How to loop through a plain JavaScript object with the objects as members
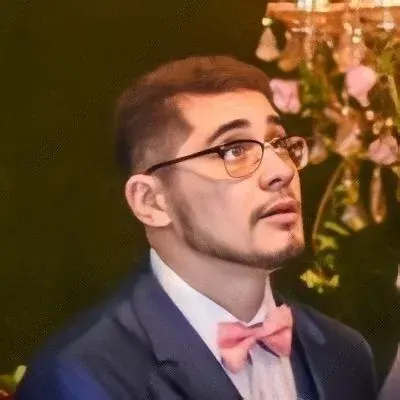
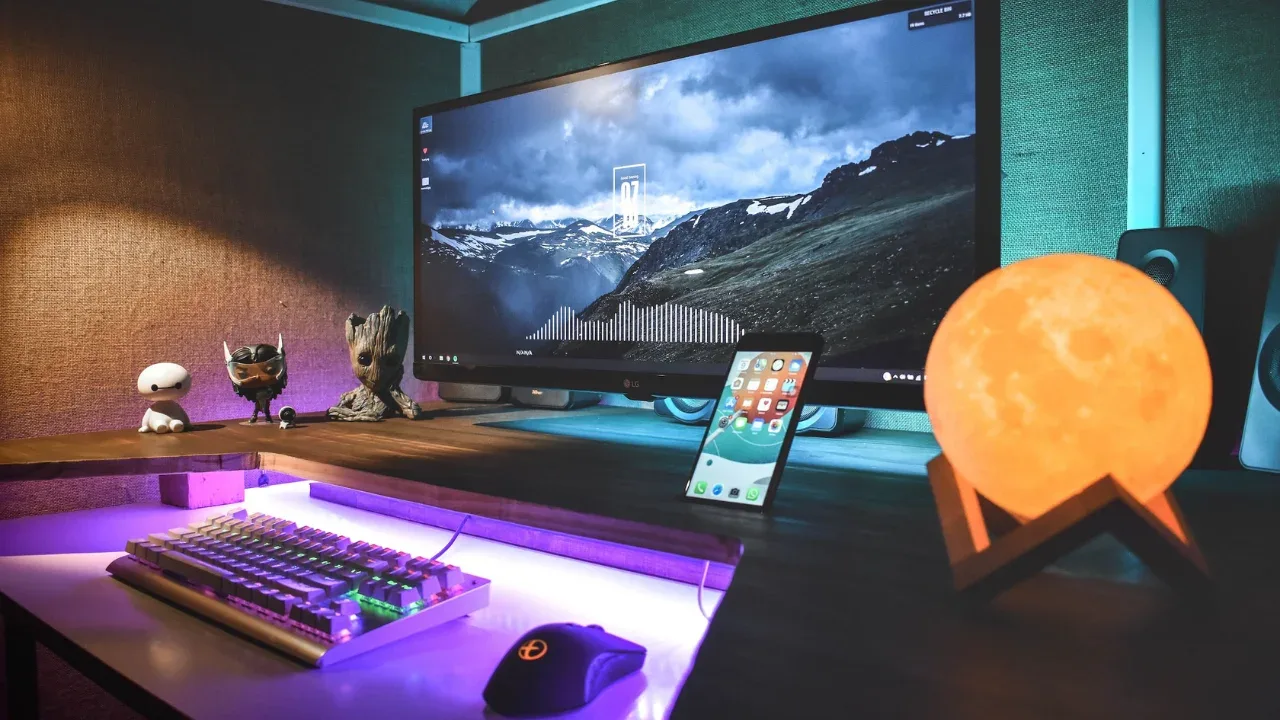
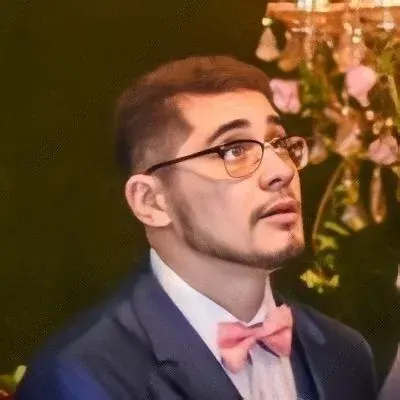
How to Loop Through a JavaScript Object with Nested Objects 🔄
So, you have a JavaScript object with objects as its members, and you want to loop through them. This can be a bit tricky, but worry not! In this blog post, we'll address this common issue, provide easy solutions, and empower you with the knowledge to become a looping pro! 🔥
The Object Looping Challenge 🧩
Let's take a look at the example you provided. We have a JavaScript object called validation_messages
, which contains multiple keys, each representing a unique validation message. Each key has an associated object with properties like your_name
and your_msg
.
Solution #1: Using the for...in Loop 🔄
One way to loop through this object and access the nested objects' properties is by using the for...in
loop. This loop allows us to iterate over each key in the object and access its value.
for (var key in validation_messages) {
if (validation_messages.hasOwnProperty(key)) {
var nestedObject = validation_messages[key];
console.log(nestedObject.your_name);
console.log(nestedObject.your_msg);
}
}
In this solution, we iterate over each key in the validation_messages
object. We use the hasOwnProperty
method to check if the key is a direct property of the object, filtering out any inherited properties. Then, we access the nested object using the current key and log its properties.
Solution #2: Using Object.entries() Method 🗝️
Another newer and more concise solution is to use the Object.entries()
method. This method transforms an object into an array of key-value pairs, allowing us to easily loop through them using methods like forEach()
or a traditional for
loop.
Object.entries(validation_messages).forEach(([key, value]) => {
console.log(value.your_name);
console.log(value.your_msg);
});
In this solution, we convert the validation_messages
object into an array of key-value pairs using Object.entries()
. Then, we use the forEach()
method to iterate over each pair. Inside the loop, we can directly access the nested object's properties using value.your_name
and value.your_msg
.
🎉 Conclusion and Call-to-Action 🚀
You've made it to the end of this looping adventure! Now you know two powerful ways to loop through a JavaScript object with nested objects. Whether you prefer the traditional for...in
loop or the modern Object.entries()
method, the choice is yours.
Next time you encounter a similar problem, remember these solutions and save yourself the headache of figuring it out from scratch. Happy coding! 💻✨
Now it's time for you to apply your newfound knowledge! Share with us in the comments section below which solution you prefer and why. Or if you have any other cool looping techniques, we'd love to hear about them too! Let's keep the conversation going! 🗣️💬