How to open a Bootstrap modal window using jQuery?
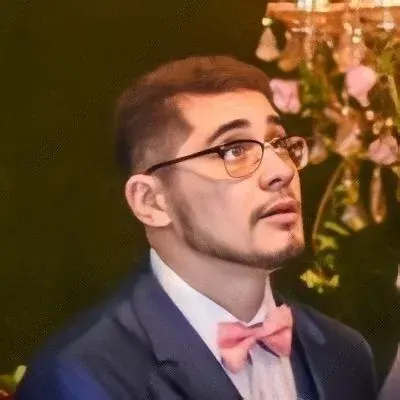
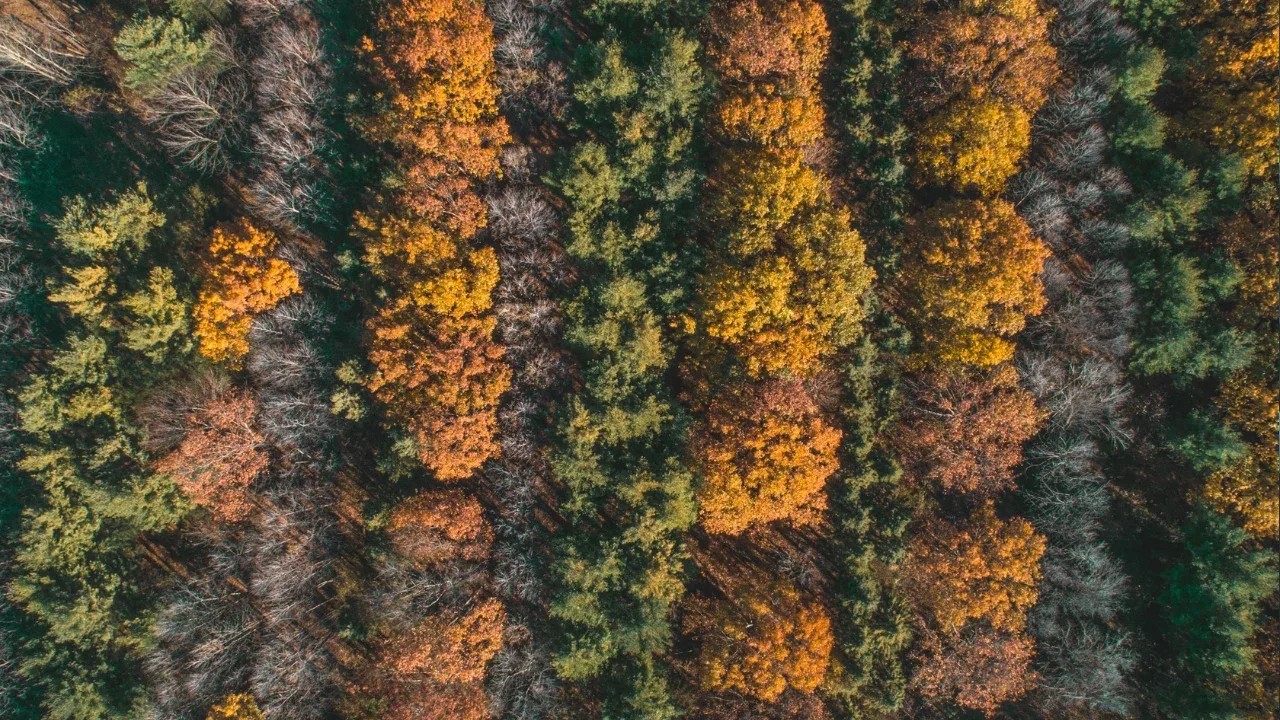
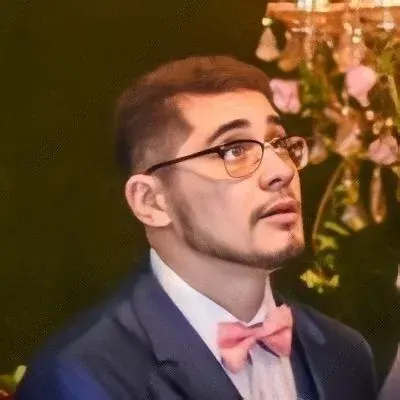
🎉 How to Open a Bootstrap Modal Window Using jQuery 🎉
Are you using Twitter Bootstrap's amazing modal window functionality? Do you want to show the modal window upon clicking the "submit" button in your form? You're in the right place! In this blog post, we'll tackle this common issue and provide easy solutions.
Let's start by taking a look at the code snippet provided:
<form id="myform" class="form-wizard">
<h2 class="form-wizard-heading">BootStap Wizard Form</h2>
<input type="text" value=""/>
<input type="submit"/>
</form>
<!-- Modal -->
<div id="myModal" class="modal hide fade" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h3 id="myModalLabel">Modal header</h3>
</div>
<div class="modal-body">
<p>One fine body…</p>
</div>
<div class="modal-footer">
<button class="btn" data-dismiss="modal" aria-hidden="true">Close</button>
<button class="btn btn-primary">Save changes</button>
</div>
</div>
The jQuery code provided attempts to open the modal window upon form submission. However, it seems there's a mistake in the code. Let's take a closer look:
$('#myform').on('submit', function(ev) {
$('#my-modal').modal({
show: 'false'
});
var data = $(this).serializeObject();
json_data = JSON.stringify(data);
$("#results").text(json_data);
$(".modal-body").text(json_data);
ev.preventDefault();
});
Solution:
To open the Bootstrap modal window correctly, we need to use the correct ID targeting the modal, which is #myModal
, instead of #my-modal
. Additionally, we should set the show
option to true
instead of the string 'false'
. The corrected code snippet should look like this:
$('#myform').on('submit', function(ev) {
$('#myModal').modal({
show: true
});
var data = $(this).serializeObject();
json_data = JSON.stringify(data);
$("#results").text(json_data);
$(".modal-body").text(json_data);
ev.preventDefault();
});
Now, when someone clicks the submit button in the form, the modal window will open successfully! 🎉
📢 Call-to-Action:
We hope this guide helped you understand how to open a Bootstrap modal window using jQuery. If you found this information useful, don't forget to share it with your fellow developers! And if you have any further questions or need additional assistance, feel free to leave a comment below.
Happy coding! 💻💪