How to reload or re-render the entire page using AngularJS
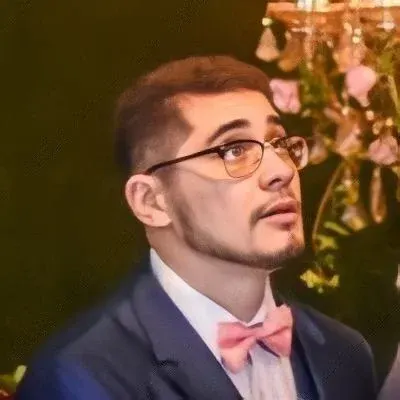
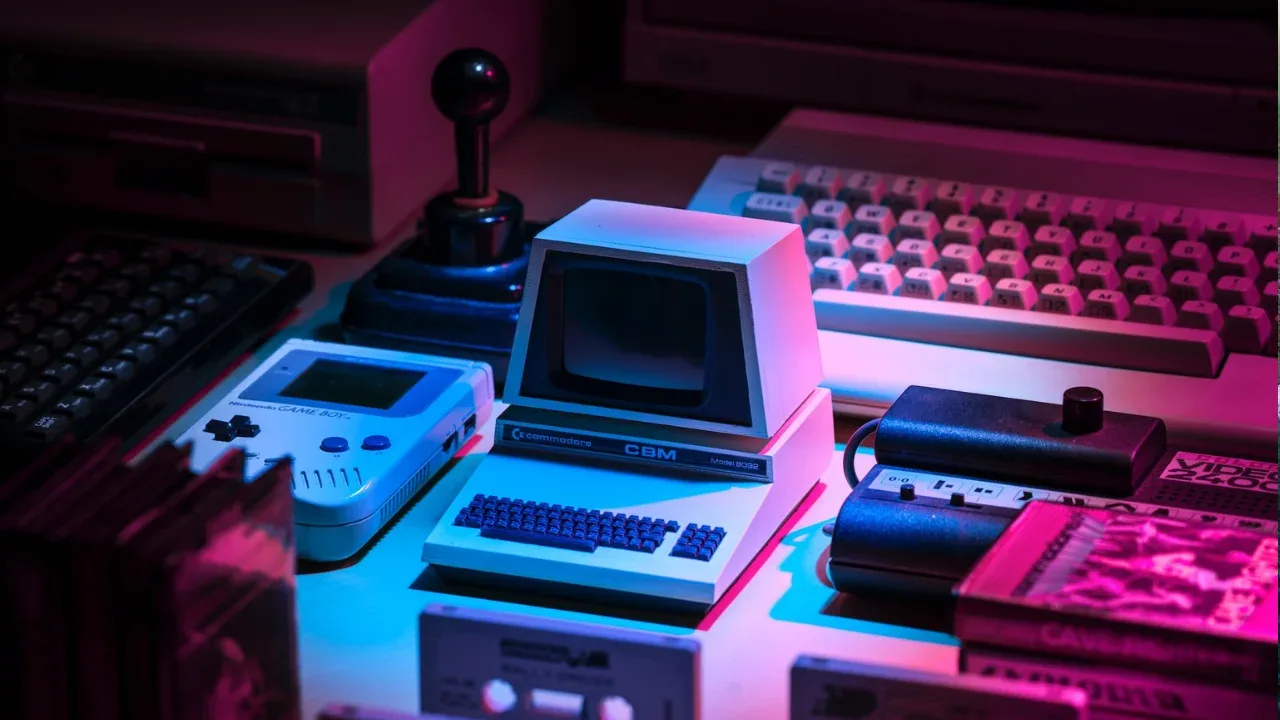
How to Reload or Re-render the Entire Page using AngularJS
Is your AngularJS application in need of a way to reload or re-render the entire page? Whether you have made multiple $http
requests or need to switch contexts, this blog post will provide you with easy solutions to common issues. You won't have to cancel any current requests or manually issue them again. Let's dive in! 💪
The Problem
The scenario is simple: you have rendered the entire page based on various user contexts and multiple $http
requests. Now, you want to give your users the ability to switch contexts and have everything re-rendered, including the resending of all $http
requests.
You might have approached this by using $window.location.reload()
, just to find out that it cancels any ongoing $http
requests. Not ideal, right? Another hack you may have encountered is $location.path($location.path())
, but that doesn't work either. 😞
The Solution
Thankfully, AngularJS provides a proper solution to re-rendering the entire page without the need for manual request issuing. All you need to do is utilize $route.reload()
. Let's see how it works. 🚀
In your controller, add the $route
dependency:
app.controller('YourController', ['$route', function($route) {
// Your code...
}]);
Now, whenever you want to trigger a full page reload, simply call $route.reload()
:
$scope.on_impersonate_success = function(response) {
// Your code...
$route.reload(); // Reloads the entire page using AngularJS
};
$scope.impersonate = function(username) {
return auth.impersonate(username)
.then($scope.on_impersonate_success, $scope.on_auth_failed);
};
By using $route.reload()
, you will seamlessly re-render the page without cancelling any pending $http
requests. It's the perfect solution! 👌
Recap
To recap, here's a step-by-step guide to reload or re-render your whole page using AngularJS:
Inject the
$route
dependency in your controller.
app.controller('YourController', ['$route', function($route) {
// Your code...
}]);
Trigger the reload using
$route.reload()
at the desired point in your code.
$scope.on_impersonate_success = function(response) {
// Your code...
$route.reload(); // Reloads the entire page using AngularJS
};
And that's it! Enjoy the power of seamless page reloading with AngularJS. 🎉
Take Action
Now that you have the knowledge to reload or re-render the entire page using AngularJS, it's time to put it into action. Go ahead and implement this solution in your application, and let us know how it worked for you! Have any questions or other AngularJS topics you'd like us to cover? Leave a comment below! 💬
#HappyCoding 🖥️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
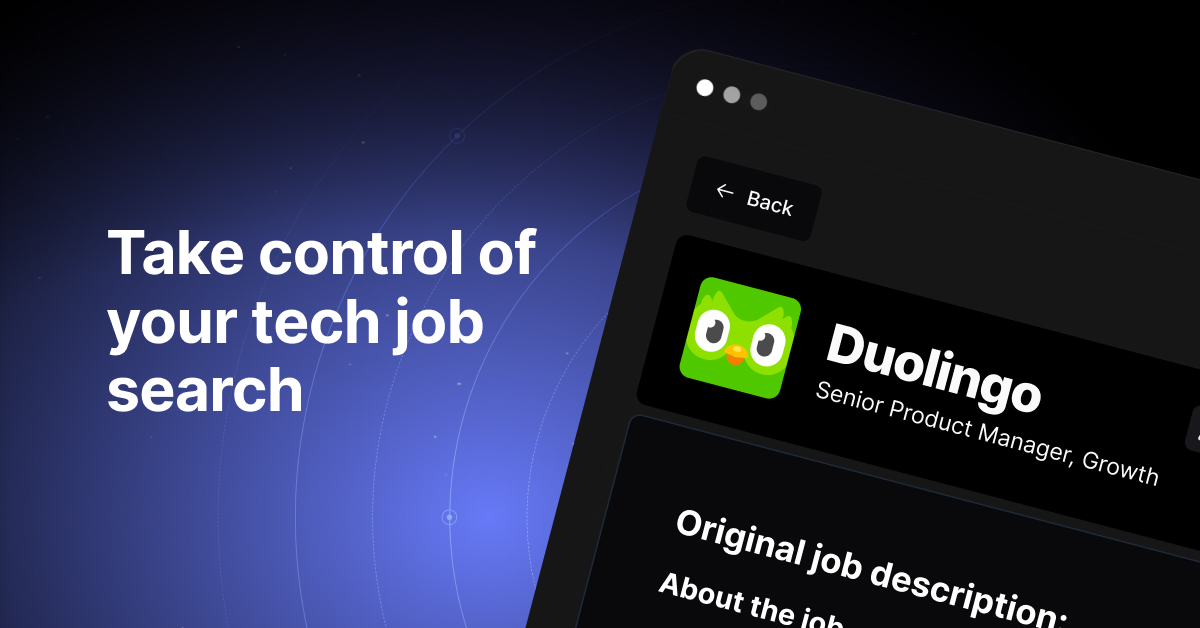