How to load external scripts dynamically in Angular?
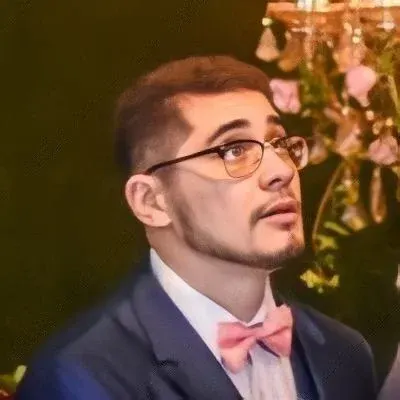
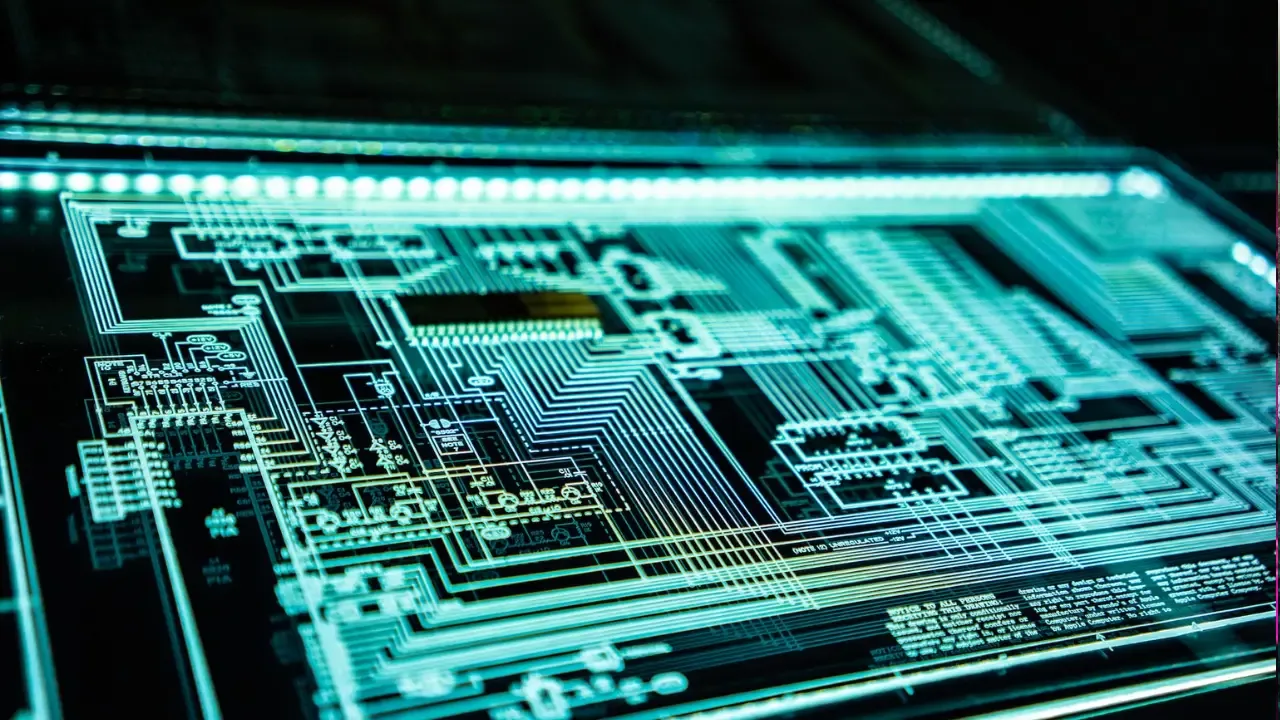
š How to Load External Scripts Dynamically in Angular?
š” Do you ever find yourself needing to load external scripts dynamically in your Angular application? Perhaps you want to keep your code modular and componentized, without directly adding the <script>
tag into your index.html. This blog post will guide you through common issues and provide easy solutions for dynamically loading external scripts in Angular.
š¤ The Dilemma š„
The dilemma arises when you have a module that requires an external library, but you want to avoid directly adding the <script>
tag into the index.html file. This could be due to various reasons, such as keeping your codebase clean and modular, or needing to switch between loading the script from a CDN or a local folder.
š The Solution ā”
To load external scripts dynamically in Angular, you can make use of the ScriptLoaderService
, a utility service that allows you to programmatically add script tags to the document. Here's how you can do it:
Install the
scriptjs
library by running the following command:
npm install scriptjs
scriptjs
is a lightweight library that helps with loading scripts dynamically.
Create a new service called
ScriptLoaderService
:
import { Injectable } from '@angular/core';
import { Observable, Observer } from 'rxjs';
import * as scriptjs from 'scriptjs';
@Injectable({
providedIn: 'root'
})
export class ScriptLoaderService {
private scripts: { [url: string]: { loaded: boolean; subject: Observer<any> } } = {};
loadScript(url: string): Observable<any> {
if (!this.scripts[url]) {
this.scripts[url] = { loaded: false, subject: null };
this.scripts[url].subject = new Observable((observer: Observer<any>) => {
this.scripts[url].subject = observer;
});
}
if (!this.scripts[url].loaded) {
scriptjs(url, () => {
this.scripts[url].loaded = true;
this.scripts[url].subject.next();
this.scripts[url].subject.complete();
});
}
return this.scripts[url].subject;
}
}
Inject the
ScriptLoaderService
into your component:
import { Component, OnInit } from '@angular/core';
import { ScriptLoaderService } from 'path/to/script-loader.service';
@Component({
selector: 'my-app',
template: `Your template goes here`
})
export class MyAppComponent implements OnInit {
constructor(private scriptLoaderService: ScriptLoaderService) {}
ngOnInit() {
this.scriptLoaderService.loadScript('http://external.com/path/file.js')
.subscribe(() => {
// Script loaded successfully
}, () => {
// Script failed to load
});
}
}
That's it! You have now successfully loaded an external script dynamically in your Angular application. The ScriptLoaderService
allows you to easily load scripts from both CDNs and local folders by simply changing the URL.
š¬ Engage with the Community šŖ
We hope this guide helps you overcome any challenges you may have faced while loading external scripts dynamically in Angular. If you have any questions or suggestions, feel free to leave a comment below or reach out to our community on [social media platform]. We'd love to hear from you and see how you're utilizing this technique!
š Conclusion š
Dynamically loading external scripts in Angular can be a powerful tool in keeping your code modular and componentized. By using the ScriptLoaderService
, you can easily load scripts from both CDNs and local folders, providing flexibility and scalability to your application. So go ahead, give it a try, and see how it can enhance your Angular development experience!
Keep coding, keep innovating! āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
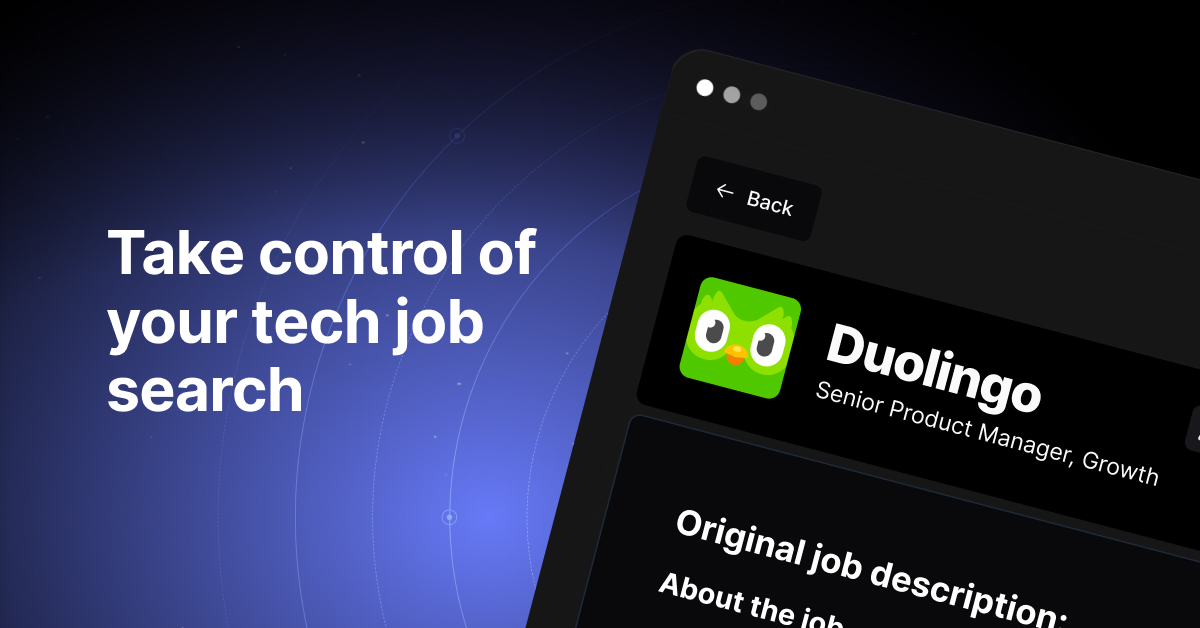