How to make JavaScript execute after page load?
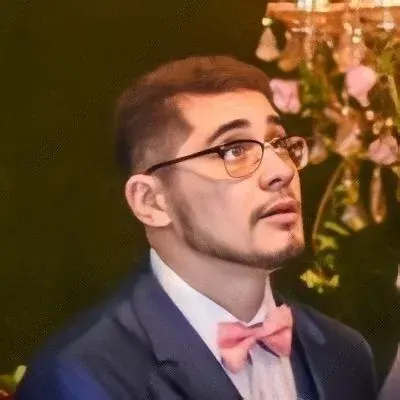
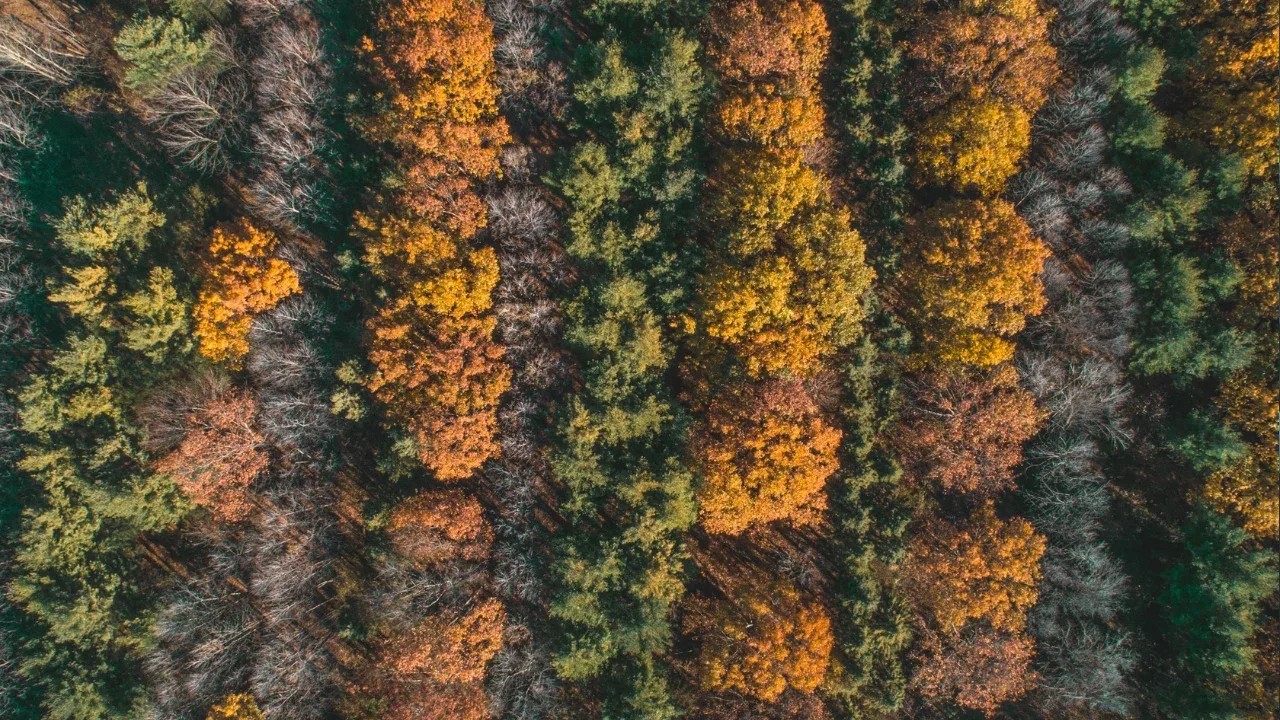
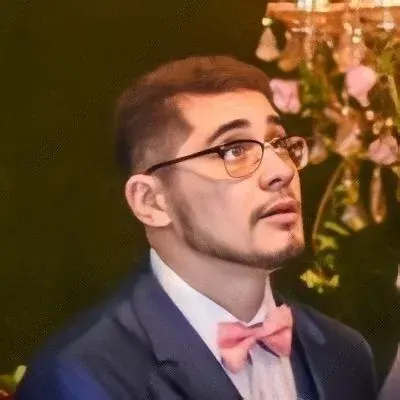
šŖ How to Make JavaScript Execute After Page Load? šŖ
š Introduction: We've all been there. You write some JavaScript code that manipulates the DOM, only to find out that it's not working because the script executes before the page has finished loading. š© Fret not, my friend! In this guide, I'll show you some easy solutions to ensure that your JavaScript code runs only after the page has fully loaded. Let's dive in! šāāļø
š Identifying the Problem:
The scenario described above highlights a common issue faced by developers. When JavaScript code sits inside the <head>
tag, it often executes before the document is fully loaded. This limitation prevents you from accessing elements in the <body>
or performing other essential operations. š¢ So, what can be done? š¤
š§ Solution 1: Using the window.onload
Event:
One way to ensure your JavaScript code runs after the page has finished loading is by utilizing the window.onload
event. This event fires when all the HTML content, including images and external resources, has been fully loaded. To implement this solution, simply wrap your code within the window.onload
event handler, like this:
window.onload = function() {
// Your JavaScript code goes here...
};
By doing this, you guarantee that your code won't execute until the whole page has loaded, giving you unhindered access to the entire DOM. š
š§ Solution 2: Utilizing the DOMContentLoaded
Event:
Another alternative is to use the DOMContentLoaded
event. This event triggers as soon as the HTML structure is fully parsed and ready for manipulation. Unlike window.onload
, this event doesn't wait for external resources like images to finish loading. Here's an example of how to use it:
document.addEventListener("DOMContentLoaded", function() {
// Your JavaScript code goes here...
});
By leveraging this event, your code will execute as soon as it can safely interact with the DOM, providing a better user experience. š
š£ Call-to-Action: Now that you have a couple of solutions up your sleeve, go ahead and implement them in your code. Experiment with both options and see which one suits your specific needs. Remember, happy developers create happy websites! š
š Share your success stories or any other tips you might have in the comments below. Let's join forces and help each other overcome JavaScript execution challenges!
Happy coding! š»š