How to run Gulp tasks sequentially one after the other
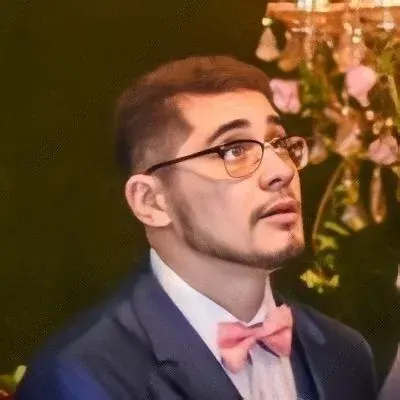
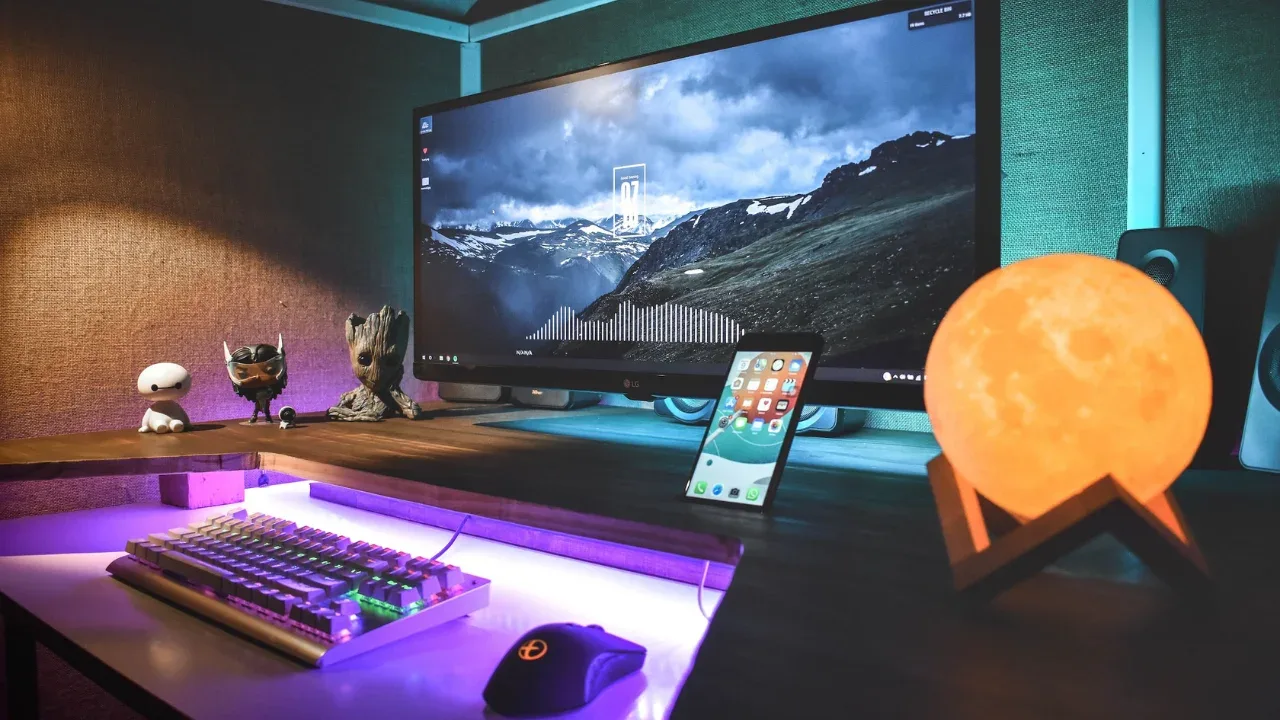
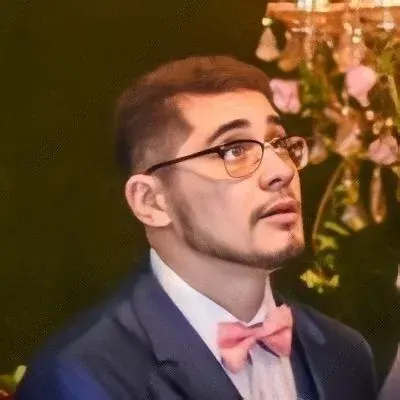
How to Run Gulp Tasks Sequentially: An Easy Solution 😎
Do you find yourself struggling to run Gulp tasks sequentially, one after the other? You're not alone! It's a common issue that can be quite frustrating. But fear not, because in this blog post, we're going to provide you with an easy solution to this problem.
Understanding the Problem 🤔
Let's take a closer look at the code snippet provided:
gulp.task("coffee", () => {
gulp.src("src/server/**/*.coffee")
.pipe(coffee({bare: true})).on("error", gutil.log)
.pipe(gulp.dest("bin"))
});
gulp.task("clean", () => {
gulp.src("bin", {read: false})
.pipe(clean({force: true}));
});
gulp.task("develop", ["clean", "coffee"], () => {
console.log("run something else");
});
The intention here is to run the clean
task first, then the coffee
task, and finally, do something else in the develop
task. However, as the original poster mentioned, running the code as it is doesn't achieve the desired sequential execution.
The Easy Solution 🚀
To make Gulp tasks run sequentially, we can leverage the power of Gulp series or Gulp 4's gulp.series(). Here's how you can modify the code to achieve the desired outcome:
// Require the 'gulp' and 'next' modules
const gulp = require('gulp');
const next = require('gulp-next');
gulp.task("coffee", () => {
return gulp.src("src/server/**/*.coffee")
.pipe(coffee({bare: true})).on("error", gutil.log)
.pipe(gulp.dest("bin"))
});
gulp.task("clean", () => {
return gulp.src("bin", {read: false})
.pipe(clean({force: true}));
});
gulp.task("develop", gulp.series("clean", "coffee", () => {
console.log("run something else");
}));
// Register the default task
gulp.task("default", gulp.series("develop"));
// Exporting the gulp object for external use
module.exports = gulp;
Now, when you run the gulp develop
command, it will run the tasks sequentially in the order specified. The develop
task will ensure that clean
task runs first, followed by the coffee
task, and finally, perform the desired action.
An Example to Make it Crystal Clear 💡
Let's say you have a project where you compile CoffeeScript code, clean the bin
directory, and then run some tests. You can modify the code like this:
gulp.task("coffee", () => {
return gulp.src("src/server/**/*.coffee")
.pipe(coffee({bare: true})).on("error", gutil.log)
.pipe(gulp.dest("bin"))
});
gulp.task("clean", () => {
return gulp.src("bin", {read: false})
.pipe(clean({force: true}));
});
gulp.task("test", () => {
return gulp.src("bin/**/*.js") // Assuming compiled JS goes to 'bin' directory
.pipe(mocha());
});
gulp.task("build", gulp.series("clean", "coffee"));
gulp.task("default", gulp.series("build", "test"));
// Exporting the gulp object for external use
module.exports = gulp;
By creating a build
task that runs the clean
and coffee
tasks in sequence, and then making the default
task run the build
and test
tasks in order, you now have a beautifully organized and sequential Gulp task pipeline. You can just run gulp
in the command line and watch everything work seamlessly.
Conclusion 🌟
Running Gulp tasks sequentially doesn't have to be a headache. By using Gulp's built-in series functionality or the gulp-next
package, you can easily solve this problem and streamline your task pipeline.
Now that you know how to run Gulp tasks sequentially, go ahead and boost your productivity! Give it a try and let us know your thoughts and feedback in the comments below. Happy Gulp-ing! 🎉