How to load all server side data on initial vue.js / vue-router load?
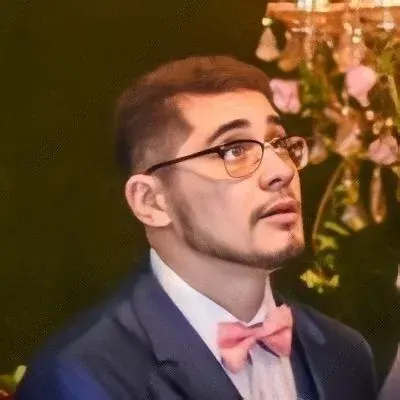
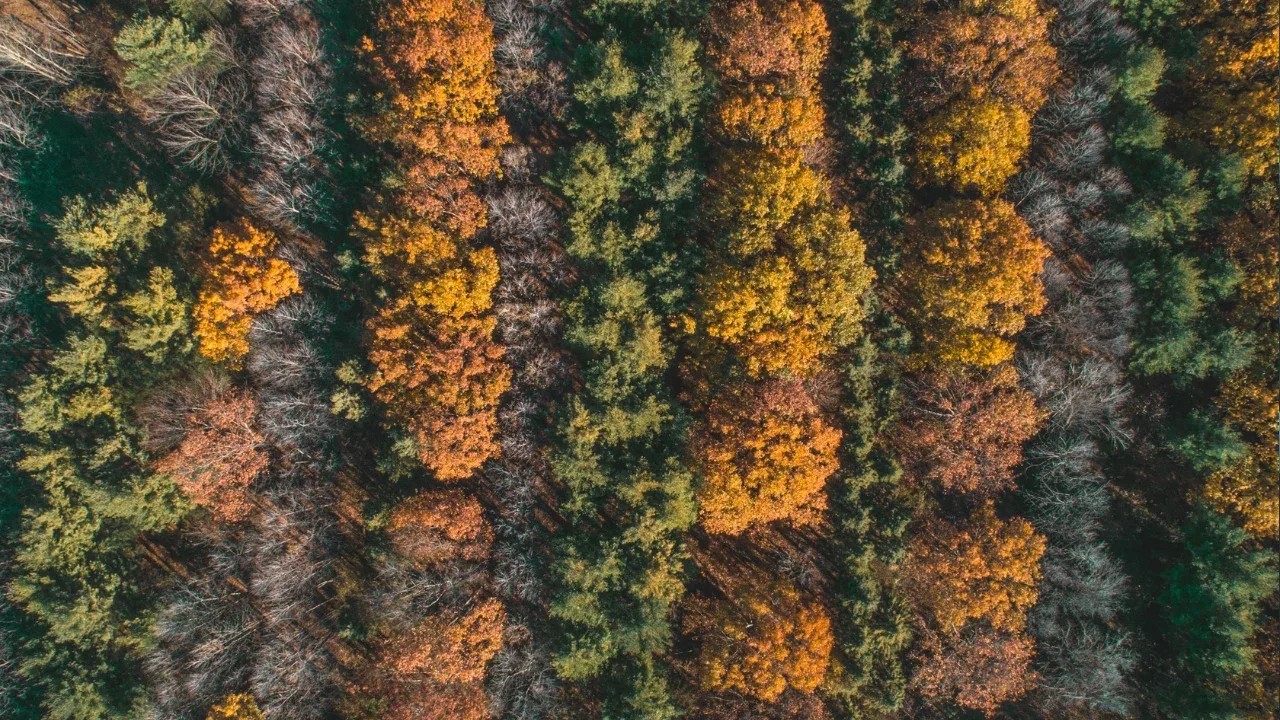
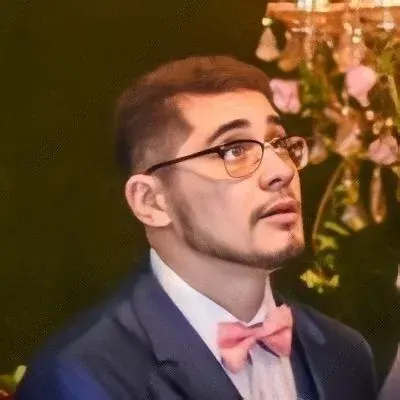
📝 How to Load All Server Side Data on Initial Vue.js/Vue Router Load
Are you facing the issue of your server side data loading only after the page has rendered when using Vue.js and vue-router? This can be frustrating, especially when you want to load all route and page data on the initial page load. But fret not, we've got you covered!
🌐 The Problem
As mentioned in the context, there's a single-page site built with Vue.js and vue-router, making use of the WordPress REST API. However, the data loads only after the page has rendered when making an AJAX call to the server using the REST API. This can lead to delayed data display and a poor user experience.
💡 The Solution
To load all server side data on the initial page load, we need to make a few changes to our code.
Update main.js Router Code:
In the
created()
method of your main.js file, make the AJAX call to fetch the data from the server. Instead of directly assigning the response tothis.acfs
, we will create a Promise that resolves when the data has loaded. This way, we can ensure that the route components receive the data before rendering.const app = new Vue({ router, data: { acfs: '' }, created() { const loadData = () => { return new Promise((resolve, reject) => { $.ajax({ url: 'http://localhost/placeholder/wp-json/acf/v2/page/2', type: 'GET', success: function(response) { console.log(response); // Assign the response to acfs data property this.acfs = response.acf; resolve(); }.bind(this), error: function(error) { reject(error); } }); }); }; // Call the loadData function and wait for the promise to resolve loadData().then(() => { // Once the data has loaded, mount the Vue app this.$mount('#app'); }).catch((error) => { console.error(error); }); } });
Update Home.vue Component Code:
In your Home.vue component, update the code to access the data from the
acfs
property of the parent component.export default { name: 'about', data () { return { acf: this.$parent.acfs, } }, }
🎉 Engage with Us!
Now that you know how to load all server side data on initial Vue.js/vue-router load, it's time to level up your Vue.js game! If you found this guide helpful, share it with your fellow developers and encourage them to join the Vue.js community. Feel free to leave comments below if you have any questions or want to share your own experiences. Happy coding! 👩💻👨💻
Reference: Vue.js Documentation - Data Fetching