How to parse JSON using Node.js?
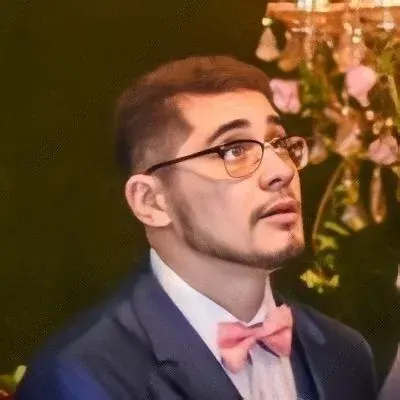
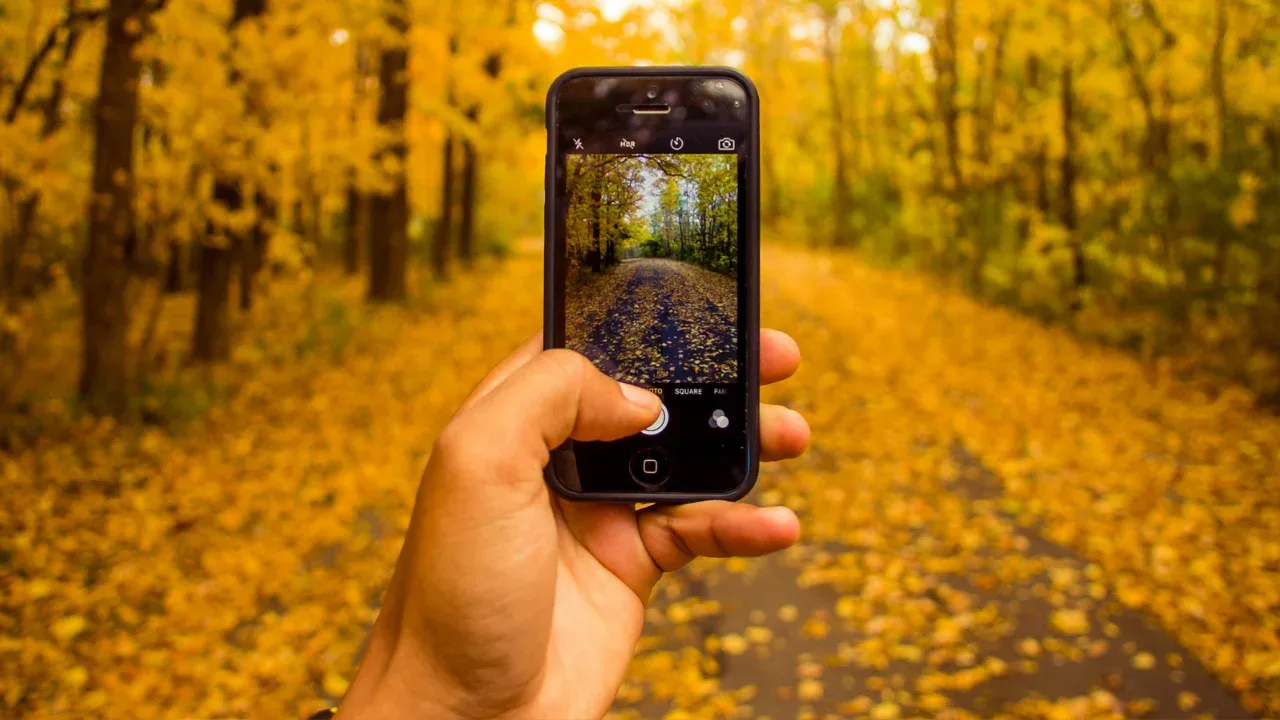
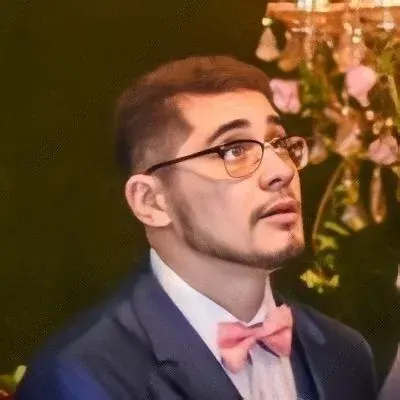
š” Parsing JSON Using Node.js: A Secure and Easy Guide š”
š Hey there, tech enthusiasts! Are you wondering how to parse JSON using Node.js? Do you want to ensure that your JSON is validated and parsed securely? Look no further because we've got you covered! In this guide, we'll provide you with step-by-step instructions on how to parse JSON in Node.js securely. Let's dive right in! š
š Understanding the Basics of JSON Parsing
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is widely used to transmit data between a server and a web application. Parsing JSON in Node.js allows you to extract and manipulate data with ease.
š To get started with JSON parsing, you'll need to have Node.js installed on your machine. If you haven't already done that, head over to the official Node.js website and follow the instructions.
š Securing Your JSON Parsing Process
When parsing JSON, it is crucial to ensure that the input data is validated and sanitized to prevent security vulnerabilities such as code injection or denial-of-service attacks. Fortunately, Node.js provides a built-in module called JSON.parse()
that handles the parsing process and ensures security by default. Therefore, you can trust Node.js to handle most security concerns for you.
š Parsing JSON with Node.js: Step-by-Step Guide
Now, let's walk through the step-by-step process of parsing JSON using Node.js:
1. Importing Required Modules
const fs = require('fs');
First, we need to import the necessary modules. In this case, we'll be using the fs
module to read the JSON file.
2. Reading the JSON File
const rawData = fs.readFileSync('data.json');
Next, we read the JSON file using readFileSync()
method from the fs
module. Make sure to replace 'data.json'
with the path to your JSON file.
3. Parsing JSON
const jsonData = JSON.parse(rawData);
Now, we can parse the JSON data using JSON.parse()
method and assign it to a variable called jsonData
.
4. Accessing Parsed Data
console.log(jsonData);
Finally, we can access and manipulate the parsed JSON data. In this example, we simply log the jsonData
variable to the console. You can perform various operations on the data based on your requirements.
š And that's it! You have successfully parsed JSON using Node.js. š
š¦ Common Issues and Troubleshooting
š« Invalid JSON: If the JSON file you're trying to parse is invalid, Node.js will throw an error. Make sure your JSON is properly formatted and follows the JSON syntax rules. You can use online JSON validators to verify the validity of your JSON.
š« File Not Found: If the readFileSync()
method throws a FileNotFoundError
, double-check the file path and ensure that the JSON file exists in the specified location.
šÆ Join the JSON Parsing Adventure
Now that you have learned how to parse JSON using Node.js, it's time to put your skills to the test! š
š Share your JSON parsing journey with us in the comments below! What challenges did you face, and how did you overcome them? Let's start a conversation and help each other grow as Node.js developers. šŖ
š Stay tuned for more exciting and informative tech articles by following our blog. Don't forget to subscribe to our newsletter for regular updates and exclusive content. Happy JSON parsing! šāØ