How to remove all duplicates from an array of objects?
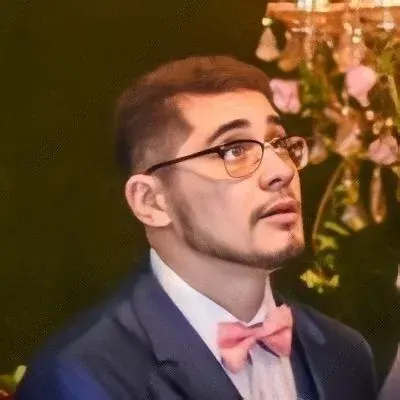
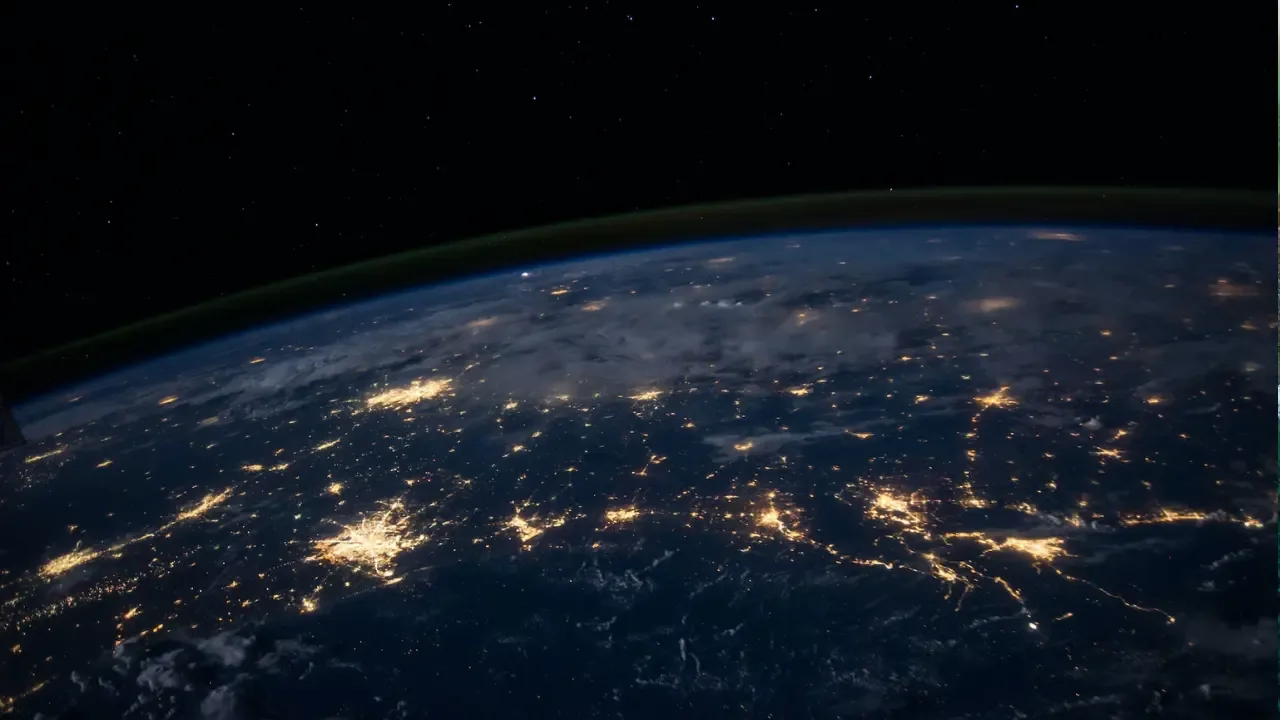
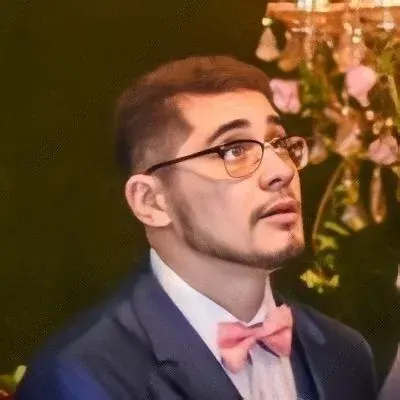
How to Remove All Duplicates from an Array of Objects
Are you tired of dealing with duplicate objects cluttering up your array? Fret not, my friend! In this blog post, we'll dive into the world of arrays and objects to find the best method to remove those pesky duplicates.
Before we jump into the solution, let's take a moment to understand the problem. In the provided context, we have an object that contains an array of objects. Here's a quick snippet to paint a clearer picture:
obj = {};
obj.arr = new Array();
obj.arr.push({place:"here",name:"stuff"});
obj.arr.push({place:"there",name:"morestuff"});
obj.arr.push({place:"there",name:"morestuff"});
As you can see, we have an array of objects where some of them are duplicates. Our goal is to remove these duplicates and have a clean, duplicate-free array.
The Solution: Unique and Sorted Arrays
To achieve this, we'll leverage JavaScript's powerful array methods. One such method is the filter()
method, which comes in handy for our task. We'll use this method to filter out the duplicate objects. Here's how:
const uniqueArray = obj.arr.filter((item, index, arr) => {
const firstIndex = arr.findIndex((duplicate) =>
duplicate.place === item.place && duplicate.name === item.name
);
return index === firstIndex;
});
Let's break it down. By using the filter()
method, we pass a callback function that accepts three parameters: item
, index
, and array
(which in this case is obj.arr
). Inside the callback function, we use the findIndex()
method to find the first occurrence of the object in the array with the same place
and name
properties.
If the current index matches the index of the first occurrence, it means that the object is unique and should be included in our resulting array. Otherwise, it should be filtered out.
After executing the above code, you'll have a gorgeous, duplicate-free uniqueArray
ready for use. 🎉
Let's Put It into Action
To see the magic happen, let's take a look at the resulting uniqueArray
after applying the solution to our initial problem:
{place:"here", name:"stuff"},
{place:"there", name:"morestuff"}
Hurray! All duplicates have been removed, and we have a tidy array showcasing the unique objects.
Share Your Thoughts!
Have you ever struggled with removing duplicates from an array of objects before? What other JavaScript tips or tricks would you like us to cover? Let us know in the comments section below! We'd love to hear from you and continue providing content that solves your coding conundrums.
Now that you're armed with the knowledge to tackle this problem head-on, go forth and code fearlessly! Happy coding! ✨🚀