How to make Regular expression into non-greedy?
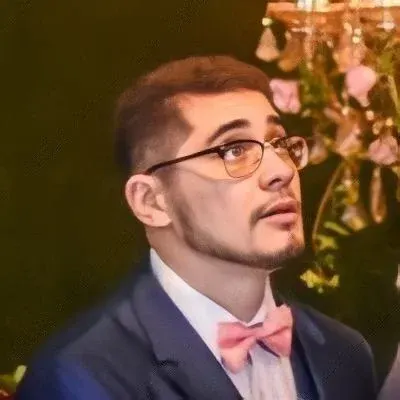
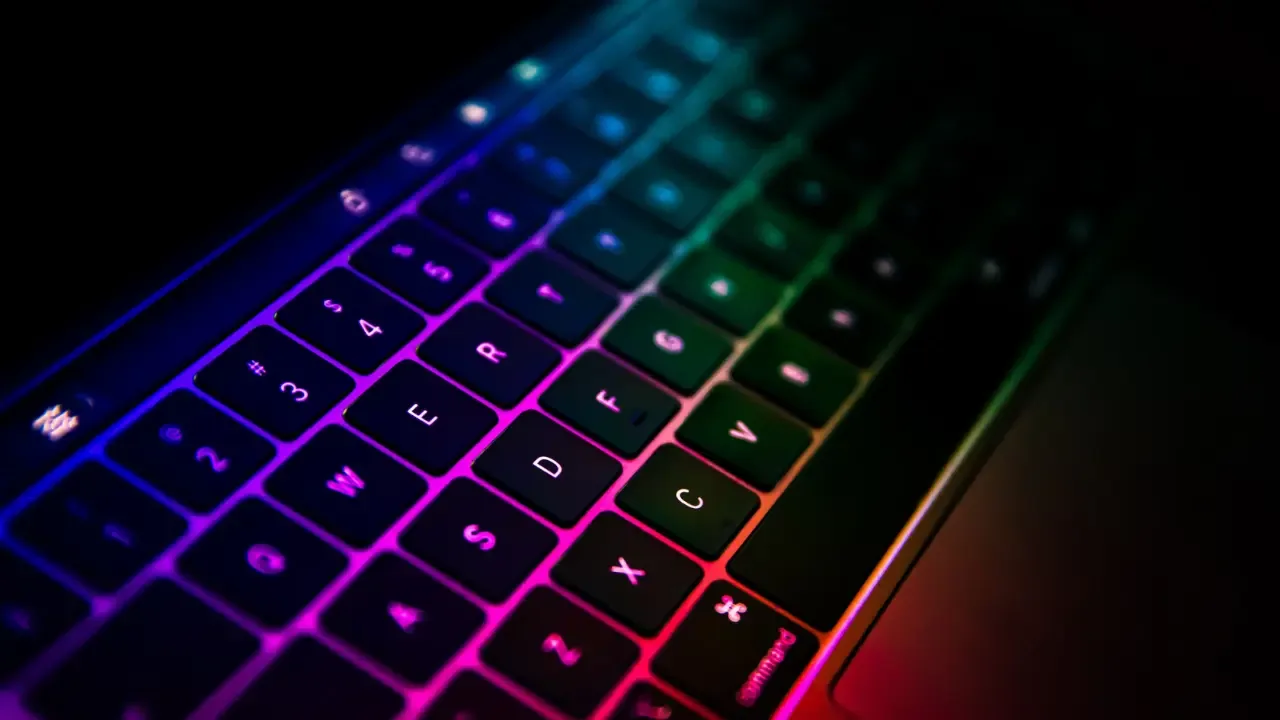
🔍 How to Make Regular Expression into Non-Greedy 🔍
Are you tired of getting unexpected results when using regular expressions? Do you want to learn how to make your regular expression non-greedy? Look no further! In this article, we'll walk you through the process and provide easy solutions to solve this common problem. Let's dive in! 💪
⚠️ The Problem: Let's imagine you are using jQuery and you have a string with a block of special characters (beginning and end). Your goal is to extract the text from that special character block. You decide to use a regular expression object for in-string finding. However, you encounter a challenge: how can you tell jQuery to find multiple results when there are two or more special characters blocks? 😕
Here's an example of HTML code that highlights the problem:
<div id="container">
<div id="textcontainer">
Cuộc chiến pháp lý giữa [|cơ thử|nghiệm|] thị trường [|test2|đây là test lần 2|] chứng khoán [|Mỹ|day la nuoc my|] và ngân hàng đầu tư quyền lực nhất Phố Wall mới chỉ bắt đầu.
</div>
</div>
And here's the JavaScript code you've written so far:
$(document).ready(function() {
var takedata = $("#textcontainer").text();
var filterdata = takedata.match(/(\[.+\])/);
alert(filterdata);
});
The result you get is [|cơ thử|nghiệm|] thị trường [|test2|đây là test lần 2|] chứng khoán [|Mỹ|day la nuoc my|]
. Unfortunately, this is not what you intended. So, how can we modify the regular expression to achieve the desired results? 🤔
✨ The Solution:
To make your regular expression non-greedy, you need to use the ?
modifier. By adding ?
after the .*
, you tell the regular expression to match as little as possible instead of matching as much as it can. Here's how the modified code looks:
var filterdata = takedata.match(/(\[.*?\])/g);
The g
flag at the end of the regular expression ensures that all matching results are returned. 🎉
💡 Explanation: Now, you might be wondering why this modification works. 🤔
In the original regular expression /(\[.+\])/
, the .+
is considered a greedy quantifier. It matches as many characters as possible, resulting in the longest possible match. In our case, it matches everything between the first [
and the last ]
, including the intermediate blocks.
By using the non-greedy .*?
in /(\[.*?\])/g
, we are telling the regular expression to match as few characters as possible, resulting in individual matches for each special character block. This way, we get the desired outcome. 🌟
💭 Further Questions: While we have provided a solution to your specific problem, you might still have some lingering questions. We're here to help! Feel free to reach out to us if you have any further queries or are seeking clarification. 😊
📣 Call to Action: Did you find this article helpful? Are you curious about exploring more regex-related topics? Head over to our blog for more tech guides and handy tips! Don't forget to share this article with your friends or colleagues who might find it useful. 👍
Now go forth and make your regular expressions non-greedy like a pro! 💪🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
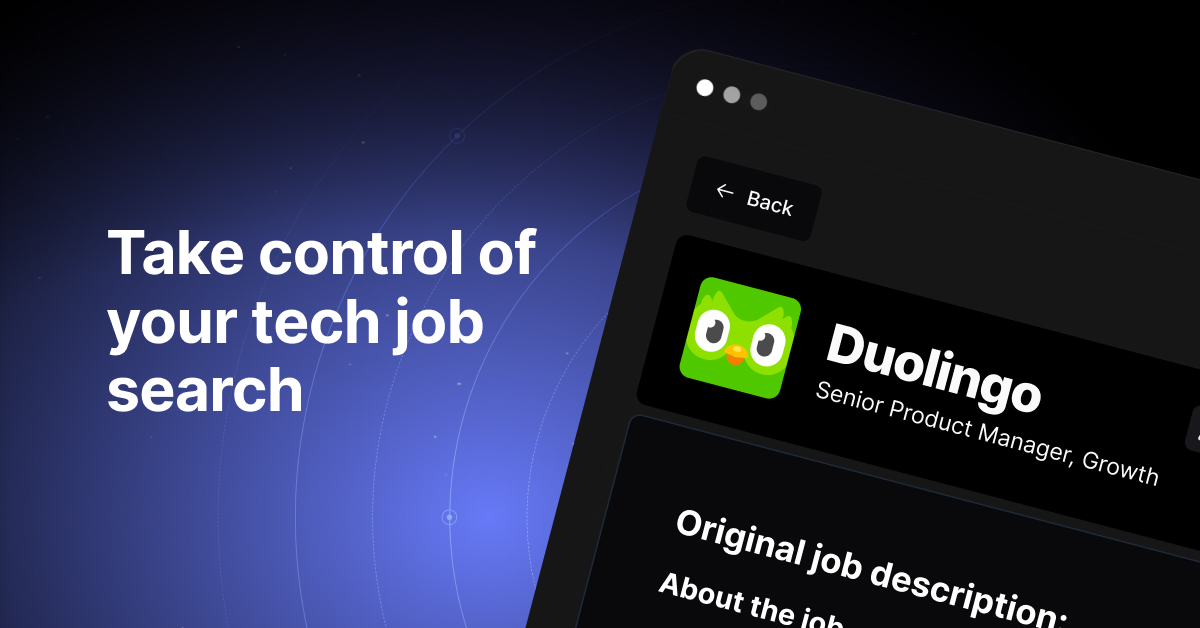