How to intercept all AJAX requests made by different JS libraries
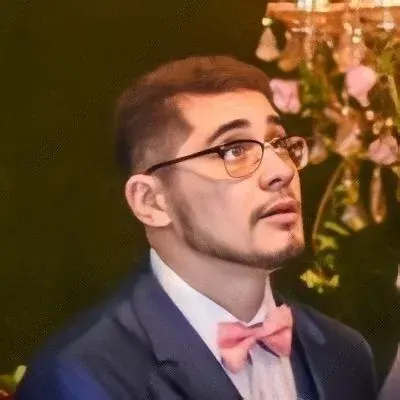
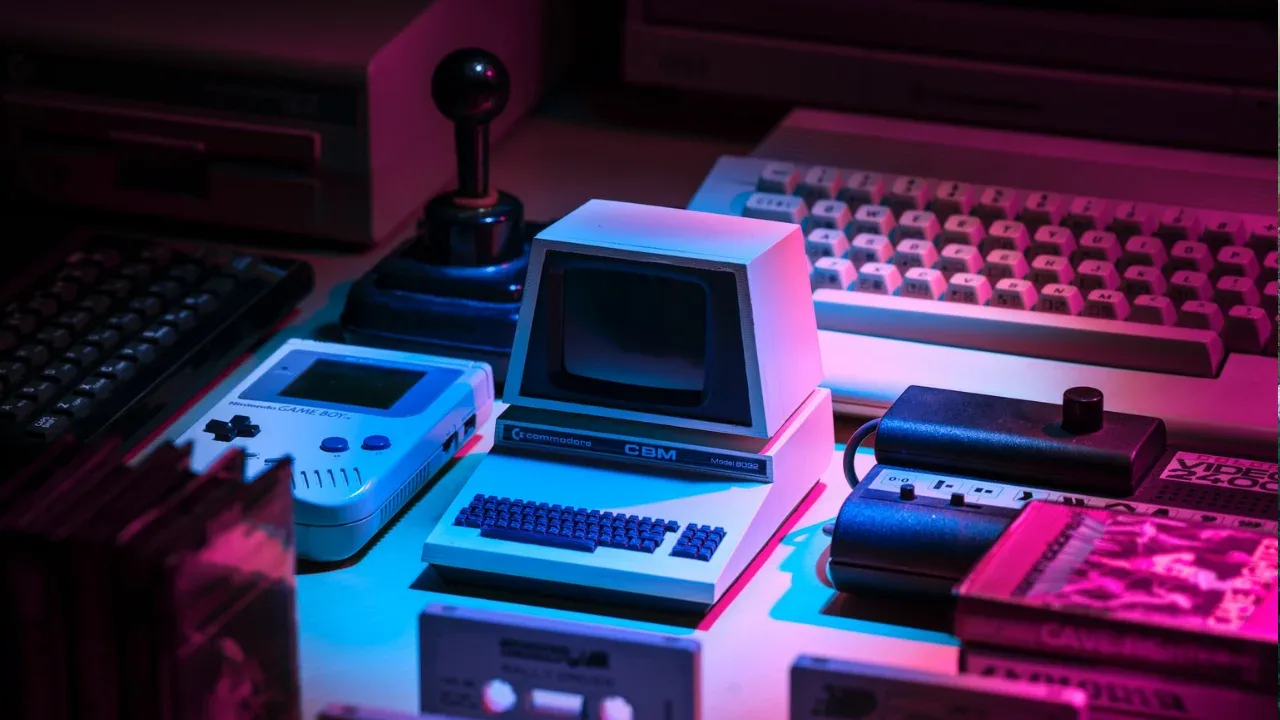
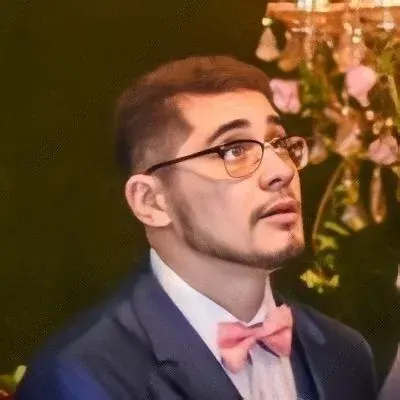
Intercepting AJAX Requests Made by Different JS Libraries: A Complete Guide
Are you building a web app with multiple JS libraries like AngularJS and OpenLayers? Do you need to intercept all AJAX responses to handle scenarios such as session expiration? We've got you covered! In this guide, we'll show you how to intercept all AJAX requests made by different JS libraries and redirect users to the login page when necessary. 🚀
The Challenge
The challenge arises when different JS libraries handle AJAX requests in different ways. While AngularJS provides interceptors for managing such scenarios, it's not as straightforward with libraries like OpenLayers. This guide will focus on achieving AJAX request interception using vanilla JS, ensuring compatibility across various libraries and applications.
Intercepting AJAX Responses
To intercept AJAX responses, we can modify the XMLHttpRequest
prototype. This approach allows us to add a listener to the readystatechange
event, where we can check the response status and perform necessary actions. Here's an example of how to intercept AJAX responses:
(function(open) {
XMLHttpRequest.prototype.open = function(method, url, async, user, pass) {
this.addEventListener("readystatechange", function() {
if (this.readyState === 4 && this.status === 401) {
// Redirect user to the login page
window.location.href = "/login";
}
}, false);
open.call(this, method, url, async, user, pass);
};
})(XMLHttpRequest.prototype.open);
In the code above, we override the open
method of the XMLHttpRequest
prototype. Within the overridden method, we add an event listener to the readystatechange
event. When the response state reaches 4
(indicating completion) and the status is 401 Unauthorized
, we redirect the user to the login page.
Please note that this code snippet has been adapted from Stack Overflow and has been tested in the latest version of Google Chrome.
Performance and Safety Considerations
You might wonder if modifying the prototype of XMLHttpRequest
could result in dangerous or performance issues. While this approach is generally safe, it's essential to understand potential implications.
Modifying the prototype affects all instances of XMLHttpRequest
, not just those used within specific libraries. Therefore, it's crucial to thoroughly test the behavior in different scenarios and on various browsers before implementing it in a production environment.
Regarding performance, the additional event listener introduces a minimal overhead. However, the impact is negligible for most modern web apps, especially when dealing with occasional AJAX responses.
Updating the Solution: Intercepting Requests Before Sending
What if you want to inject additional headers into AJAX requests before they get sent? Here's an update to the previous solution:
(function(send) {
XMLHttpRequest.prototype.send = function(data) {
// Inject headers before sending the request
if(accessToken) {
this.setRequestHeader('x-access-token', accessToken);
}
send.call(this, data);
};
})(XMLHttpRequest.prototype.send);
In the code above, we override the send
method of the XMLHttpRequest
prototype. Within the overridden method, we check if an accessToken
exists and then use setRequestHeader
to inject the access token as a header into the request.
This updated solution allows you to intercept requests before sending them and modify headers as required.
Conclusion and Call to Action
Intercepting AJAX requests made by different JS libraries is now within your grasp! By following the techniques and code snippets in this guide, you can easily intercept AJAX responses and requests, ensuring smooth handling of scenarios like session expiration.
Remember to thoroughly test the implementation in different scenarios and browsers to ensure compatibility and performance. Feel free to share your experiences, ask questions, or suggest alternative solutions in the comments below. Let's make web app development a breeze together! 💪