How to randomize (shuffle) a JavaScript array?
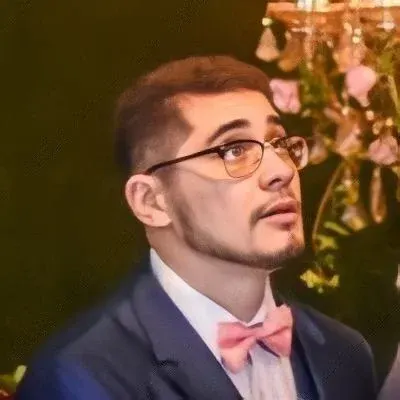
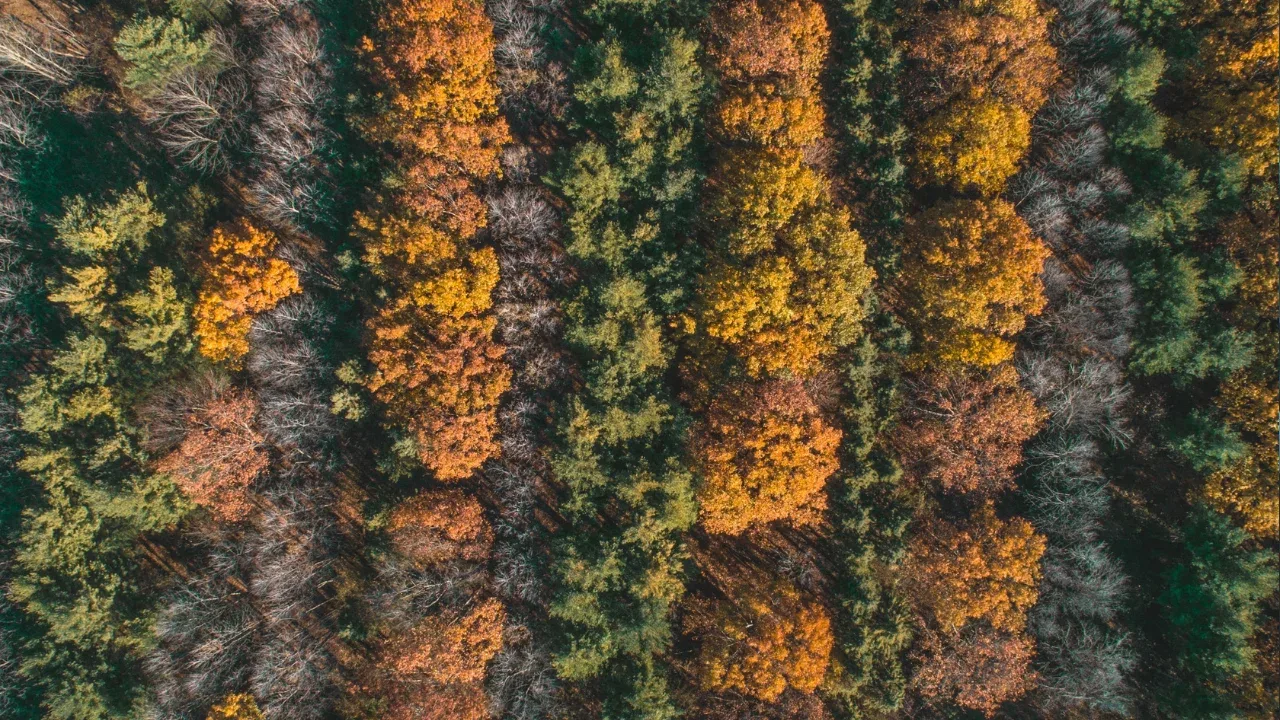
How to Randomize (Shuffle) a JavaScript Array? ๐ฒ
Oh, the joy of randomness โ it keeps life interesting! ๐ If you have ever wanted to shuffle an array in JavaScript, you have come to the right place. Whether you need to randomize a deck of cards, jazz up a list of options, or just inject some unpredictability into your code, shuffling an array is the answer. Let's dive right in and explore some easy solutions to this common problem, shall we? ๐ช
Understanding the Challenge
So, you've got an array that needs some shaking up. Here's the array in question:
var arr1 = ["a", "b", "c", "d"];
Your task is to randomize the order of the elements within arr1
, effectively shuffling it. This means that after shuffling, elements like "a"
, "b"
, "c"
, and "d"
could be in any order โ like a surprise party for your array! ๐
Solution 1: The Fisher-Yates Algorithm
One popular and efficient algorithm for shuffling an array is the Fisher-Yates algorithm. It's like a DJ remixing your playlist โ pure magic! ๐งโจ To utilize this algorithm, follow these easy steps:
// Step 1: Create a function to shuffle the array
function shuffleArray(arr) {
for (let i = arr.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[arr[i], arr[j]] = [arr[j], arr[i]];
}
}
// Step 2: Call the function and pass your array as an argument
shuffleArray(arr1);
Voilร ! Your arr1
array is now shuffled, and the elements are dancing like nobody's watching. ๐๐ถ
Solution 2: The Sort and Randomize Technique
If you prefer a simpler approach without getting into the nitty-gritty of algorithms, we have got you covered. Just breathe out, and let JavaScript's built-in sort()
method do the shuffling for you. Here's how:
// Step 1: Create a function to shuffle the array
function shuffleArray(arr) {
return arr.sort(() => Math.random() - 0.5);
}
// Step 2: Call the function and pass your array as an argument
shuffleArray(arr1);
Magic, right? ๐ชโจ By utilizing the sort()
method with a random sorting function, your array will be shuffled in no time. Much easier than trying to juggle with the elements yourself!
The Big Finale and a Call to Action
Now that you know two amazing techniques to shuffle a JavaScript array, isn't life a little more exciting? ๐๐ You can spread this newfound knowledge to your fellow coders or even show off your newly shuffled arrays. Share this blog post with them, and let them experience the rush of randomness! ๐
Remember, the world thrives on the unexpected. Shuffling arrays is just a small taste of what JavaScript can do. So, experiment, embrace the randomness, and keep coding like a rockstar! ๐ค๐ป
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
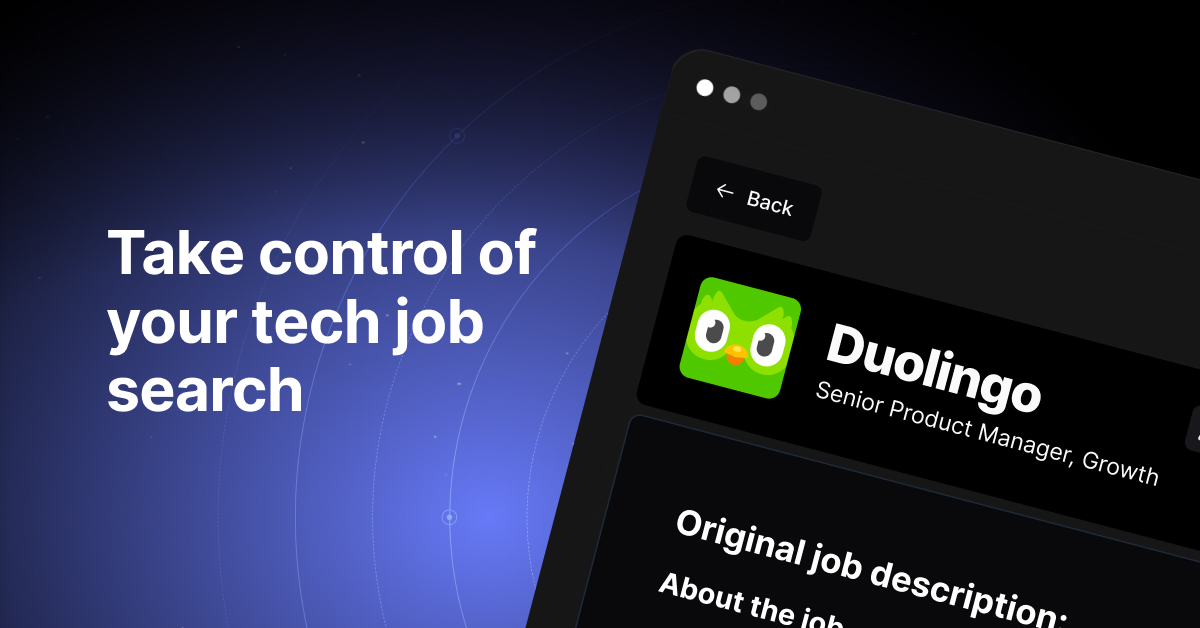