How to perform debounce?
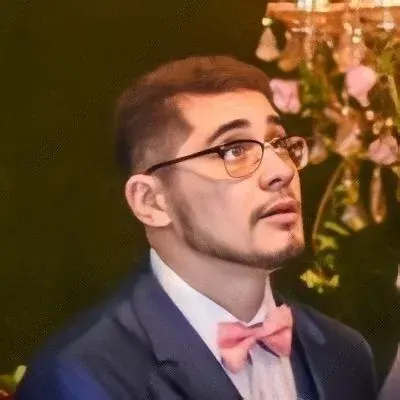
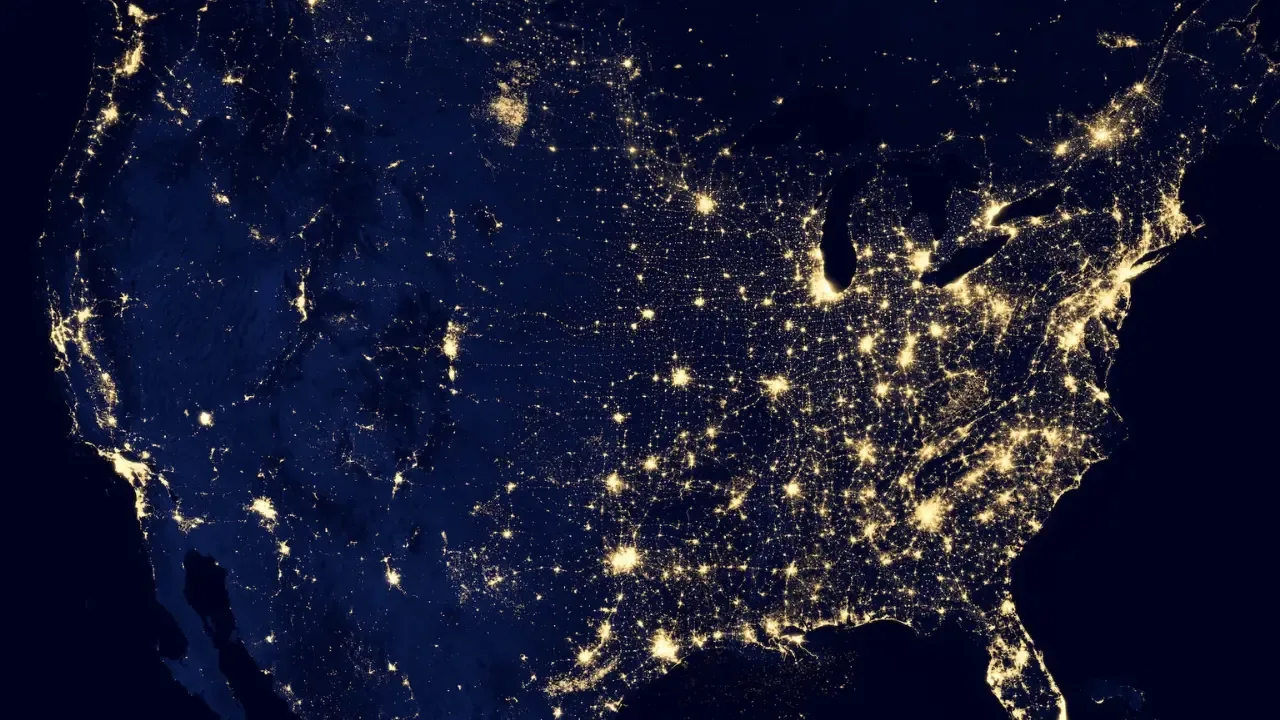
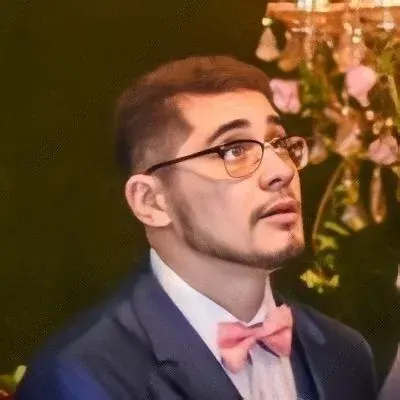
How to Perform Debounce in React.js
Are you wondering how to implement debounce in React.js? 🧐
Debouncing is a technique used to optimize performance by delaying the execution of a particular function until after a specific period of inactivity. This can be especially useful when handling events that may trigger multiple times within a short time interval, such as input changes or scroll events. 🕒
In the context of React.js, you might encounter situations where you want to debounce the onChange
event of an input field, just like in the example provided. Let's dig into some common issues and provide easy solutions to help you successfully perform debounce in React.js. 💡
Issue: Debounce Not Working
You mentioned that you tried using debounce(this.handleOnChange, 200)
but it did not work. What could be causing this? 🤔
The problem lies in the way the debouncing function is being implemented. Let's take a closer look at the code snippet you posted:
function debounce(fn, delay) {
var timer = null;
return function() {
var context = this,
args = arguments;
clearTimeout(timer);
timer = setTimeout(function() {
fn.apply(context, args);
}, delay);
};
}
var SearchBox = React.createClass({
render: function() {
return <input type="search" name="p" onChange={this.handleOnChange} />;
},
handleOnChange: function(event) {
// make ajax call
}
});
The debounce
function you've defined is correct. However, the way it is being used on the onChange
event of the input
element in your SearchBox
component is causing it to fail.
Solution: Binding the Debounced Function
To properly implement debounce, you need to modify your code so that the debounced version of the handleOnChange
function is bound to the onChange
event. Here's how you can do it:
var SearchBox = React.createClass({
componentDidMount: function() {
this.debouncedHandleOnChange = debounce(this.handleOnChange, 200);
},
render: function() {
return <input type="search" name="p" onChange={this.debouncedHandleOnChange} />;
},
handleOnChange: function(event) {
// make ajax call
}
});
By creating a new property called debouncedHandleOnChange
in the componentDidMount
lifecycle method, we bind the debounced version of handleOnChange
to it. Then, we use this new property in the onChange
event of the input
element.
Conclusion
And there you have it! 👏 By following the solution outlined above, you should now be able to successfully debounce the onChange
event in React.js. Whether you are handling input changes, scroll events, or any other events that could trigger multiple times in a short timeframe, debouncing can optimize your application's performance and prevent unnecessary, rapid function calls. 🚀
Feel free to try this solution in your own codebase and let us know how it goes! If you have any further questions or want to share your experience, leave a comment below. We'd love to hear from you. 😊