How to replace item in array?
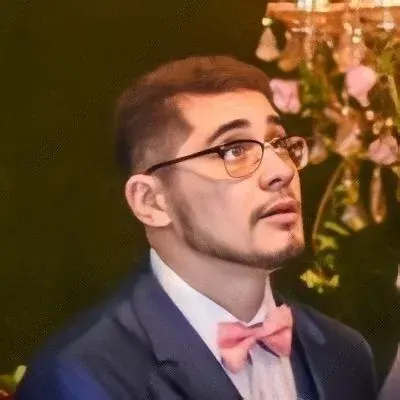
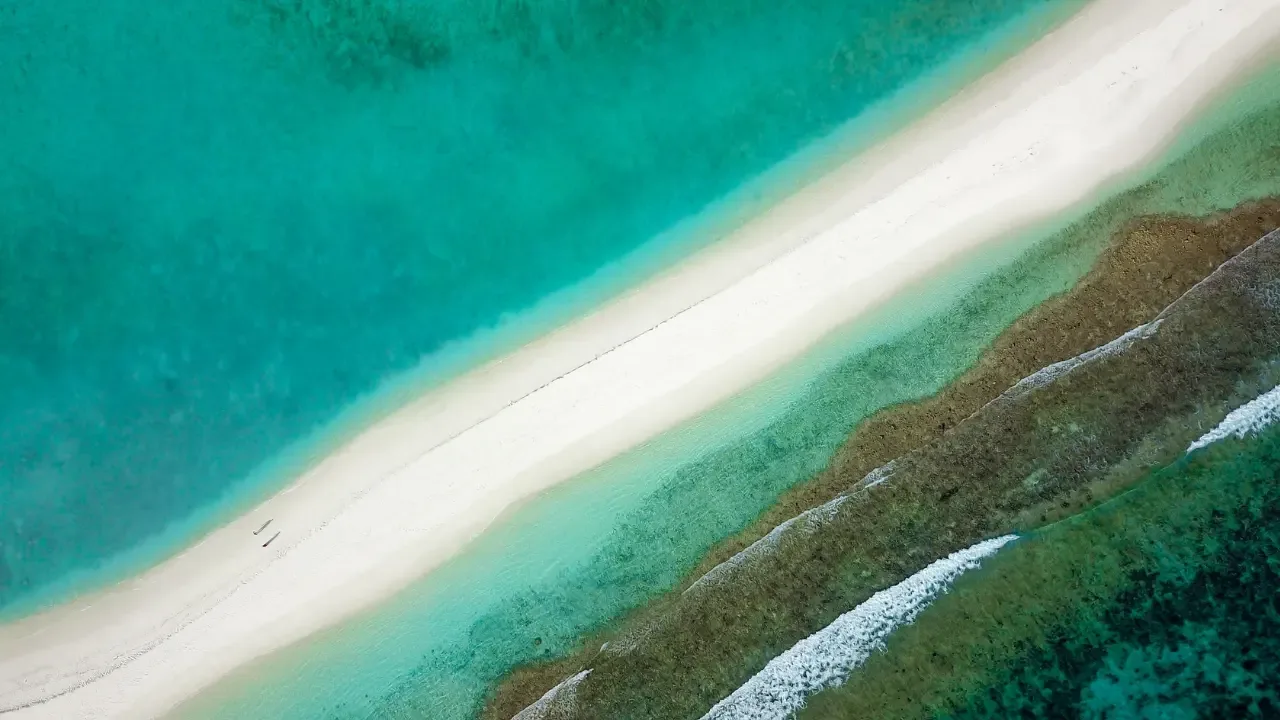
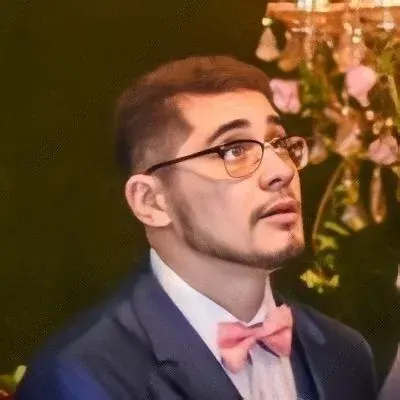
πππHow to Replace an Item in an Array?πππ
Do you find yourself needing to replace an item in an array? Don't worry, we've got you covered with some easy solutions to this common problem! π οΈπ‘
Let's dive right in with an example to make things crystal clear. Imagine you have the following array:
var items = [523,3452,334,31, ...5346];
You want to replace the item 3452
with a new value of 1010
. How can you accomplish this task? π€
π‘ Solution 1: Using the array index
One way to replace an item in an array is by accessing it through its index. In JavaScript, arrays are zero-indexed, meaning the first element has an index of 0, the second element has an index of 1, and so on. To replace an item, you can simply assign a new value to its corresponding index.
Here's how you can replace 3452
with 1010
using the array index:
items[1] = 1010;
In this example, we're using index 1
because 3452
is the second item in the array. Remember, index starts at 0!
π‘ Solution 2: Using the Array.prototype.findIndex() method
Another option is to use the findIndex()
method provided by JavaScript arrays. This method returns the index of the first element in the array that satisfies a given condition. We can then use this index to replace the desired item.
Here's how you can use findIndex()
to replace 3452
with 1010
:
var index = items.findIndex(item => item === 3452);
items[index] = 1010;
In this example, we're using an arrow function to compare each item in the array with 3452
until a match is found. Once we have the index, we can replace the item with 1010
just like in Solution 1.
β¨Conclusion and Reader Engagementβ¨
Now you know how to replace an item in an array using two different approaches. So go ahead and give it a try in your own projects! πͺ
If you found this guide helpful, be sure to share it with your developer friends and spread the knowledge. π₯π£
Have you ever had difficulties replacing items in an array? How did you solve the problem? Let us know in the comments below! π¬π
Happy coding! π»π