How to merge two arrays in JavaScript and de-duplicate items
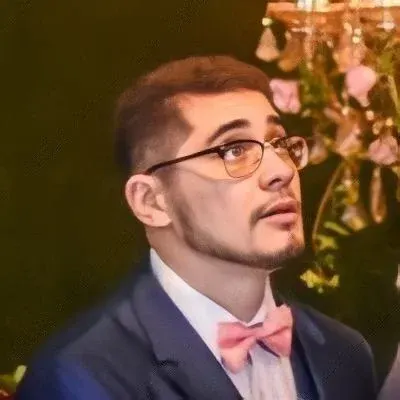
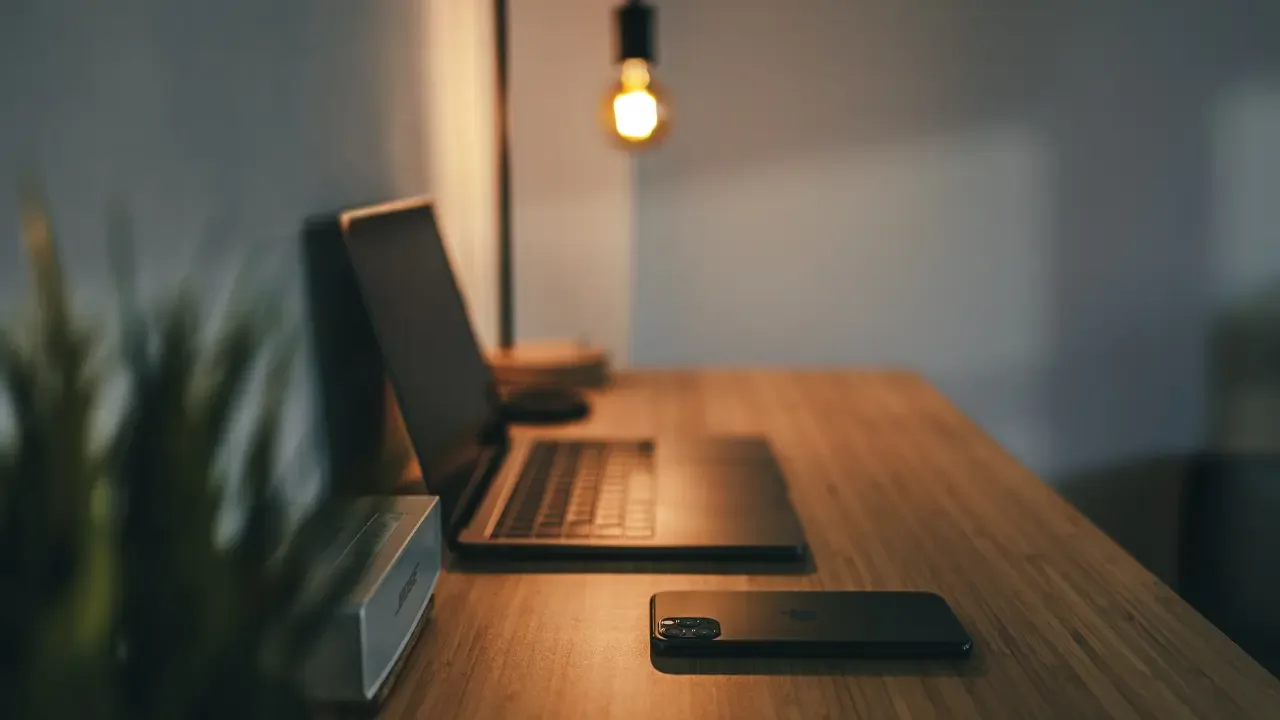
How to Merge Two Arrays in JavaScript and De-Duplicate Items
Introduction
In JavaScript, merging two arrays and removing duplicated items can be a common task. Whether you are working with data manipulation or building an application, this guide will walk you through an easy solution to this problem.
The Problem
Let's consider the following example:
var array1 = ["Vijendra", "Singh"];
var array2 = ["Singh", "Shakya"];
The goal is to merge array1
and array2
to obtain a new array array3
that contains all the unique items, preserving the original order. In this case, array3
should be:
["Vijendra", "Singh", "Shakya"]
Solution
To merge and de-duplicate the arrays, you can follow these steps:
Create an empty array to store the merged and de-duplicated items.
Concatenate
array1
andarray2
into a single array using theconcat()
method.Use the
filter()
method to remove duplicate items from the merged array.Return the de-duplicated array as the result.
Here's the JavaScript code that implements this solution:
var array1 = ["Vijendra", "Singh"];
var array2 = ["Singh", "Shakya"];
function mergeAndDeDuplicateArrays(array1, array2) {
var mergedArray = array1.concat(array2);
var deduplicatedArray = mergedArray.filter(function (item, index) {
return mergedArray.indexOf(item) === index;
});
return deduplicatedArray;
}
var array3 = mergeAndDeDuplicateArrays(array1, array2);
console.log(array3);
Explanation
The
concat()
method is used to mergearray1
andarray2
intomergedArray
.The
filter()
method is called onmergedArray
. It creates a new array by filtering out any item for which the index of its first occurrence is not equal to the current index. This removes duplicate items from the array.The de-duplicated array is returned as the result.
Conclusion
By following the steps outlined in this guide, you can effortlessly merge two arrays in JavaScript while removing duplicate items. You now have a handy function, mergeAndDeDuplicateArrays
, that you can reuse in your projects.
Feel free to experiment and apply this technique to your own scenarios. Happy coding! 💻🚀
Call-to-Action
If you found this guide helpful, make sure to share it with your fellow developers and leave a comment below sharing your thoughts or any additional tips you have on merging arrays in JavaScript. Let's learn together! 😊💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
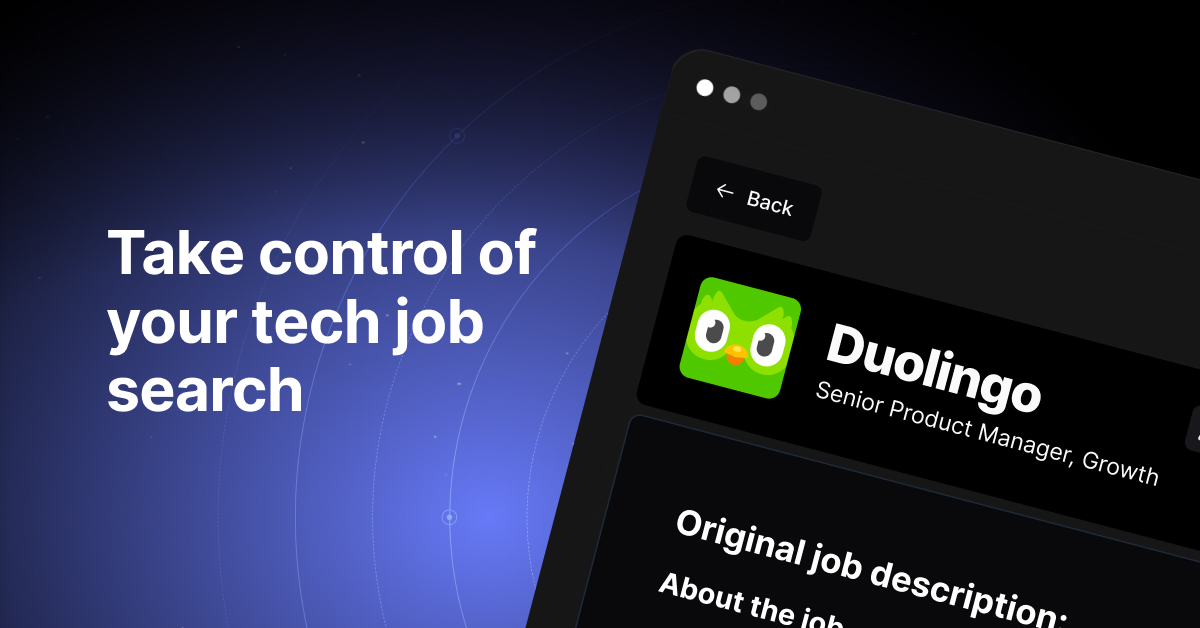