How to pass parameters using ui-sref in ui-router to the controller
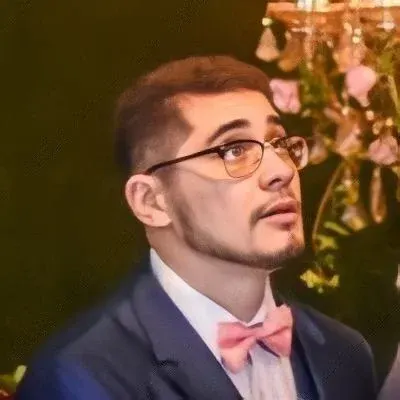
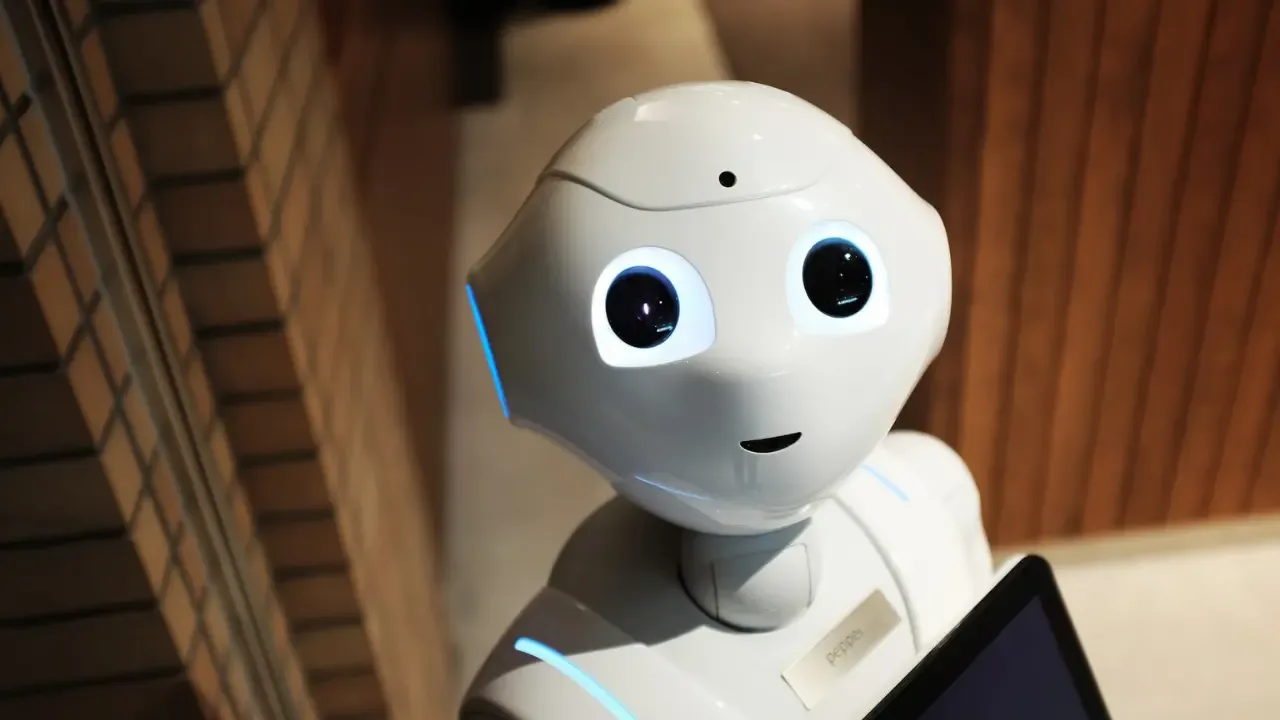
📝 Tech Blog: Passing Parameters using ui-sref in ui-router to the Controller
Hey there, tech enthusiasts! 👋 Today we are here to tackle a common question that often leaves developers scratching their heads: "How can I pass parameters using ui-sref
in ui-router
to the controller?" We'll dig deep into this issue, provide easy solutions, and empower you to navigate this challenge with ease. Let's get started! 💪
To set the stage, imagine you have a link that needs to transition from one state to another while passing some parameters to the destination state. Here's an example that demonstrates how you can achieve this using the ui-sref
attribute:
<a ui-sref="home({foo: 'fooVal', bar: 'barVal'})">Go to home state with foo and bar parameters</a>
In the above code snippet, we're specifying the state to transition to as "home" and passing the foo
and bar
parameters with values "fooVal" and "barVal", respectively.
Now, let's talk about how to receive these parameters in your controller. In AngularJS, you can access them via the $stateParams
service. However, in your provided code, you encountered an issue of getting undefined
for $stateParam
in the controller.
The reason behind this error is most likely due to a typo. The correct service name is $stateParams
, plural, not $stateParam
. 🙃
Here's an updated code snippet of your controller, with the corrected service name:
app.controller('SomeController', function($scope, $stateParams) {
// ..
var foo = $stateParams.foo; // Now you'll get the value "fooVal"
var bar = $stateParams.bar; // Now you'll get the value "barVal"
// ..
});
Now you're all set! By accessing $stateParams.foo
and $stateParams.bar
in your controller, you'll be able to retrieve the values passed as parameters using ui-sref
.
In case you're wondering, your ui-router
configuration hasn't been provided, but to give you an idea, here's a sample snippet:
.state('home', {
url: '/',
views: {
'': {
templateUrl: 'home.html',
controller: 'MainRootCtrl'
},
'A@home': {
templateUrl: 'a.html',
controller: 'MainCtrl'
},
'B@home': {
templateUrl: 'b.html',
controller: 'SomeController'
}
}
});
Feel free to adapt this to fit your project structure! 😉
Before we wrap up, we'd love for you to engage with our tech community and share your thoughts! Have you ever faced a similar issue while passing parameters in ui-router
? Did you find this guide helpful? Drop a comment below and let's learn from each other! 💬
That's a wrap, folks! We hope this guide has demystified the process of passing parameters using ui-sref
in ui-router
, making it a breeze for you to implement in your projects. Keep coding and stay curious! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
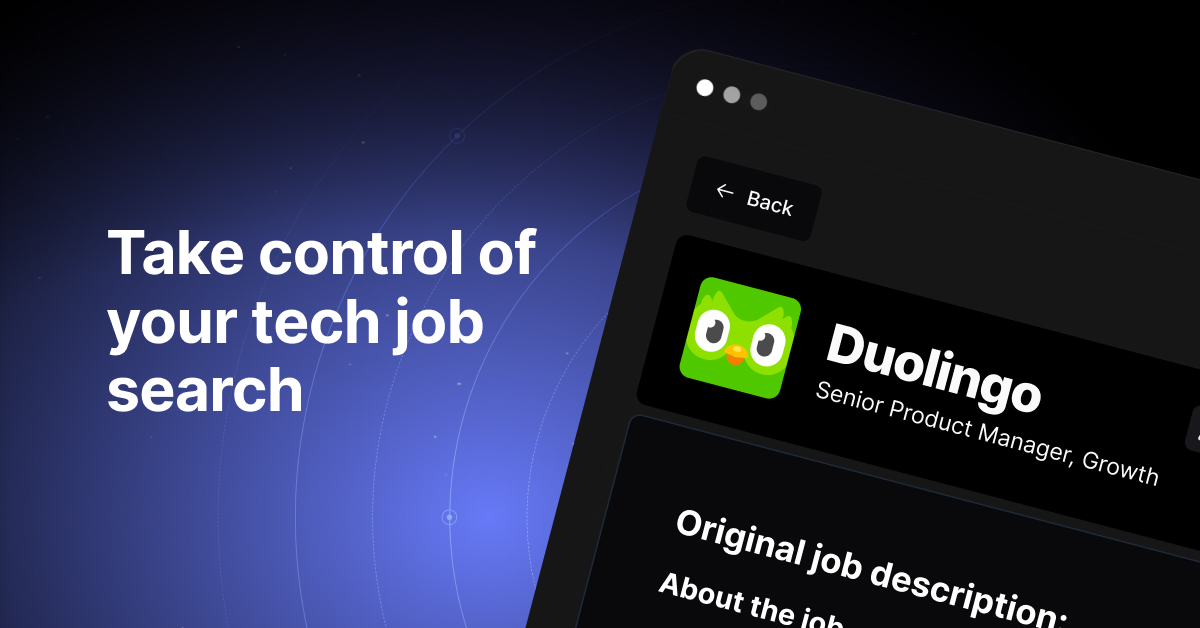