How to pass props to {this.props.children}
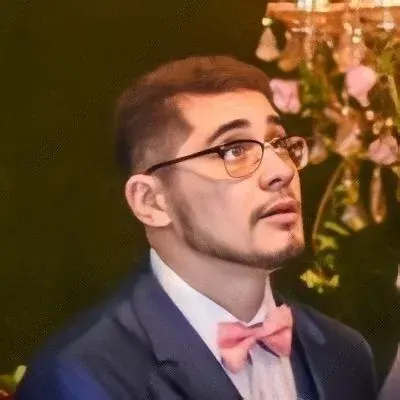
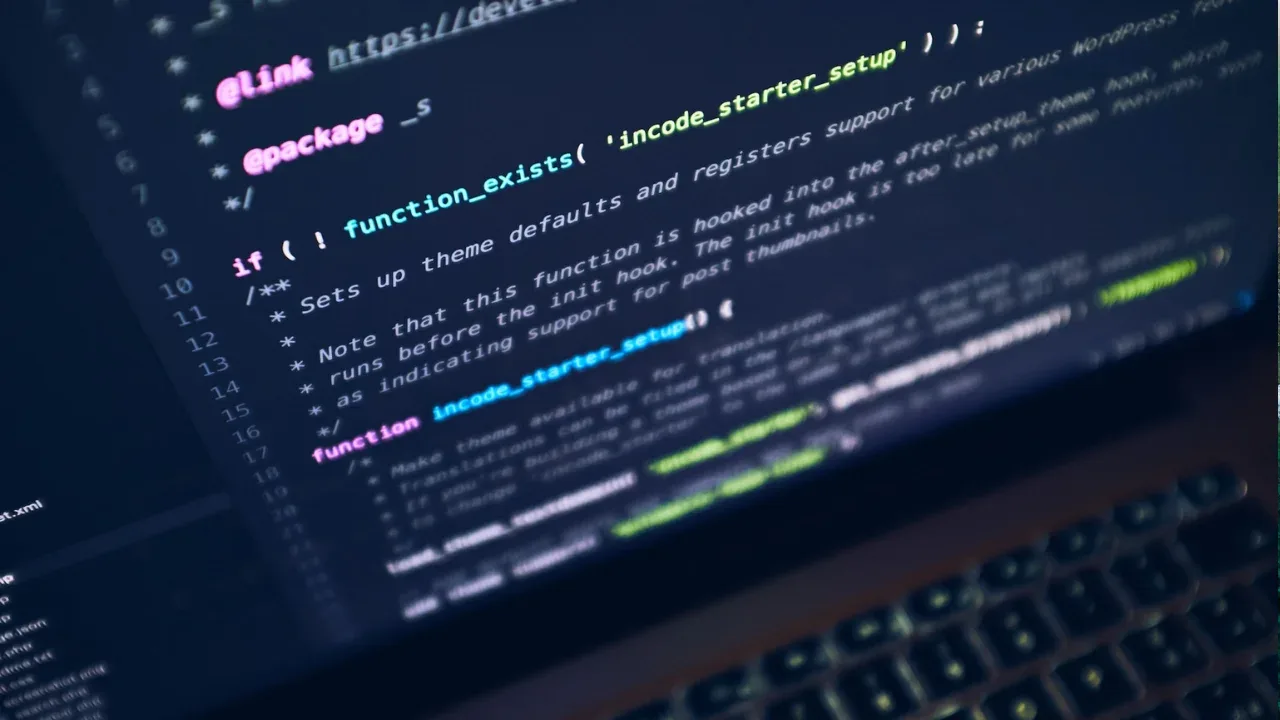
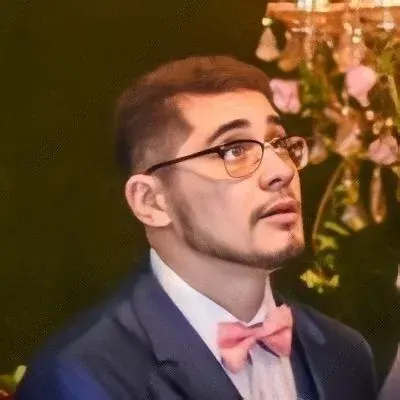
How to pass props to {this.props.children}
So, you want to pass some props to all the children components when using {this.props.children}
in a wrapper component? Gotcha! 😎
The Problem
Let's take a look at the code snippet you provided:
var Parent = React.createClass({
doSomething: function(value) {
},
render: function() {
return (<div>{this.props.children}</div>);
}
});
var Child = React.createClass({
onClick: function() {
this.props.doSomething(this.props.value); // doSomething is undefined
},
render: function() {
return (<div onClick={this.onClick}></div>);
}
});
In this example, the Parent
component is rendering its children components using {this.props.children}
. However, the Child
component needs to call a function doSomething
defined in the Parent
component, which is currently undefined.
The Solution
To pass props to {this.props.children}
, you can utilize React's React.Children
utility. This utility allows you to iterate over the children components, clone them, and pass additional props to them. 🙌
Here's the updated code:
var Parent = React.createClass({
doSomething: function(value) {
// do something with the value
},
render: function() {
var childrenWithProps = React.Children.map(this.props.children, function(child) {
return React.cloneElement(child, { doSomething: this.doSomething });
}, this);
return (<div>{childrenWithProps}</div>);
}
});
var Child = React.createClass({
onClick: function() {
this.props.doSomething(this.props.value);
},
render: function() {
return (<div onClick={this.onClick}></div>);
}
});
In the Parent
component's render
method, we use React.Children.map
to iterate over the children components passed in. By calling React.cloneElement
on each child, we can add the doSomething
prop and pass it the function defined in the Parent
component.
Example
Let's see this in action! 😄
<Parent>
<Child value="1" />
<Child value="2" />
</Parent>
In this example, both Child
components will now have access to the doSomething
prop, which points to the function defined in Parent
. When the onClick
event is triggered in any Child
component, it will call doSomething
with the corresponding value.
Conclusion
Passing props to {this.props.children}
is a common requirement when working with wrapper components. Utilizing React.Children.map
and React.cloneElement
allows you to easily add additional props to all the children components. 😊
So, next time you encounter this situation, remember to use these techniques and make your React components dance to your tune! 💃
Do you have any other cool React tricks up your sleeve? Share them with us in the comments below and let's continue leveling up our React skills together! 🚀