How to post a file from a form with Axios
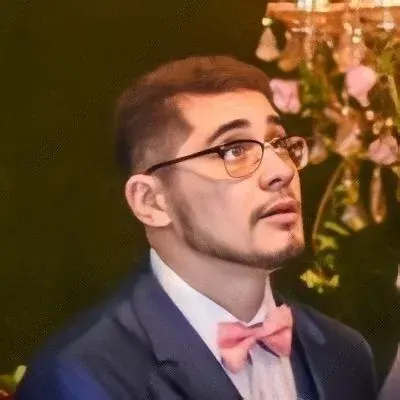
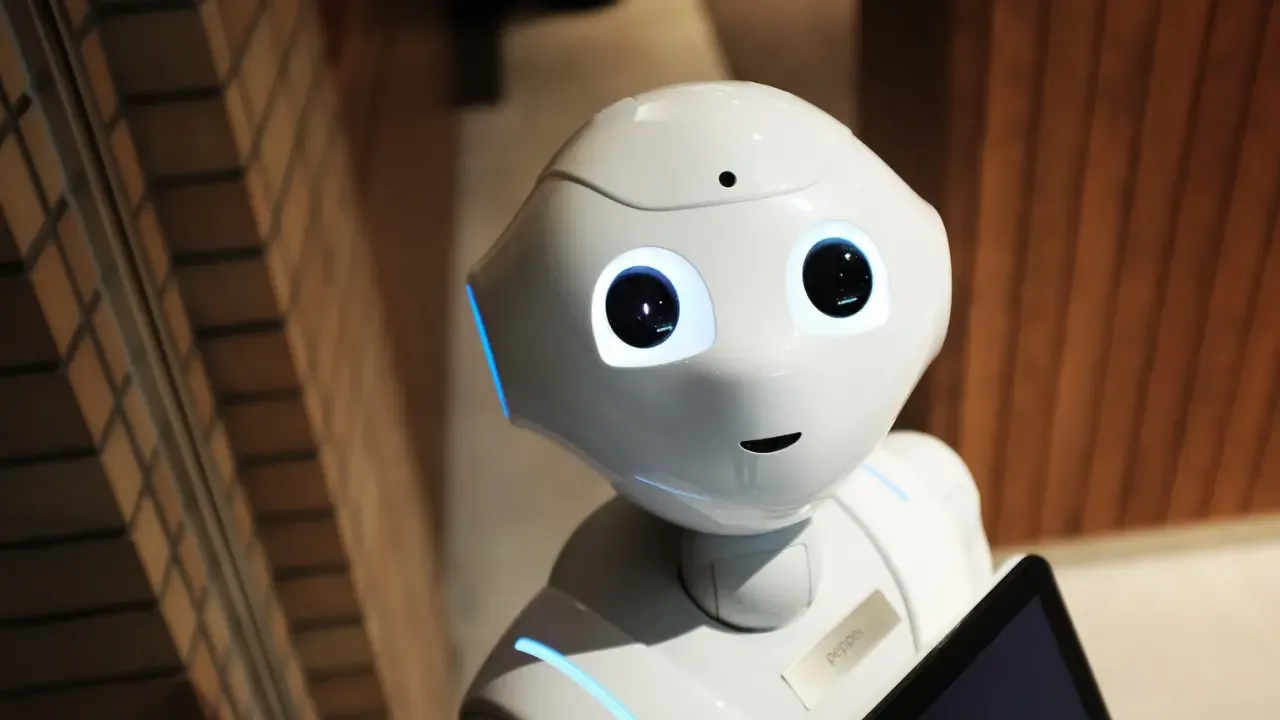
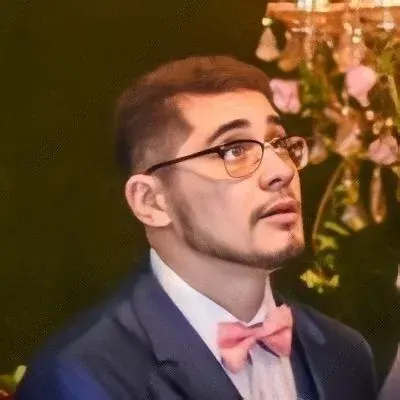
📂 How to Post a File from a Form with Axios
So, you're trying to post a file from a form to a Flask server using Axios, but you're facing some issues. Don't worry, we've got you covered! In this guide, we'll address the common problem of Axios not sending a file object and provide easy solutions to help you overcome this hurdle.
The Issue:
When you try to post a file using Axios, the Flask request global appears to be empty. 😞
The Cause:
The problem lies in the way the file is being sent via Axios. By default, Axios serializes the data as JSON, which is not suitable for sending files. This is why the Flask request global doesn't receive the file object.
The Solution:
To send a file object via Axios, you need to set the Content-Type
header to multipart/form-data
. Let's see how to do that!
First, make sure the form element has a
change
event listener that calls theuploadFile
function. This event will be triggered when a file is selected.<form id="uploadForm" enctype="multipart/form-data" v-on:change="uploadFile"> <input type="file" id="file" name="file"> </form>
In the
uploadFile
function, retrieve the file object from the event object.uploadFile: function (event) { const file = event.target.files[0] // ... }
Now comes the essential part. Use Axios to send the file object to the server. Set the
Content-Type
header tomultipart/form-data
to ensure the file is sent correctly.axios.post('upload_file', file, { headers: { 'Content-Type': 'multipart/form-data' } })
🎉 That's it! Now, the Flask request global should receive the file object correctly.
Example Usage:
Here's a complete example of how the code should look like:
<form id="uploadForm" enctype="multipart/form-data" v-on:change="uploadFile">
<input type="file" id="file" name="file">
</form>
<script>
const app = new Vue({
el: '#app',
data: {},
methods: {
uploadFile: function (event) {
const file = event.target.files[0]
axios.post('upload_file', file, {
headers: {
'Content-Type': 'multipart/form-data'
}
})
.then(response => {
// Handle the response
})
.catch(error => {
// Handle any potential errors
});
}
}
})
</script>
Make sure to replace 'upload_file'
with the appropriate endpoint URL.
📣 Take Action!
Now that you know how to post a file from a form using Axios, go ahead and give it a try! If you still face any issues or have any questions, feel free to ask for help in the comments below. Let's get those files uploaded like a pro! 💪📂✨