How to replace captured groups only?
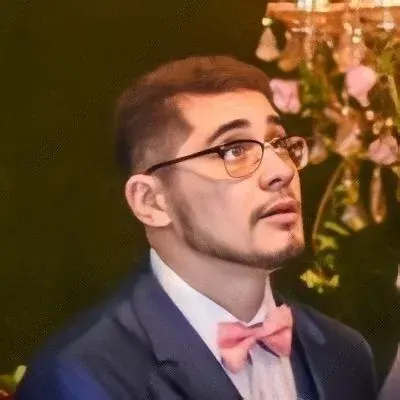
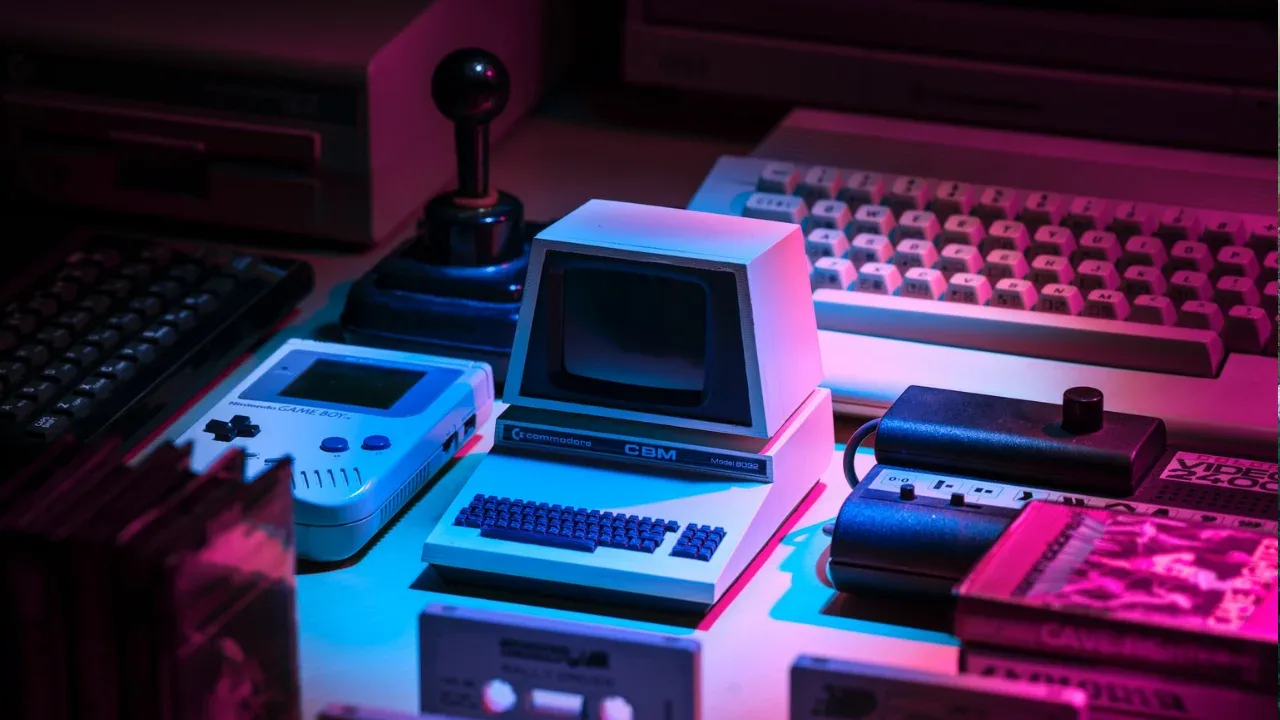
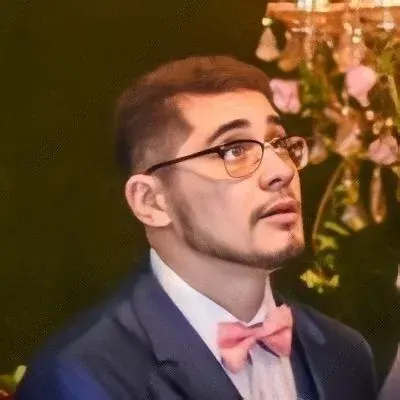
How to Replace Captured Groups Only: A Guide for Regex Newbies
š TL;DR: Looking to replace a specific part of a string using captured groups in regex? We've got you covered! Follow our step-by-step guide below to easily replace captured groups and give your strings a fresh new look. š
Introduction
Regex can be a powerful tool for manipulating strings, but it can also leave you scratching your head when it comes to replacing only specific parts of a captured group. In this guide, we'll address a common issue faced by regex newbies and provide you with easy solutions to replace captured groups without messing up the rest of your string. Let's get started! šŖ
The Problem
Imagine you have a snippet of HTML code that contains a string you want to modify. Specifically, you want to replace the numeric value in a certain section with a new ID, but preserve the rest of the string intact. Here's an example:
name="some_text_0_some_text"
You want to replace the 0
with a new ID, let's say !NEW_ID!
. š
The Regex Solution
To achieve this, you'll need to use regex to capture the desired part of the string and replace it with your new value. In this case, we'll use the (\d+)
pattern to capture the numeric portion. Here's the regex pattern for the entire code snippet:
.*name="(\w+(\d+)\w+)".*
Let's break it down:
.*
matches any characters before the target string.name="
matches the literal textname="
.(\w+(\d+)\w+)
captures the desired part of the string (0
in this case) while preserving the surrounding text.".*
matches any characters after the target string.
The Solution
Now that we've captured the desired part of the string, we can replace it with our new value !NEW_ID!
. Here's how you can achieve this in different programming languages:
JavaScript
const originalString = 'name="some_text_0_some_text"';
const newId = '!NEW_ID!';
const modifiedString = originalString.replace(/(.*name="\w+)(\d+)(\w+".*)/, `$1${newId}$3`);
console.log(modifiedString);
Python
import re
original_string = 'name="some_text_0_some_text"'
new_id = '!NEW_ID!'
modified_string = re.sub(r'(.*name="\w+)(\d+)(\w+".*)', fr'\g<1>{new_id}\g<3>', original_string)
print(modified_string)
š Voila! You have successfully replaced the captured group. The modified string will now look like this:
name="some_text_!NEW_ID!_some_text"
Conclusion
Replacing captured groups in regex doesn't have to be complicated. By using the right regex pattern and understanding how to utilize the capturing groups, you can effortlessly modify specific parts of a string without affecting the rest. Now it's time to unleash your regex powers and transform your strings like a pro! š„
If you found this guide helpful, feel free to share it with your friends! And if you have any questions or suggestions, let us know in the comments below. Happy coding! š