How to parse JSON string in Typescript
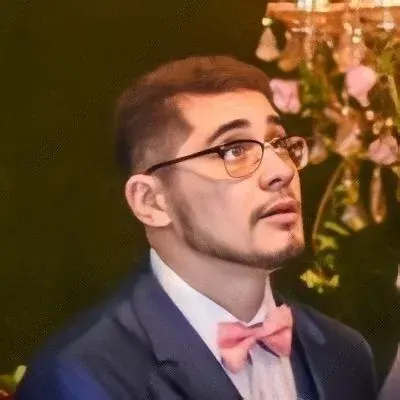
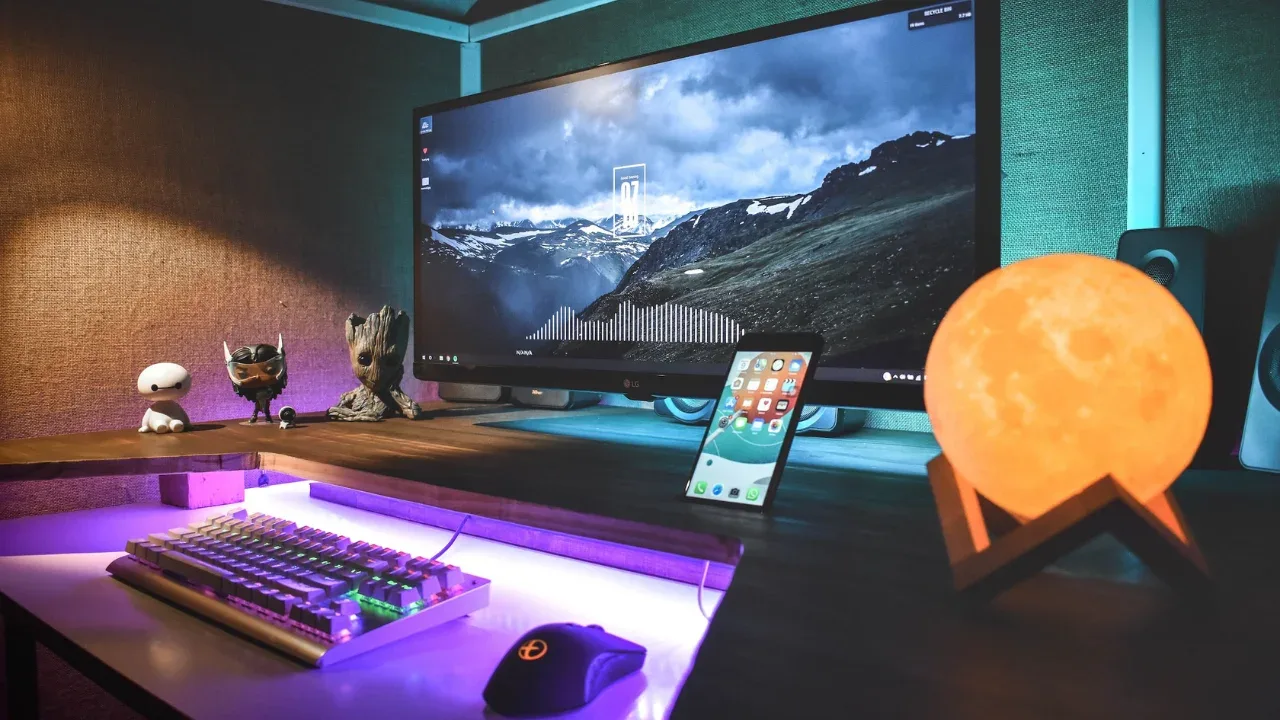
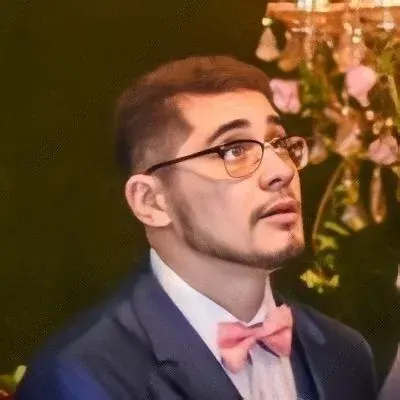
📚 How to Parse JSON String in TypeScript
Are you a TypeScript developer looking for a way to parse strings as JSON? Don't worry, we've got you covered! In this blog post, we will explore how to parse a JSON string in TypeScript and provide easy solutions to common issues you may encounter along the way. Let's get started! 🚀
Understanding JSON Parsing in TypeScript
To begin, let's have a quick refresher on JSON parsing in TypeScript. JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy to read and write. In TypeScript, JSON parsing allows us to convert a JSON string into a TypeScript object, making it easier to work with and manipulate the data.
Using JSON.parse() in TypeScript
In JavaScript, we can use the JSON.parse()
function to parse JSON strings. However, TypeScript does not have a built-in JSON parsing function. Instead, we can leverage the JSON.parse()
function available in the browser's JavaScript runtime.
Here's an example of how we can parse the JSON string you provided in your question:
const jsonString = `{"name": "Bob", "error": false}`;
const parsedObject = JSON.parse(jsonString);
console.log(parsedObject); // Output: { name: 'Bob', error: false }
As you can see, we use the JSON.parse()
function and pass the JSON string as a parameter. The function returns a TypeScript object that represents the parsed JSON string. We can then work with the parsed object just like any other TypeScript object.
Handling Common Issues
While parsing JSON strings in TypeScript is relatively straightforward, there are a couple of common issues you may encounter:
Malformed JSON: If the JSON string is not properly formatted, the
JSON.parse()
function will throw aSyntaxError
. To handle this, you can wrap the parsing logic in a try-catch block and handle the error gracefully:try { const parsedObject = JSON.parse(jsonString); console.log(parsedObject); } catch (error) { console.error('Invalid JSON string:', error); }
JSON with Special Characters: If your JSON string contains special characters that need to be escaped (e.g., double quotes within a string), make sure to use proper escaping techniques to prevent syntax errors:
const jsonString = `{"name": "Bob said \\"Hello!\\", "error": false}`; const parsedObject = JSON.parse(jsonString); console.log(parsedObject);
In this example, we use backslashes (
\
) to escape the double quotes within the string value.
Call-to-Action: Engage with Us!
We hope this guide has helped you understand how to parse a JSON string in TypeScript. If you have any questions, encountered any issues, or have additional tips to share, we'd love to hear from you! Leave a comment below and let's start a conversation. Happy coding! 🎉✨
References: MDN Web Docs - JSON.parse()