How to insert an item into an array at a specific index?
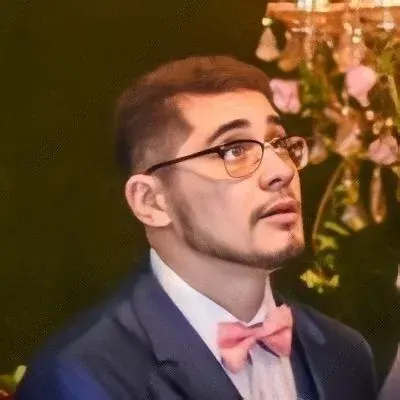
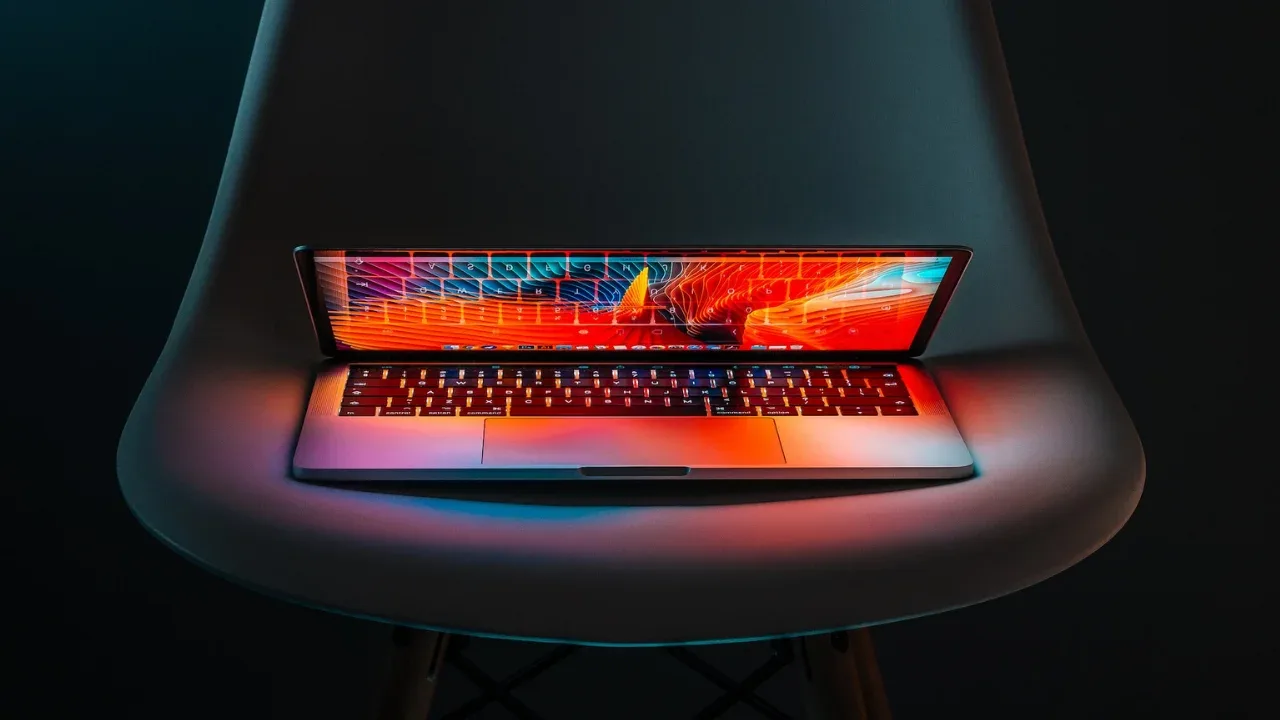
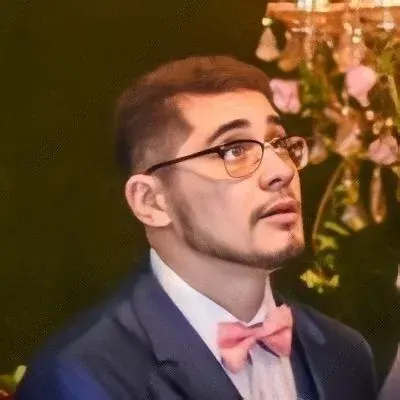
π’βοΈ**Hey techies! You ever wondered how to insert an item into an array at a specific index?**π€π»
I've got your back! In this blog post, I'll show you some easy solutions using the JavaScript programming language. Whether you're a jQuery aficionado or prefer pure JavaScript, I've got you covered! πͺπ
πFirst things first, let's understand the problem. The question is asking for a JavaScript array insert method, using the syntax arr.insert(index, item)
. The goal is to insert an item at a particular index in an array. Pretty neat!π‘
Now, let's dive into the nitty-gritty details. Here are three different approaches to insert an item into an array at a specific index, each with its own pros and cons. Choose the one that suits your needs!π§βοΈ
π₯ Approach 1: Using the splice()
method
This method allows us to modify an array by adding or removing elements. Here's how you can use it to insert an item at a specific index:
arr.splice(index, 0, item);
In the above code snippet, arr
is the target array, index
is the position where you want to insert the item
, and item
is the value you want to insert.
β Pros: The inserted item becomes part of the original array, and this method is widely supported.
β Cons: It mutates the original array, and if you only want to insert a single item, it might be an overkill.
π₯ Approach 2: Using the spread operator (...
)
Take advantage of ES6 and spread the existing array into two parts, with the new item in the middle:
arr = [...arr.slice(0, index), item, ...arr.slice(index)];
In this approach, arr
is reassigned with a new array. The spread operator ...
concatenates the array slices.
β Pros: The original array remains unchanged, and you have more control over the insertion process.
β Cons: Requires more code compared to the splice()
method, and it might not work in older versions of JavaScript.
π₯ Approach 3: Using a custom function
If you prefer to follow the arr.insert(index, item)
syntax, or if you're a big fan of jQuery, you can create your own method:
Array.prototype.insert = function(index, item) {
this.splice(index, 0, item);
};
With this custom function, you can use the insert()
method on any array, just like you've imagined! π©π«
β Pros: It gives you a reusable method, following the desired syntax.
β Cons: Modifying the prototype of built-in objects like Array
may lead to conflicts in larger codebases.
πβ¨ Conclusion
Congratulations! You're now equipped with three different ways to insert an item into an array at a specific index in JavaScript. It's time to unleash your coding powers and elevate your array manipulation skills!πͺπ‘
π Now it's your turn! Which method are you most excited to try? Have you encountered any other alternative ways? Share your thoughts and experiences in the comments below! Let's keep the discussion going!π£οΈπ
π If you found this blog post helpful, don't forget to share it with your fellow developers and give it a thumbs up! Sharing is caring!πβ€οΈ
π Stay connected! Join our coding community for more tech tips and tricks like this. Follow us on Twitter, Facebook, and Instagram. Remember, the best way to learn is by sharing and exploring together!π₯π»
Happy coding!ππ
Disclaimer: The code examples provided are simplified for clarity and may not cover all special cases. It's always a good practice to consider edge cases and handle errors in real-world scenarios.