How to interpolate variables in strings in JavaScript, without concatenation?
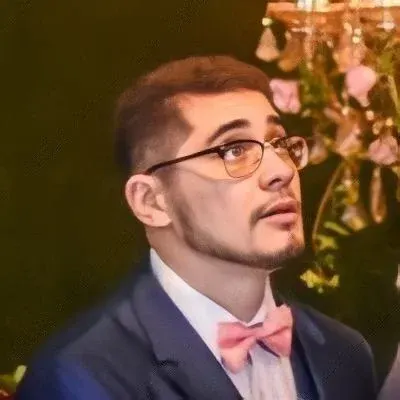
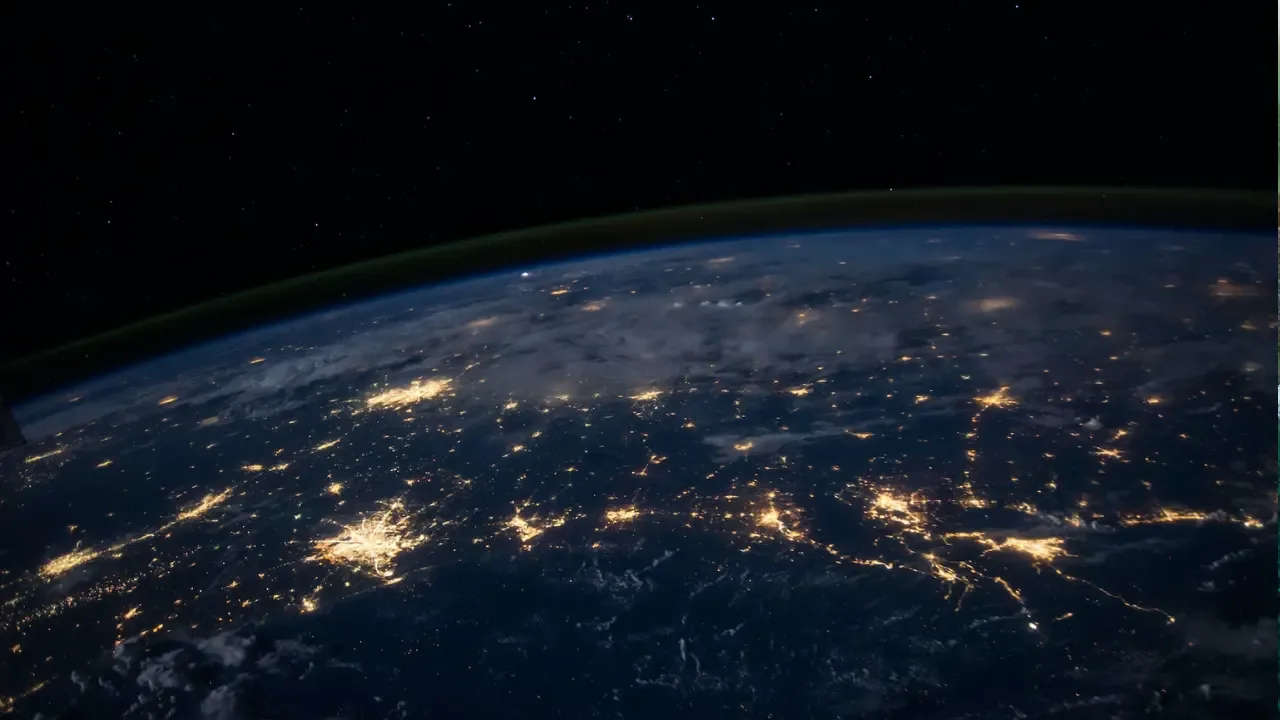
How to Interpolate Variables in Strings in JavaScript, without Concatenation? 🌟
JavaScript is a versatile and powerful programming language that allows you to work with strings in various ways. One common requirement is the need to interpolate variables within strings without using the traditional concatenation approach.
In other programming languages like PHP, variable interpolation is possible using a similar syntax where variables are directly embedded within double-quoted strings. However, JavaScript handles string interpolation in a slightly different manner.
The Traditional Way: Concatenation
Before we dive into the world of string interpolation in JavaScript, let's briefly touch upon the traditional approach of concatenation.
Here's an example:
const hello = 'foo';
const myString = 'I pity the ' + hello;
The output of myString
would be 'I pity the foo'
. While this works perfectly fine, it can quickly become cumbersome and less readable, especially when dealing with multiple variables or complex string constructions.
The Modern Solution: Template Literals
JavaScript introduced template literals in ECMAScript 6 (ES6) as a concise and elegant way to interpolate variables within strings. They utilize backticks (`) instead of single or double quotes and offer greater flexibility compared to concatenation.
Let's take a look at how it works using the same example:
const hello = 'foo';
const myString = `I pity the ${hello}`;
With template literals, we wrap the string within backticks and embed variables using the ${variable}
syntax. In this case, the output of myString
would still be 'I pity the foo'
.
Template literals not only allow variable interpolation but also support multiple lines and the inclusion of expressions. This makes them more versatile and powerful than concatenation alone.
Dealing with Complex Expressions
Template literals can also handle complex expressions, enabling you to perform calculations or call functions within the interpolated section. Here's an example:
const num1 = 10;
const num2 = 5;
const result = `${num1} + ${num2} is ${num1 + num2}`;
The result
variable would contain the string '10 + 5 is 15'
, where the expression ${num1 + num2}
is evaluated and the sum of num1
and num2
is displayed within the string.
💡 Quick Takeaways:
JavaScript introduced template literals as a modern way to interpolate variables within strings.
Template literals use backticks (`) instead of single or double quotes.
Variables are embedded using the
${variable}
syntax.Template literals can handle complex expressions and enable calculations or function calls within the interpolated section.
By utilizing the power of template literals, you can create more concise, readable, and flexible code when it comes to string interpolation in JavaScript.
So, go ahead and give template literals a try in your next JavaScript project and experience the beauty of string interpolation without concatenation!
If you found this guide helpful, make sure to share it with your fellow JavaScript enthusiasts. And if you have any other cool JavaScript tips or tricks up your sleeve, feel free to leave a comment below. Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
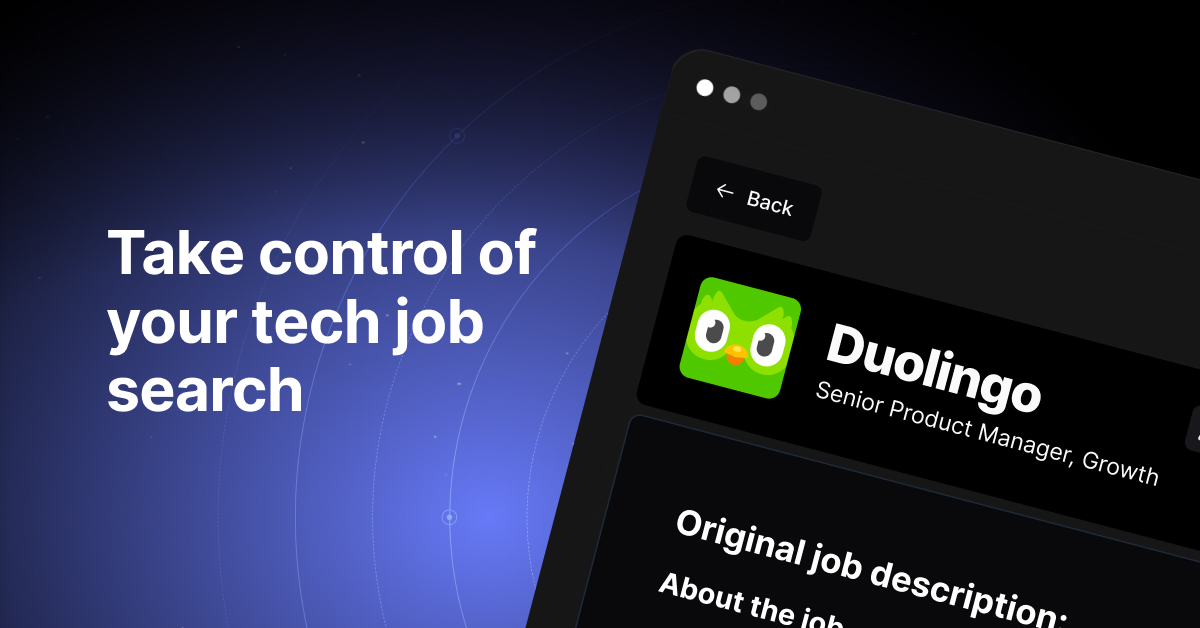