How to run function in AngularJS controller on document ready?
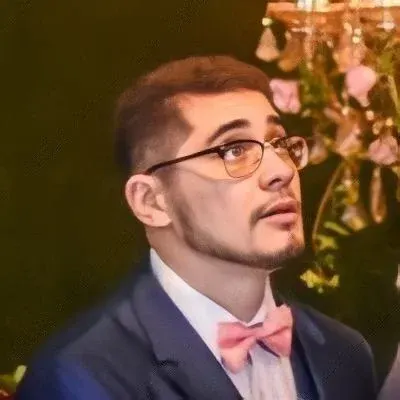
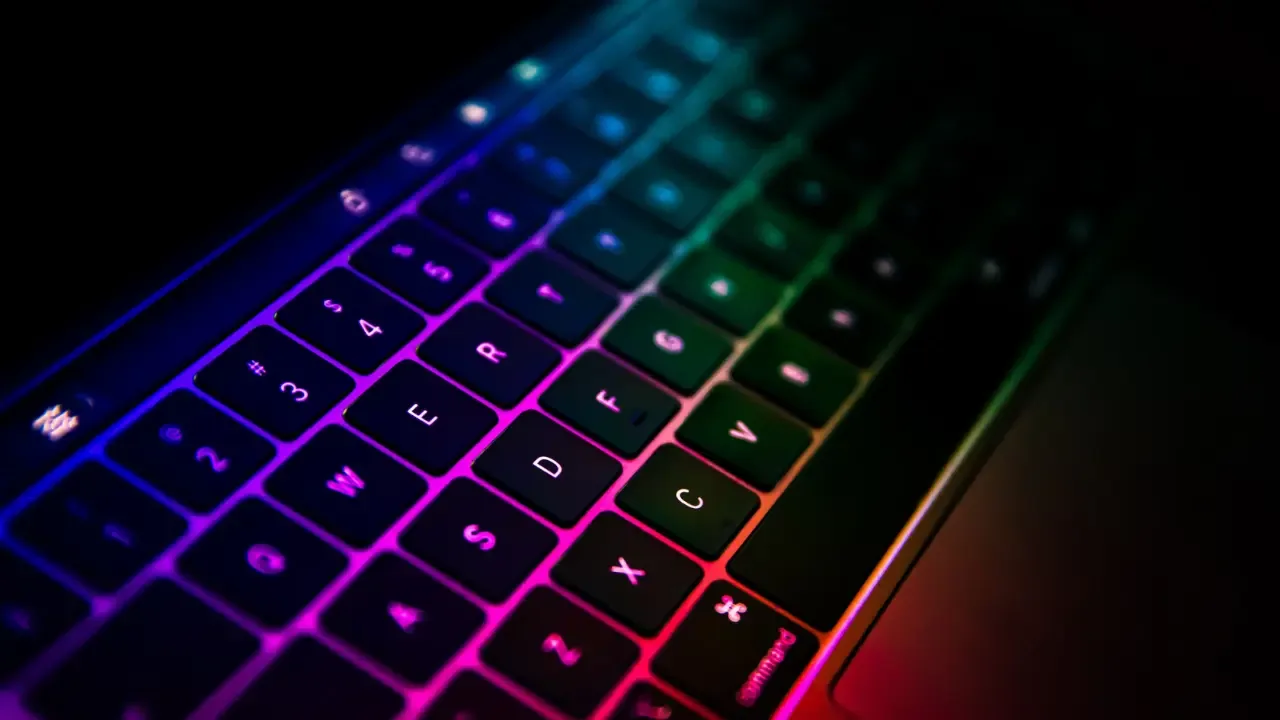
How to run function in AngularJS controller on document ready?
š¤ Have you ever found yourself in a situation where you wanted to run a function in your AngularJS controller when the document is ready? š You might have noticed that AngularJS executes functions as soon as the DOM elements are created, which doesn't always align with our needs. But worry not, my friend! I'm here to guide you through this common issue and provide you with some easy solutions.
The Problem
š Let's first understand the issue at hand. You have a function within your Angular controller that you want to run when the document is fully loaded. However, the current implementation executes the function before the page has completely loaded. š
š Here's the code snippet you provided:
function myController($scope) {
$scope.init = function() {
// I'd like to run this on document ready
}
$scope.init(); // doesn't work, loads my init before the page has completely loaded
}
š As you can see, the $scope.init
function is being called immediately, which prevents it from running when the document is ready. So, how can we solve this problem? Let's explore some options!
Solution 1: AngularJS $timeout
ā±ļø One of the solutions is to make use of the AngularJS $timeout
service. By leveraging the $timeout
service, you can delay the execution of your function, ensuring that it runs when the DOM is fully loaded.
function myController($scope, $timeout) {
$scope.init = function() {
// Your initialization code here
}
$timeout(function() {
$scope.init();
});
}
š In this solution, we inject the $timeout
service into our controller and utilize it to delay the execution of the $scope.init
function. By wrapping the function call inside the $timeout
callback, we ensure that it is triggered after the DOM has loaded.
š” Additionally, you can pass an optional delay value as the second argument to $timeout
if you need to introduce a delay before running the function.
Solution 2: AngularJS ng-init
š Another straightforward solution is to use the ng-init
directive provided by AngularJS. This directive allows you to initialize variables and run expressions on an element when it is instantiated. By attaching the ng-init
directive to your desired element, you can execute your function on document ready.
<div ng-controller="myController" ng-init="init()">
<!-- Your content here -->
</div>
š In this solution, we define the ng-controller
directive to bind your controller to the HTML element. Additionally, the ng-init
directive is attached to the same element, executing the init()
function when the element is instantiated.
š” Note that using the ng-init
directive can introduce some coupling between your controller and the view. So, use it judiciously and consider it only for simple initialization tasks.
Conclusion
š Dealing with functions in AngularJS controllers that need to be executed on document ready can be a bit tricky. But with the help of the AngularJS $timeout
service or the ng-init
directive, you can easily overcome this obstacle. š
šŖ So, whether you want to introduce a delay using $timeout
or tap into the ng-init
directive, you now have two reliable solutions at your disposal.
š I hope this guide was helpful in resolving your query. If you have any further questions or other AngularJS challenges you'd like assistance with, feel free to reach out! Let's keep coding and building awesome things together! š¤
⨠And, don't forget to share this post with other AngularJS enthusiasts who might be facing similar issues. Sharing is caring! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
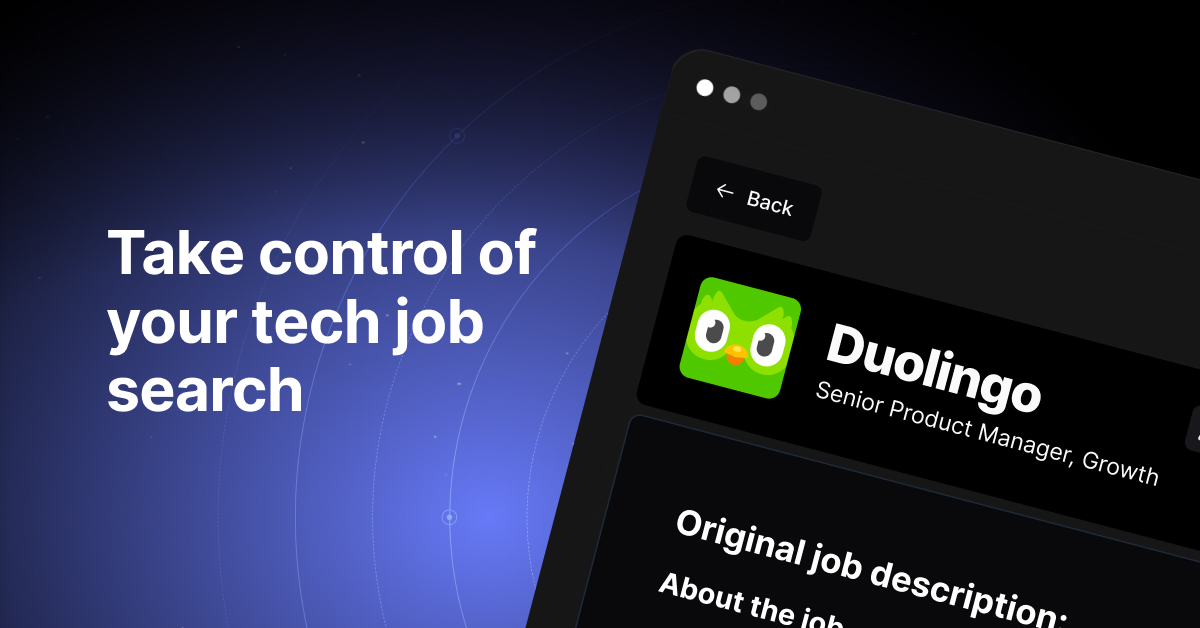