How to manage a redirect request after a jQuery Ajax call
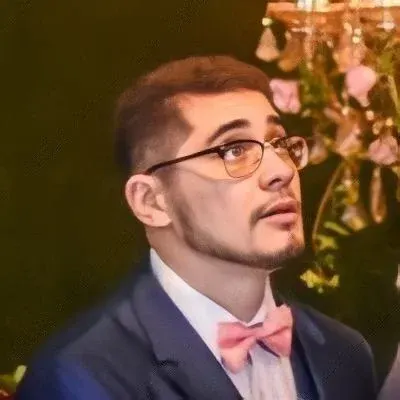
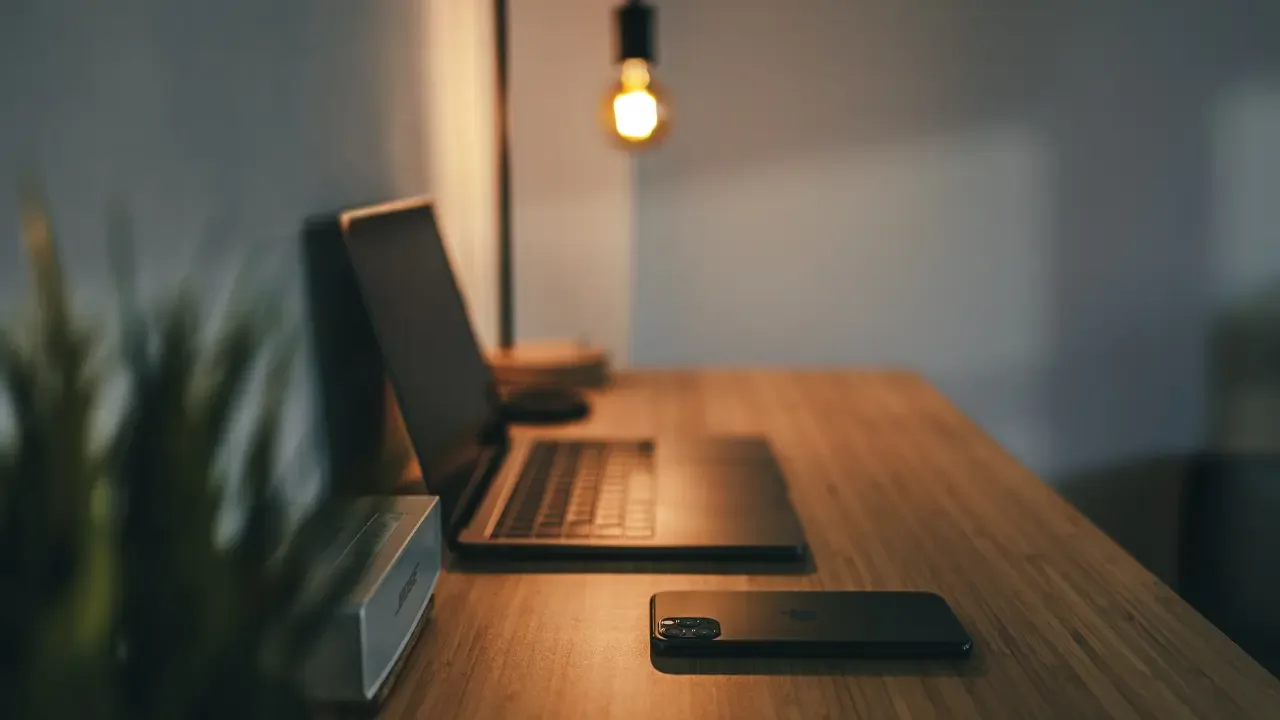
✨📝 How to Manage a Redirect Request After a jQuery Ajax Call 🚀
So, you're using the mighty and powerful jQuery Ajax to make cool on-page changes dynamically. But there's a twist! You've encountered the dreaded redirect directive, which has led your app to replace a beautiful div with the unsightly login page 😱 Fear not, fellow developer! In this guide, we'll explore common issues and provide easy solutions to help you gracefully handle redirect requests after a jQuery Ajax call and prevent your users from experiencing this unfortunate sight 🙅♂️
🤔 The Problem: Redirecting from an Ajax Call
You mentioned that you're using the $.post()
method to call a servlet using Ajax and then replacing the content of a div with the resulting HTML fragment. However, when the user's session times out, the server sends a redirect directive to navigate them to the login page. The issue arises when jQuery obediently replaces the div with the login page, resulting in a UX disaster. We need to find a way to intercept and manage this redirect request!
🛡️ Solution 1: Detect the Redirect and Handle It
One way to tackle this issue is by detecting the redirect from the server response and handling it accordingly. Here's a step-by-step breakdown:
Inside your Ajax success callback function, check the response headers for the presence of a "Location" header.
If the "Location" header exists, it means a redirect is happening.
You can then perform custom actions, such as displaying a friendly message to the user or redirecting them to a specific page of your choice.
Here's an example code snippet to put you on the right track:
$.post(yourUrl, yourData, function(response, status, xhr) {
if (xhr.getResponseHeader("Location")) {
// Handle the redirect here
// e.g., display a message or redirect the user
// to a custom page
alert("Session expired. Please login again.");
window.location.href = "/login";
} else {
// Process the Ajax response as usual
// e.g., replace the div with the HTML fragment
}
});
💫 Solution 2: Update Your Server-side Logic
Another way to solve this problem is by modifying your server-side logic to prevent the redirect when an Ajax request is made. Instead of sending a redirect directive, consider sending a specific response code or a JSON object that your client-side code can interpret and handle appropriately.
For example, you can update your servlet or backend code to return a JSON response with a custom status code indicating the session timeout. Your client-side code can then take action based on this status code, such as displaying a message or redirecting the user.
📣 Now It's Your Turn!
Handling redirect requests after a jQuery Ajax call doesn't have to be a nightmare. With the solutions provided above, you can manage redirect directives gracefully and improve the user experience of your app. Give them a try and let us know how it goes! 👍
🔗 Remember to engage with our tech community 🔗
Whether you're an experienced developer or just starting your coding journey, we'd love to hear your thoughts and experiences on managing redirect requests after a jQuery Ajax call. Have you encountered any unique challenges? Do you have alternative solutions to share? Leave a comment below and let's create an engaging conversation that will benefit everyone in the community! 🤝💬
Happy coding! 🎉👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
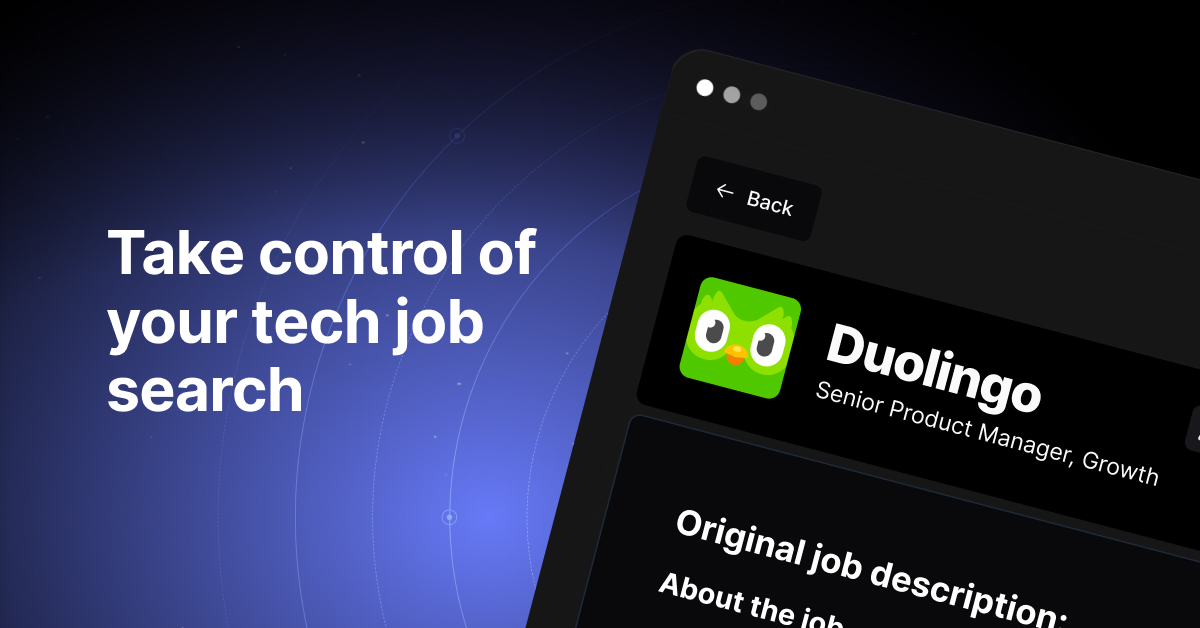