How to print a stack trace in Node.js?
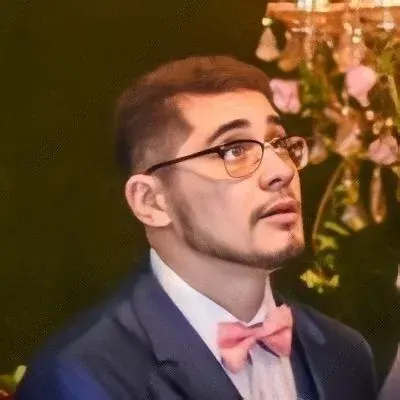
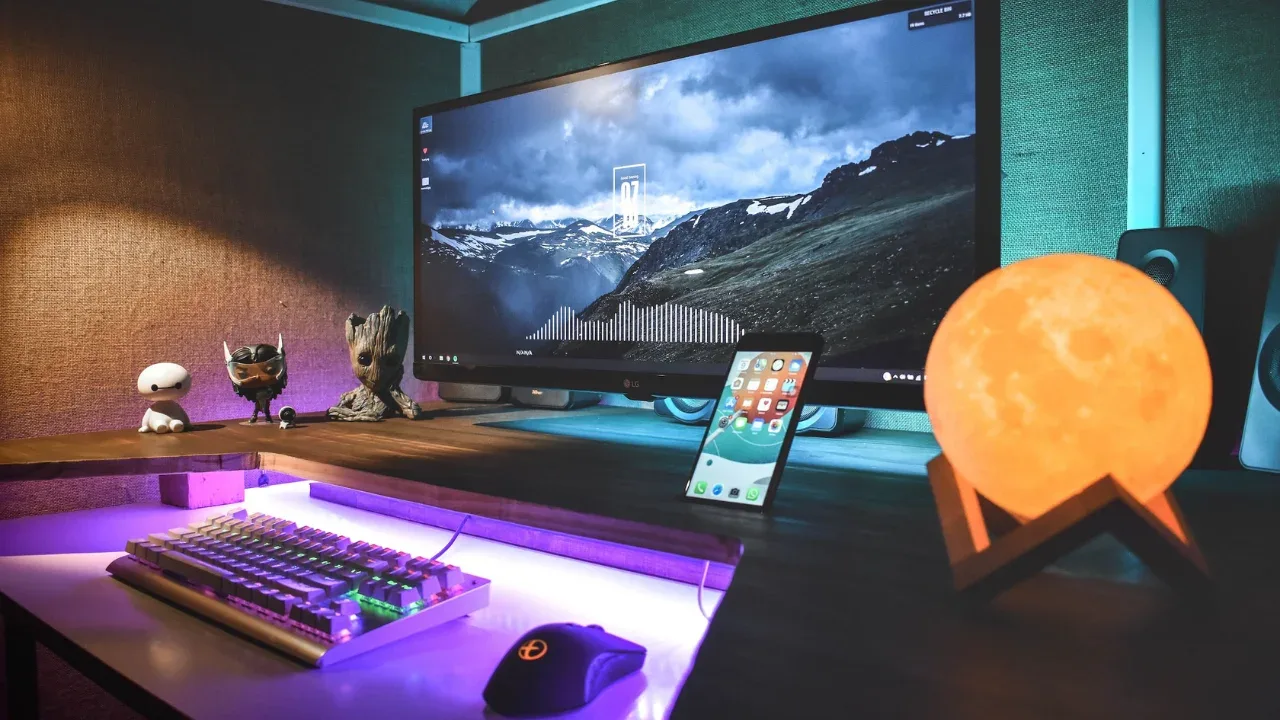
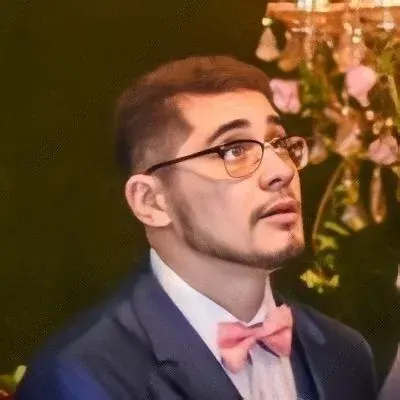
How to Print a Stack Trace in Node.js? 📜🔍
So, you've found yourself in a situation where you need to print a stack trace in Node.js. Don't worry, we've got you covered! 😉 In this article, we'll explore the common issues faced when trying to print a stack trace in Node.js and provide you with easy solutions to overcome them. Let's dive right in! 🚀
Understanding the Stack Trace 🧩
Before we jump into solving the problem, let's make sure we understand the concept of a stack trace. In simple terms, a stack trace is a detailed report of the function calls that are currently on the stack at a particular point during the execution of a program. It helps developers identify the flow of execution and find errors within their code. 🕵️♀️
Common Issues and Easy Solutions 🛠️
1. Error Objects and the stack
Property 🚨
One of the easiest ways to print a stack trace in Node.js is by utilizing the built-in Error
object. Whenever an error occurs in your Node.js application, it will create an Error
object containing valuable information, including the stack trace. To access the stack trace, simply access the stack
property of the Error
object. Here's an example:
try {
// your code here
} catch (error) {
console.error(error.stack);
}
By logging error.stack
, you can print the entire stack trace to your console. This is a quick and reliable method, especially when dealing with synchronous code.
2. Asynchronous Code and Error Events ⌛
When working with asynchronous code, such as callbacks or promises, printing a stack trace can be a bit trickier. However, Node.js provides a helpful callback function that allows you to capture the stack trace within error events. Here's an example:
process.on('uncaughtException', (error) => {
console.error(error.stack);
});
By registering an event listener for the 'uncaughtException'
event, you can print the stack trace whenever an uncaught exception occurs within your Node.js application. Remember to use this approach with caution and only as a last resort, as it can lead to unpredictable state and should not be used for handling expected exceptions.
Take It to the Next Level! 🚀✨
Congratulations! You now have the tools to print a stack trace in Node.js. But why stop there? 🤔 Take your debugging skills to the next level by exploring more advanced tools and techniques, such as:
Using a debugger like the built-in Node.js debugger or popular third-party tools like
ndb
.Leveraging logging libraries like
winston
,pino
, ordebug
for more extensive error logging and handling.
By continually improving your debugging skills, you'll become a more efficient and effective developer. 🌟
Wrapping Up 🎉
In this article, we demystified the process of printing a stack trace in Node.js. We explored common issues, provided easy solutions, and even offered some ways to level up your debugging game. Now it's your turn to put this knowledge into practice and become a debugging wizard! 💪
We hope you found this article helpful! If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 😄👩💻👨💻