How to measure time taken by a function to execute
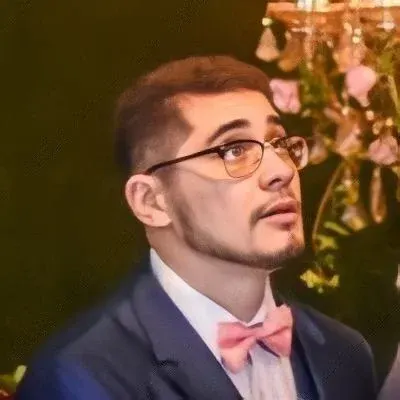
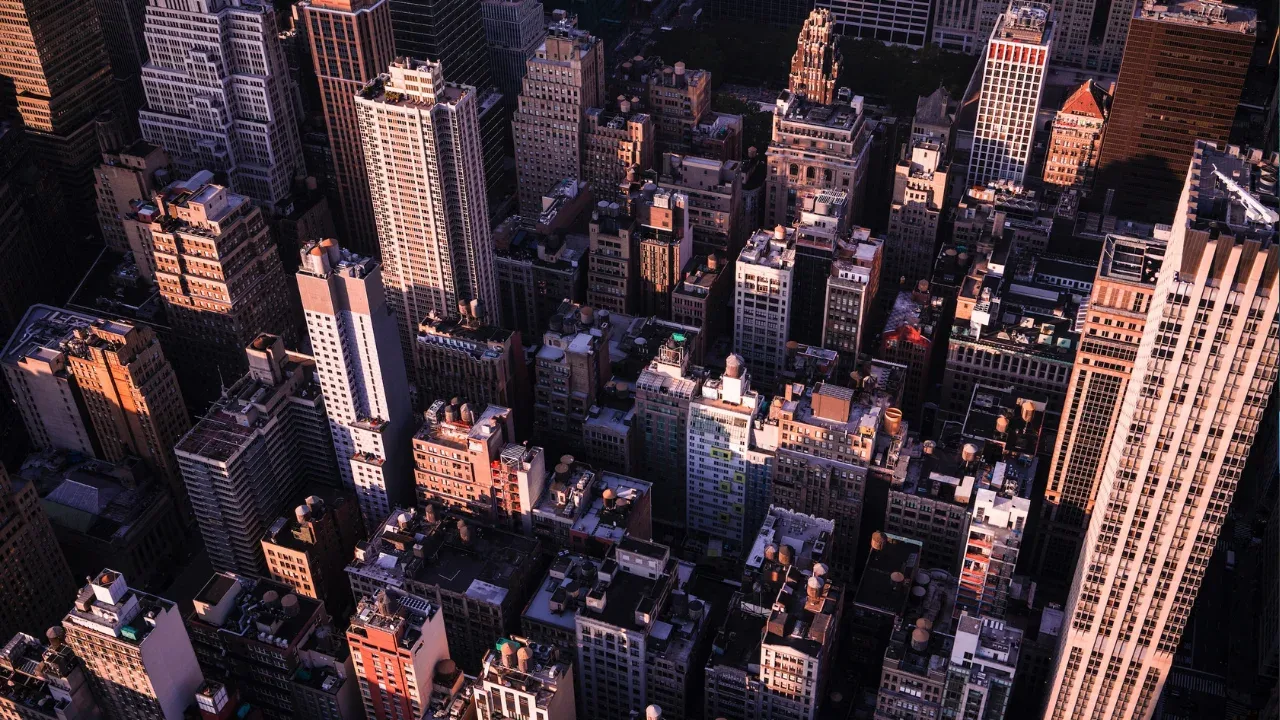
How to ⏱️ Measure Time Taken by a Function to Execute?
Have you ever wondered how long it takes for a function to complete its execution 🤔? Whether you're optimizing code or tracking performance, measuring the time taken by a function is crucial. In this post, we'll explore how to accomplish this in JavaScript and dive into the most appropriate methods to use.
The Evolution of Time Measurement ⌛
Once upon a time in 2008, developers commonly measured execution time using the new Date().getTime()
method. However, the landscape has changed, and now we have a more suitable alternative – the performance.now()
API. Let's see how we can utilize this API to measure function execution time accurately.
The Power of performance.now()
🚀
The performance.now()
method returns a high-precision timestamp indicating the number of milliseconds since the page started to load. This makes it perfect for measuring small time intervals, such as function execution duration. Here's an example of how to use it:
function measureExecutionTime() {
const startTime = performance.now();
// Code to measure execution time
const endTime = performance.now();
const executionTime = endTime - startTime;
console.log(`Function execution time: ${executionTime} milliseconds`);
}
In the code snippet above, we use performance.now()
to capture the starting and ending timestamps of the function. By subtracting the start time from the end time, we obtain the execution time in milliseconds. You can then utilize this value for optimization, logging, or other purposes.
Common 🤔 Issues and Easy Solutions 💡
1. Accuracy of Execution Time ⚖️
The accuracy of performance.now()
can vary depending on the hardware and browser implementation. In some cases, it might return a decimal value with a high precision, while in others, it could be rounded to the nearest millisecond. To ensure consistent results, we can use a polyfill like hrtime-performance
or a library like performance-now
. These tools provide a more accurate representation of execution time across different environments.
2. Avoiding Side Effects and Warm-Up Time 🥶
When measuring a function's execution time, it's essential to consider any potential side effects within the measured code. Some functions might have initialization or "warm-up" time, where they take longer to execute on initial calls but perform faster afterward. In such cases, it's recommended to run the function multiple times and calculate the average execution time, discarding any outliers.
Join the ⏲️ Measurement Revolution! 💪
Now that you have a solid understanding of how to measure the execution time of a function using performance.now()
, why not implement it in your code? You can use this technique for performance optimizations, benchmarking, or simply gaining insights into your code's behavior.
Make every millisecond count! 💥
Do you have any other cool tips for measuring function execution time? Share them in the comments below and join the conversation. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
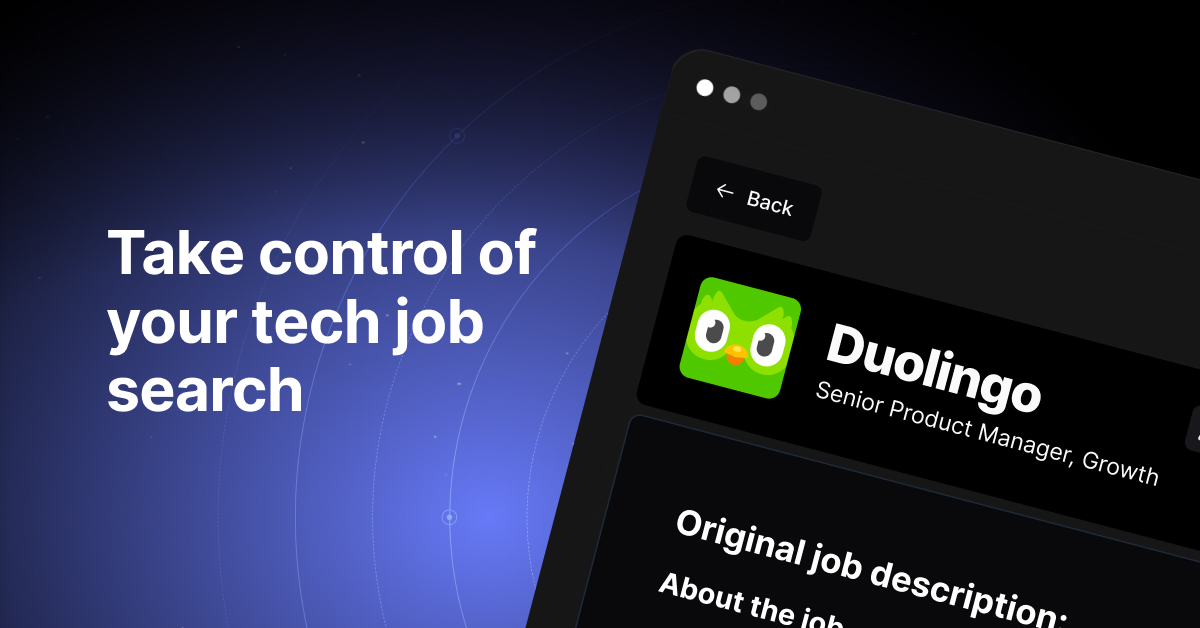