How to open a URL in a new Tab using JavaScript or jQuery?
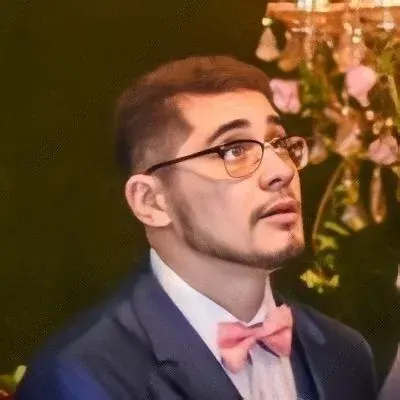
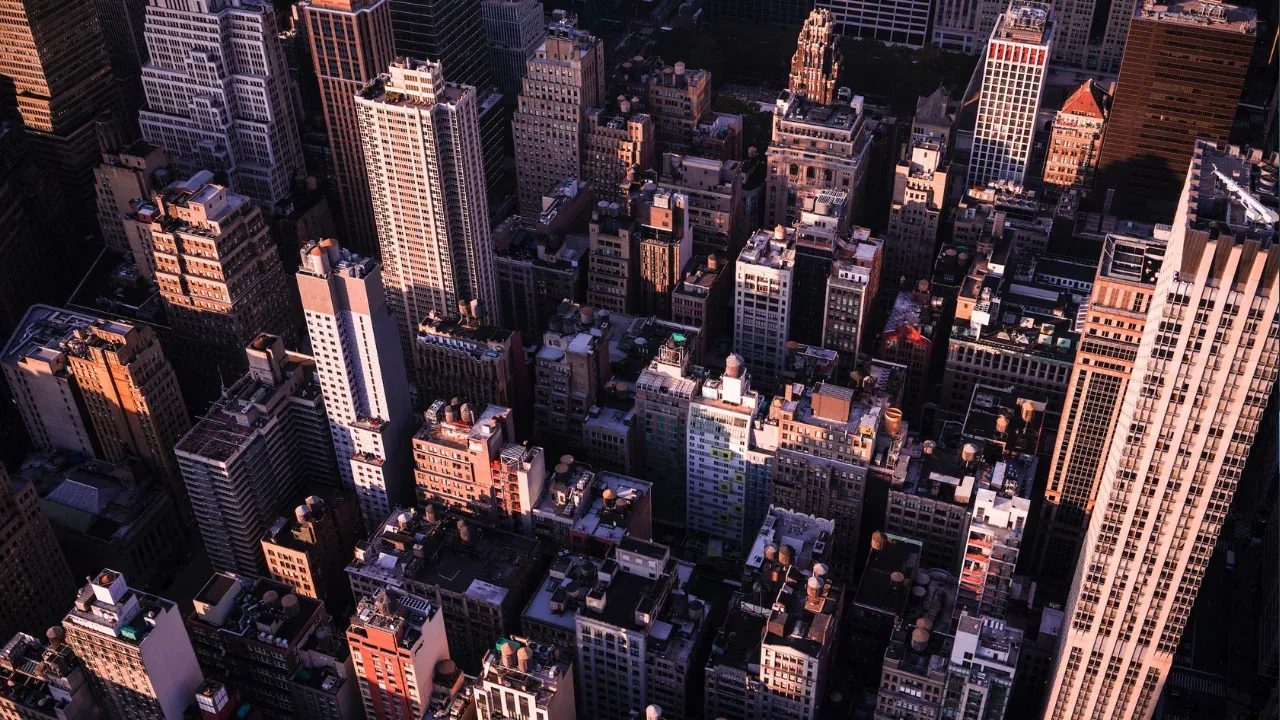
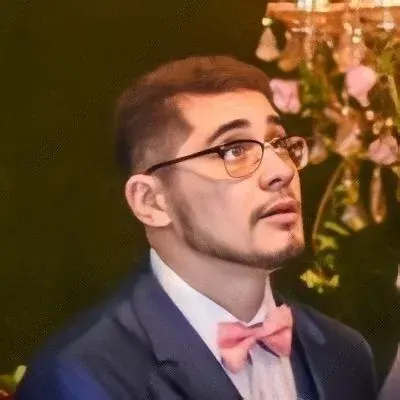
🌟 Open a URL in a New Tab Using JavaScript or jQuery! 🌟
Have you ever wanted to open a URL in a new tab instead of a new window programmatically? You're not alone! Many developers encounter this problem and aren't sure how to solve it.
In this blog post, we'll address this common issue and provide you with easy solutions using JavaScript or jQuery. By the end of this guide, you'll be able to open URLs in new tabs like a pro!
🚩 The Problem: Opening a URL in a New Tab
Imagine you have a web application, and when a user clicks a button or a link, you want to open a specific URL in a new tab instead of a new window. By default, when you use window.open()
in JavaScript or jQuery, it usually opens a new window.
But fear not! We have solutions that will empower you to open URLs in new tabs effortlessly.
🛠️ Solution 1: Vanilla JavaScript
In vanilla JavaScript, you can achieve the desired behavior using the window.open()
method along with the "_blank"
target attribute. Here's how you can do it:
function openURLInNewTab(url) {
window.open(url, "_blank");
}
In this example, the openURLInNewTab()
function takes a url
parameter and opens it in a new tab using window.open()
with "_blank"
as the target.
🛠️ Solution 2: jQuery
If you prefer using jQuery, opening a URL in a new tab becomes just as easy! Here's how you can accomplish it:
function openURLInNewTab(url) {
$('<a href="' + url + '" target="_blank">')[0].click();
}
In this jQuery solution, we create a new <a>
element with a dynamically generated href
attribute and a target
attribute set to "_blank"
. We then simulate a click on this element to open the URL in a new tab.
📢 Take Action: Open URLs with Style!
Now that you have two simple solutions to open URLs in new tabs programmatically, go ahead and give them a try! Experiment with the code snippets we provided and see which one works best for your specific use case.
But don't stop there! Share your success stories or any other solutions you've discovered in the comments below. Together, we can make the web a more user-friendly and efficient place!
So, what are you waiting for? Let's open URLs in new tabs like pros! 🚀
🗒️ Conclusion
Opening URLs in new tabs programmatically can be challenging, but with the right knowledge and these easy solutions using JavaScript or jQuery, you can conquer it. Remember to use the window.open()
method with "_blank"
as the target, and you'll be good to go.
We hope this guide has provided you with the tools you need to tackle this problem effortlessly. Now go ahead and make your web application shine with URLs that open in new tabs!
If you found this blog post helpful, don't forget to share it with your fellow developers or leave us a comment below. We love hearing from you! 😊