How to read an external local JSON file in JavaScript?
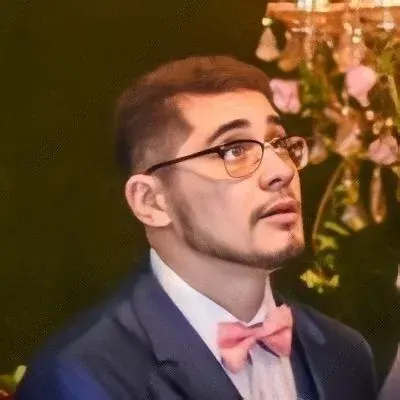
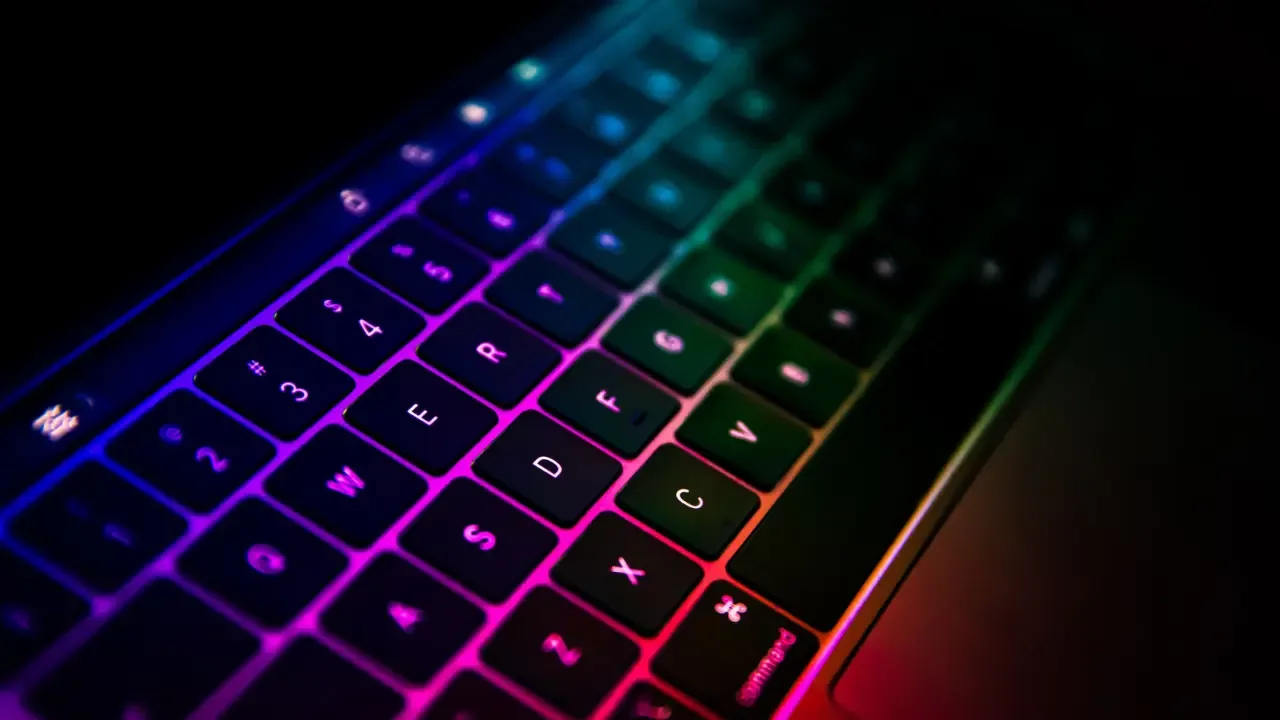
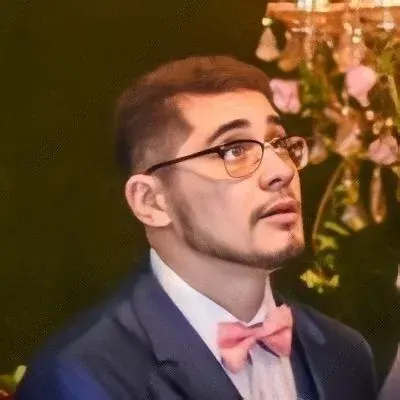
How to Read an External Local JSON File in JavaScript? 📝
So you have a JSON file saved on your local system and you want to read it using JavaScript? No worries, we've got you covered! 😄
First, let's take a look at the JSON file you have:
{
"resource": "A",
"literals": ["B", "C", "D"]
}
Assuming the path of your JSON file is /Users/Documents/workspace/test.json
, let's dive into the steps involved in reading the file and printing its data using JavaScript.
Step 1: Create an XMLHTTPRequest Object 🖥️
To read a local JSON file, we need to create an instance of the XMLHttpRequest
object. This object helps us make HTTP requests to fetch the content of the JSON file.
const xhr = new XMLHttpRequest();
Step 2: Open the JSON File 📂
Next, we need to open the JSON file using the open()
method of the XMLHttpRequest
object. In this method, we pass the HTTP method (in our case, 'GET') and the path to the JSON file.
xhr.open('GET', '/Users/Documents/workspace/test.json', true);
Step 3: Set the Response Type 📭
Before sending the request, we need to specify the response type we expect from the server. In our case, we want the response to be treated as JSON.
xhr.responseType = 'json';
Step 4: Send the Request 🚀
Now, we are ready to send the request to the server using the send()
method. This will asynchronously fetch the JSON file from your local system.
xhr.send();
Step 5: Handle the Response ✉️
To handle the response, we need to listen for the load
event on our XMLHttpRequest
object. The load
event is fired when the file has been successfully loaded.
xhr.onload = function () {
if (xhr.status === 200) {
const jsonData = xhr.response;
// Use jsonData for further processing
console.log(jsonData);
}
};
Congrats! 🎉 You have successfully read the local JSON file using JavaScript! The data from the file is now stored in the jsonData
variable and can be accessed for further processing.
Feel free to modify the code snippet according to your requirements. Now it's your turn to try it out and see the magic happen! ✨
If you have any questions or face any issues, don't hesitate to reach out in the comments below. Happy coding! 💻
Pssst! Want more awesome tech tips and tricks? Don't forget to subscribe to our newsletter for regular updates and exciting content. Stay ahead in the tech game! 📩