How to pass parameters in GET requests with jQuery
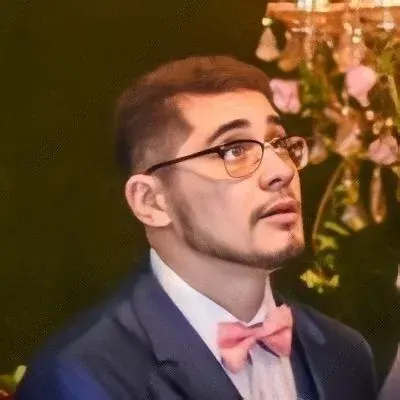
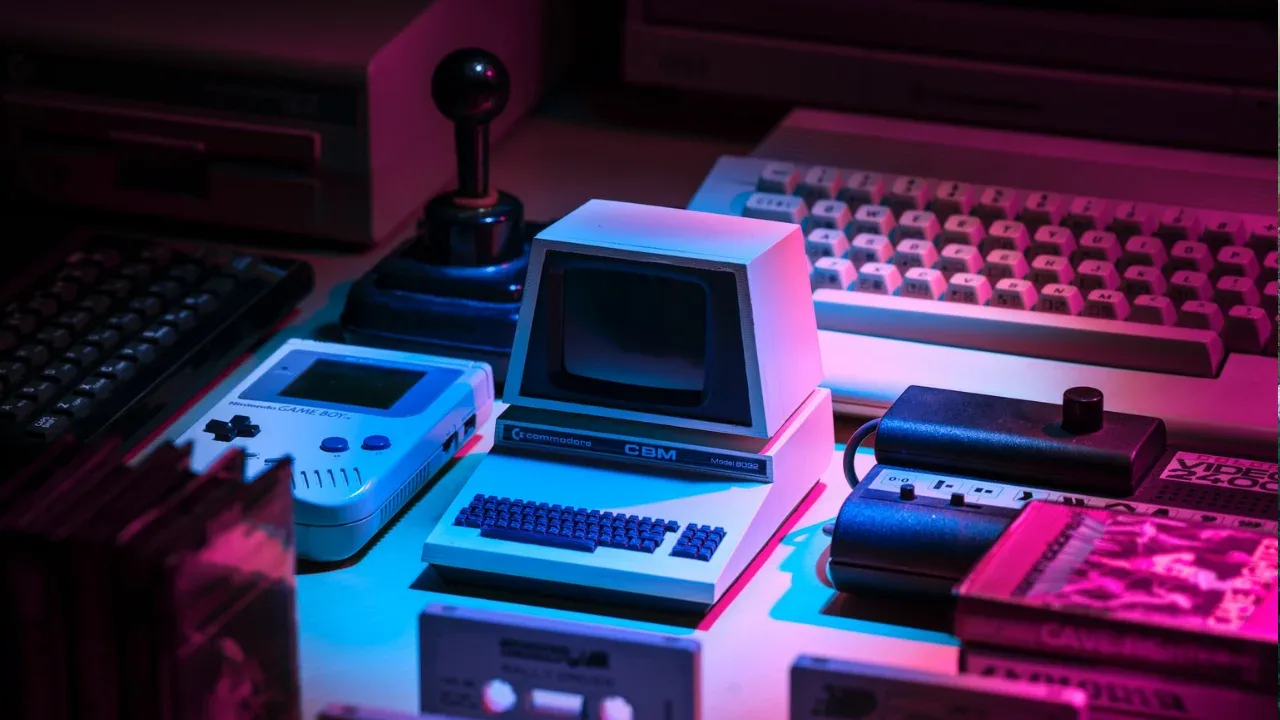
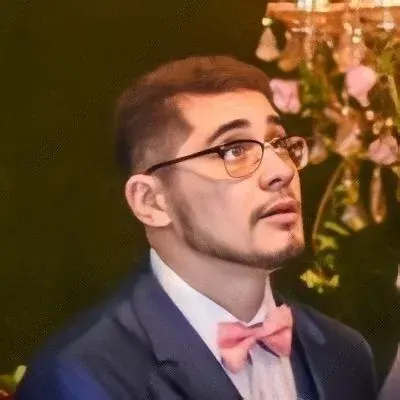
How to Pass Parameters in GET Requests with jQuery 😃
Have you ever struggled with passing query string values in a jQuery Ajax request? 🤔 It can be cumbersome, especially when you want to avoid manually encoding the parameters. But worry not! In this article, we will explore a cleaner and more efficient way to handle this common issue. Let's dive in! 💪
The Old Way: Manual Encoding 😩
Previously, you might have come across a solution like this:
$.ajax({
url: "ajax.aspx?ajaxid=4&UserID=" + UserID + "&EmailAddress=" + encodeURIComponent(EmailAddress),
success: function(response) {
// Do Something
},
error: function(xhr) {
// Handle Error
}
});
While this approach works, it requires manual encoding of each parameter using the encodeURIComponent()
function. This can quickly become tedious and error-prone. 🙅♀️
A Simpler Alternative: The $.get() Method 😍
If you prefer a cleaner and more concise solution, consider using the $.get()
method instead. It allows you to pass query string parameters as an object, eliminating the need for manual encoding. Check it out:
$.get("ajax.aspx", { UserID: UserID , EmailAddress: EmailAddress });
By passing the parameters as an object, the $.get()
method takes care of the encoding automatically. This makes your code more readable and less prone to mistakes. 🎉
Stick with Personal Preference: The $.ajax() Method 😎
But what if you prefer using the $.ajax()
method? No worries, you can still achieve the simplicity of the $.get()
method while sticking to your personal preference. Here's how:
$.ajax({
url: "ajax.aspx?ajaxid=4",
data: {
"VarA": VarA,
"VarB": VarB,
"VarC": VarC
},
cache: false,
type: "POST",
success: function(response) {
// Handle Success
},
error: function(xhr) {
// Handle Error
}
});
By specifying the data
property as an object, the $.ajax()
method automatically encodes the parameters for you. This approach not only simplifies your code but also enhances its readability. 🤩
Closing Thoughts 🌟
Passing parameters in GET requests with jQuery doesn't have to be a headache. With the $.get()
method or the simplified approach using the $.ajax()
method, you can effortlessly handle this task without worrying about manual encoding. 💪
So, the next time you find yourself struggling with passing parameters, remember these easy solutions. Give them a try and see how they make your code cleaner and more maintainable. Happy coding! 😄
If you found this article helpful, feel free to share it with your friends and colleagues. And don't forget to leave a comment below sharing your thoughts and experiences. Let's make passing parameters in GET requests a breeze for everyone! 🚀