How to replace plain URLs with links?
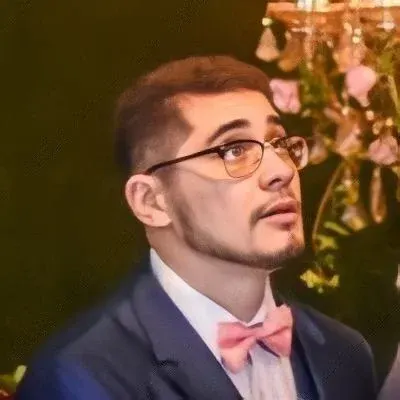

How to Replace Plain URLs with Links
Are you tired of plain, boring URLs cluttering up your text? Do you want to make your content look more professional and visually appealing? Well, you're in luck! In this blog post, we'll show you an easy solution to replace those plain URLs with clickable HTML links. 🌐💻
The Problem
A user reached out to us with a common issue. They had a function that successfully matched URLs within a given text and replaced them with HTML links. However, there was one problem - it only replaced the first match. 😱
The Solution
To replace all occurrences of URLs in a text, we need to modify the code to use the exec
method instead of replace
. Let's take a look at the updated code:
function replaceURLWithHTMLLinks(text) {
var exp = /(\b(https?|ftp|file):\/\/[-A-Z0-9+&@#\/%?=~_|!:,.;]*[-A-Z0-9+&@#\/%=~_|])/ig;
var match;
var replacedText = text;
while ((match = exp.exec(text)) !== null) {
replacedText = replacedText.replace(match[0], `<a href="${match[0]}">${match[0]}</a>`);
}
return replacedText;
}
By using a while
loop and the exec
method, we can ensure that all occurrences of URLs are replaced with HTML links. The regular expression now includes the g
flag at the end (/ig
) to make it globally match all instances.
Example
Let's see our function in action. Imagine we have the following text:
var text = "Check out my website at https://www.example.com. Also, don't forget to visit our forum at https://www.example.com/forum.";
console.log(replaceURLWithHTMLLinks(text));
The output will be:
Check out my website at <a href="https://www.example.com">https://www.example.com</a>. Also, don't forget to visit our forum at <a href="https://www.example.com/forum">https://www.example.com/forum</a>.
Voilà! All URLs have been replaced with clickable HTML links.
Take It a Step Further
Now that you have learned how to replace URLs with links, why stop there? You can enhance this function by adding additional features, such as:
Open links in a new tab/window:
<a href="${match[0]}" target="_blank">${match[0]}</a>
Display a custom text instead of the URL:
<a href="${match[0]}">Click here</a>
The possibilities are endless! Get creative and make your links stand out.
Conclusion
Don't let plain URLs dull your content. Spice it up with clickable HTML links using our simple solution. Remember to use the updated function code we provided and explore additional customizations to make it your own.
Now it's your turn! Give it a try and let us know how it goes. Feel free to share your experience in the comments section below. Happy linking! 😄🔗
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
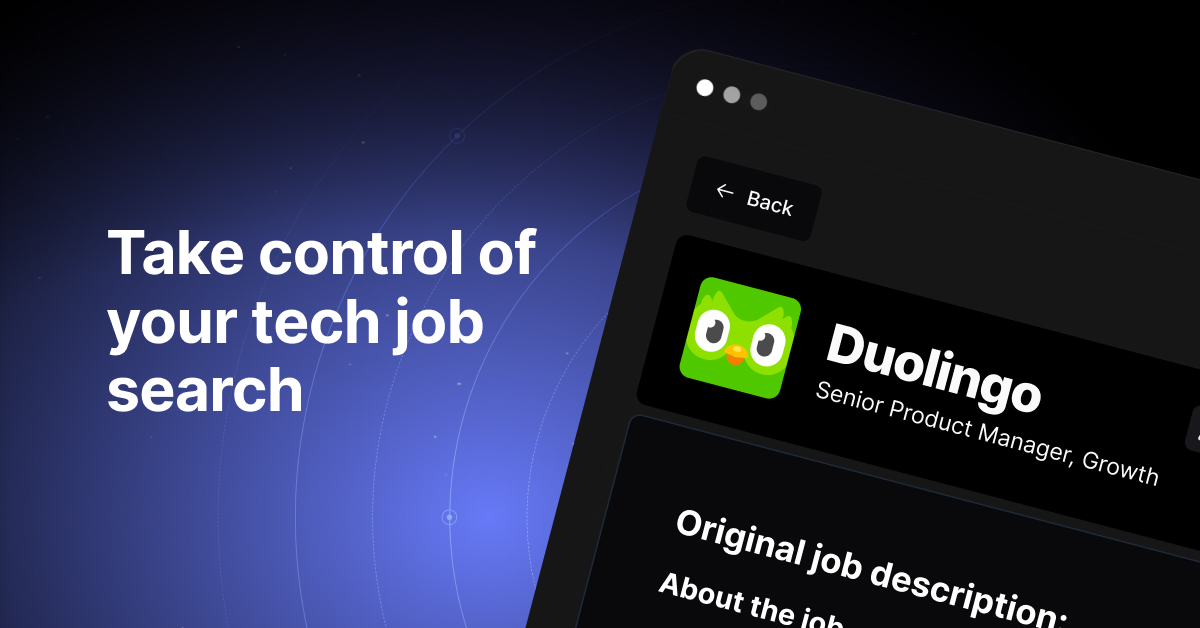