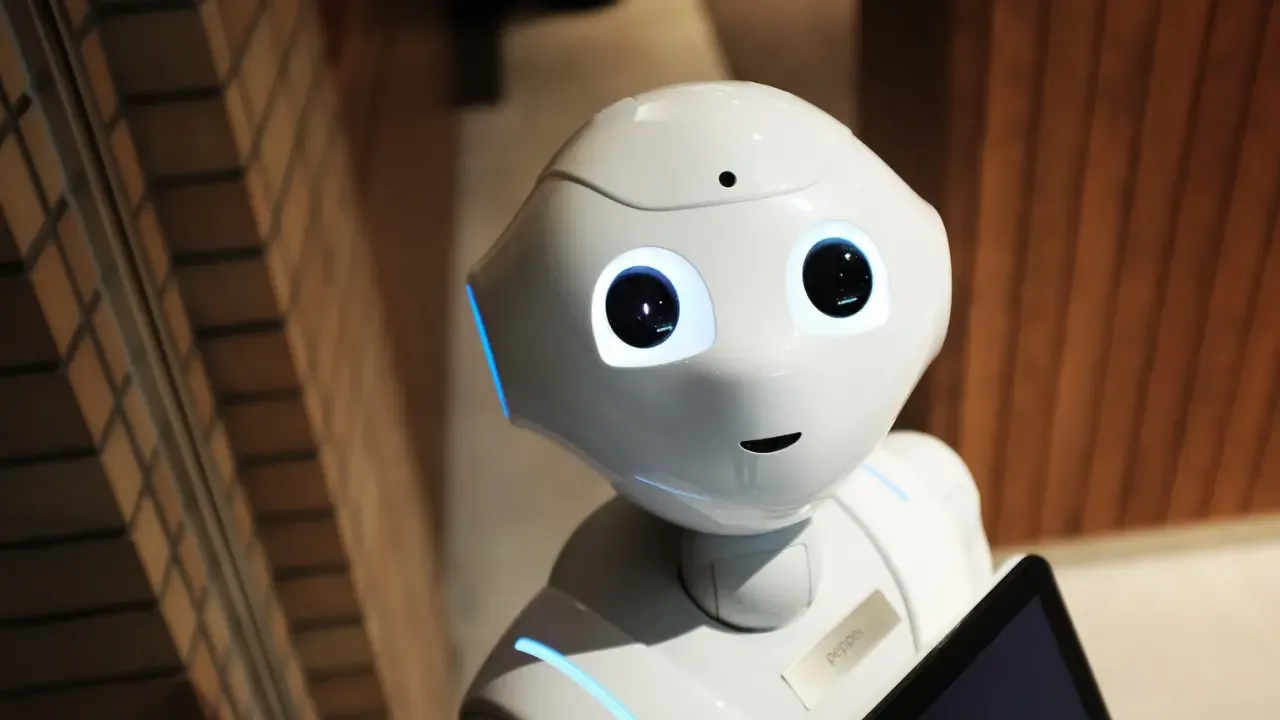
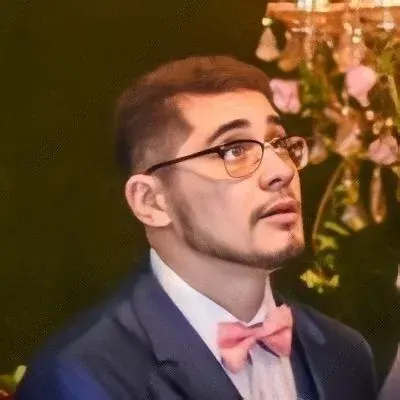
How to Save an HTML5 Canvas as an Image on a Server? 🎨💾
Have you ever worked on a cool generative art project and wanted to allow users to save the resulting images from an algorithm? 🌌🖼️ Don't worry, you're not alone. Many developers face this challenge when working with HTML5 Canvas. In this blog post, we will explore how to save an HTML5 Canvas as an image on a server 🖥️📁.
The Challenge 🔍
You've followed some tutorials and tried to implement the code found online, but you're stuck on the second step. The code you found doesn't seem to be working as expected. 😕
The Solution 💡
Let's break down the process step by step, and I'll provide you with an easy solution.
Creating an Image on an HTML5 Canvas 🖌️ Use your generative algorithm to create a visually appealing image on the HTML5 Canvas. Make sure you have the Canvas element set up correctly and that your algorithm generates the desired output.
Saving the Canvas as an Image File 💾 To save the Canvas as an image, you can use the
canvas.toDataURL()
method, which returns a data URL representing the Canvas image. The most common format is PNG, but you can also choose JPEG or others. Here's an example code snippet:
function saveImage() {
var canvasData = canvas.toDataURL("image/png");
var ajax = new XMLHttpRequest();
ajax.open("POST", "saveImage.php", false);
ajax.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
ajax.onreadystatechange = function() {
if (ajax.readyState === 4 && ajax.status === 200) {
console.log(ajax.responseText);
}
};
ajax.send("imageData=" + encodeURIComponent(canvasData));
}
In the above code, we create a new XMLHttpRequest and open a POST request to a PHP file named saveImage.php
. We set the Content-Type header to "application/x-www-form-urlencoded"
to correctly send the data. We also handle the onreadystatechange
event to check if the request is complete and successful. Finally, we send the Canvas data as a parameter named "imageData"
.
Handling the Server-Side Code 🖥️📝 On the server-side, you need to handle the request and save the received data as an image file. In this example, we'll use PHP to handle the request. Here's an example PHP code snippet:
<?php
if (isset($_POST['imageData'])) {
$imageData = $_POST['imageData'];
$filteredData = substr($imageData, strpos($imageData, ",") + 1);
$unencodedData = base64_decode($filteredData);
$fileName = '/path/to/save/directory/' . uniqid() . '.png';
$file = fopen($fileName, "wb");
fwrite($file, $unencodedData);
fclose($file);
echo $fileName;
}
?>
In the above code, we check if the POST parameter "imageData"
is set. Then, we extract the Base64-encoded data from the URL by removing the data-type prefix. Next, we decode the Base64 data into its original binary representation. Finally, we save the decoded data as a PNG file in the specified directory with a unique filename (you can modify this according to your needs).
Troubleshooting Tips and Debugging 🔧🐛
Make sure the server-side directory for saving the image has proper write permissions to allow file creation.
Check your server configuration for any restrictions on file uploads or content types.
Use browser developer tools to check for any errors or status codes in the Network tab.
Print debug information on both the client and server-side to identify any issues during the process.
Conclusion and Call to Action 🏁🔗
Congrats, you've learned how to save an HTML5 Canvas as an image on a server! Now, you can empower your generative art project to allow users to save their creations. 🎉👩🎨
If you found this guide helpful, please share it with your fellow developers and artists. Also, feel free to leave a comment below and let me know how this solution worked for you or if you have any other questions or suggestions. I'd love to hear from you! 😊💬
Happy coding and keep creating fantastic generative art! 🎨✨