How to remove item from array by value?
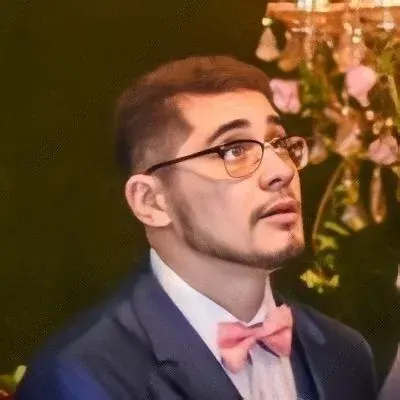
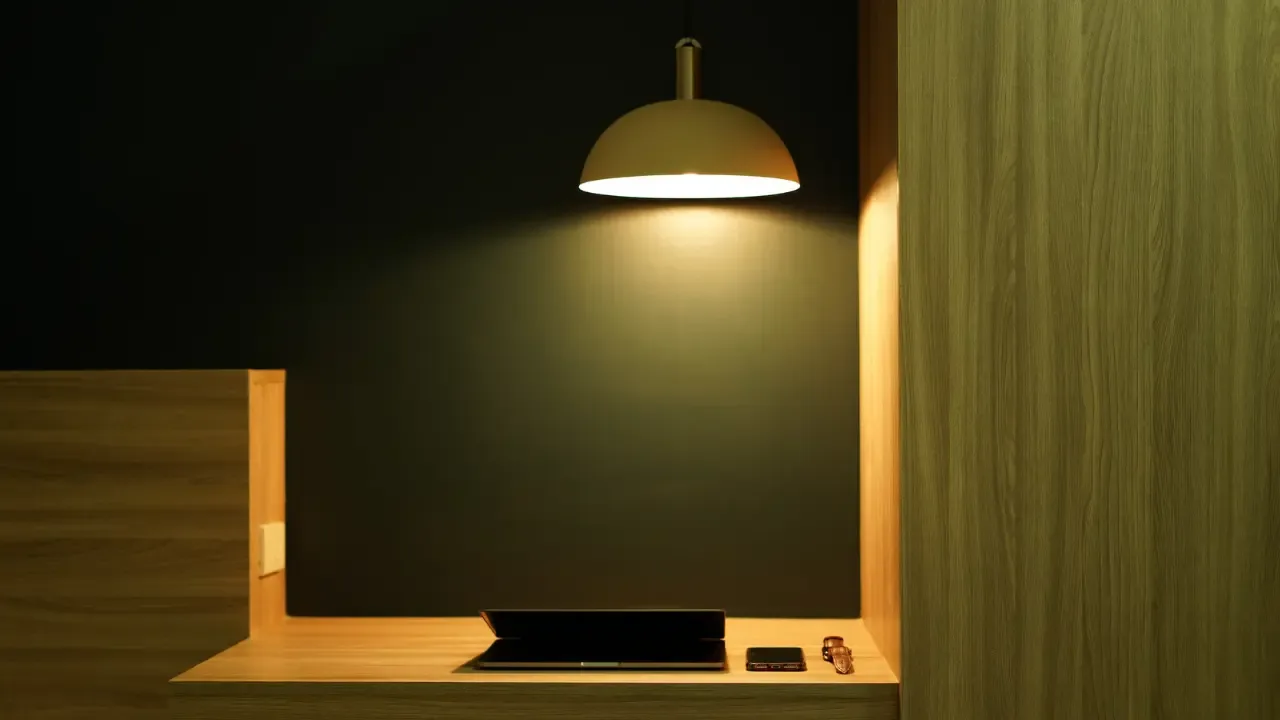
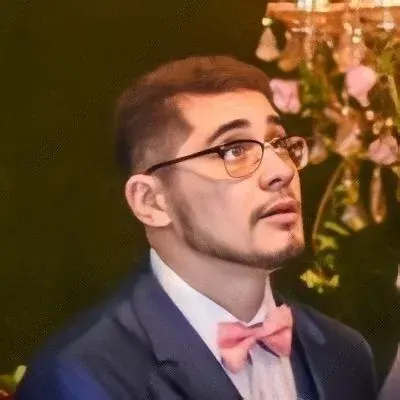
Hey there, tech enthusiasts! 😎
Do you find yourself wondering how to remove an item from a JavaScript array by its value? You're not alone! Many developers face this common conundrum. But fear not! 🙌 In this blog post, we'll dive into easy solutions to help you conquer this challenge and streamline your coding process. So, let's get started! 🚀
The first thing we need to understand is that JavaScript arrays have built-in methods, including splice()
, which allows us to remove elements by their position within the array. However, in this case, we require a method that deletes an item based on its value. Let's explore a few solutions that will do just that! 🧐
Solution 1: Using the .filter()
method
One simple yet effective way to remove an item from an array by its value is by utilizing the .filter()
method. This method creates a new array that contains only the elements that pass a specific test. Here's how you can implement it:
function removeItem(value, array) {
return array.filter(function(item) {
return item !== value;
});
}
var array = ['three', 'seven', 'eleven'];
var newArray = removeItem('seven', array);
console.log(newArray);
In this example, we define a function called removeItem()
that takes the desired value and the array as arguments. Inside the function, we use the .filter()
method to return a new array with all elements except the one matching the provided value. Lastly, we assign the filtered array to newArray
and log it to the console.
Solution 2: Using a for
loop
Another approach is to iterate over the array using a for
loop and remove the item by its value manually. Here's how it can be done:
function removeItem(value, array) {
for (var i = 0; i < array.length; i++) {
if (array[i] === value) {
array.splice(i, 1);
i--;
}
}
return array;
}
var array = ['three', 'seven', 'eleven'];
var newArray = removeItem('seven', array);
console.log(newArray);
In this example, we define a function called removeItem()
that takes the desired value and the array as arguments. Inside the function, we use a for
loop to iterate over the array and check if an element matches the provided value. If we find a match, we use the splice()
method to remove the item and decrement the i
variable to avoid skipping the next element. Finally, we return the updated array.
Engage with us!
We hope these solutions have provided you with easy and practical ways to remove an item from a JavaScript array by its value. Give them a try in your code and let us know which method you prefer! Have any other techniques or questions? Share your thoughts and join the conversation in the comments below. Your engagement is what makes our tech community thrive! 👍💬
Don't forget to follow our blog for more exciting tech tips and tricks! Until next time, happy coding! 🖥️💻✨