How to initialize an array"s length in JavaScript?
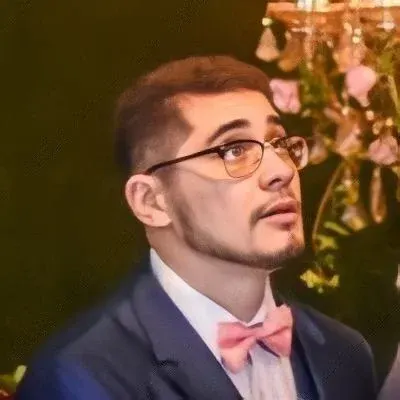
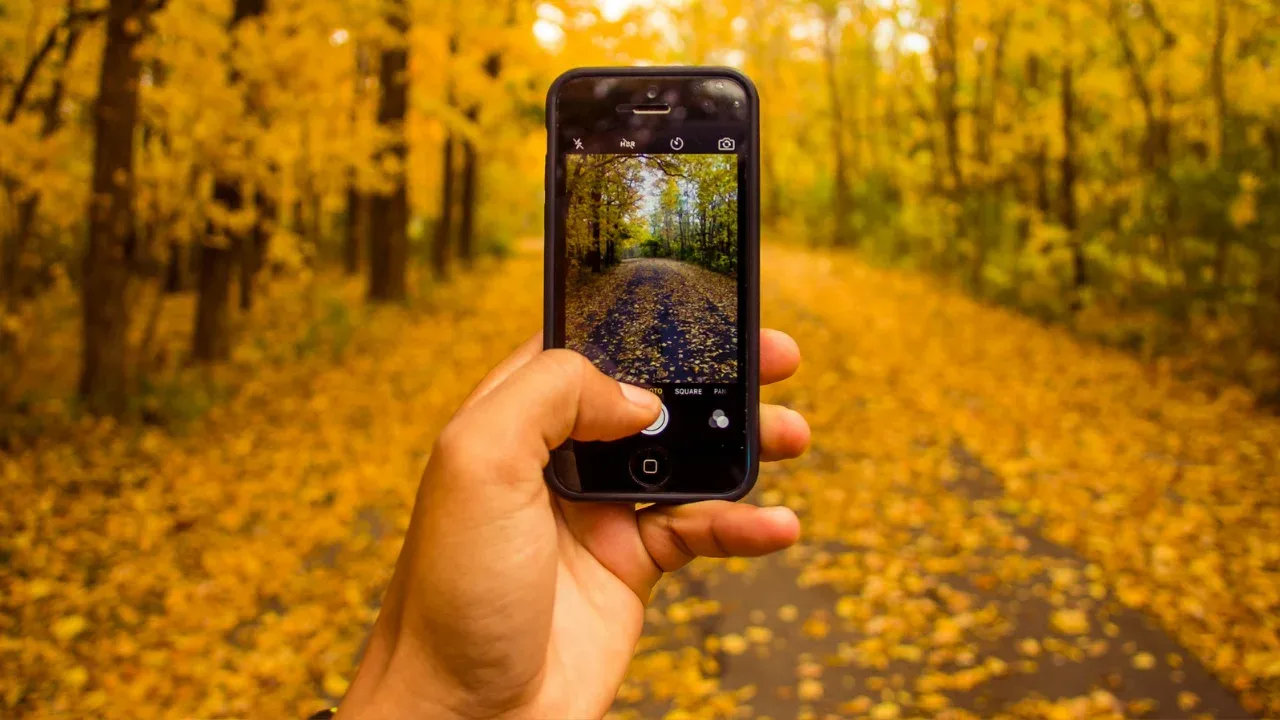
How to Initialize an Array's Length in JavaScript 📚
Have you ever encountered issues when trying to initialize the length of an array in JavaScript? 🤔 If so, you're not alone! Commonly used tutorials, like those from w3schools and devguru, often suggest using the syntax var test = new Array(4)
to set the length of an array. However, this approach may lead to unexpected errors when running your code through linters like jsLint. 😱
Why Does jsLint Prefer the []
Syntax? 🤷♀️
According to the explanation provided by jsLint, it favors the square bracket syntax []
over new Array()
because it improves code readability and avoids potential pitfalls. 📖
By using []
, you make it clear that you're declaring an array explicitly. On the other hand, new Array()
can also be used to create objects, leading to confusion and ambiguous code. 😕
Potential Risks of Using new Array()
🛑
While using new Array()
to initialize an array's length may work in most modern browsers, it's not recommended, as it can introduce human errors and lead to less maintainable code. Additionally, it may cause compatibility issues in older browsers or JavaScript engines.
Therefore, it's best to follow the industry-standard approach and use []
instead.
Setting an Array's Length in One Line 🚀
If you've decided to switch to the square bracket syntax []
, you might be wondering if there's a way to declare an array and set its length all in one line. The answer is YES! 😊
Here's an example of how you can achieve this:
const test = new Array(4).fill(undefined);
By using the fill()
method, we can initialize an array with a specific length and fill it with a default value, which is undefined
in this case. You can also specify a different default value if needed.
Alternatively, you can do it in two lines, like this:
const test = [];
test.length = 4;
While this approach is not as concise, it still gets the job done. 💪
Get Rid of Potential Errors by Embracing Best Practices 🛠️
To summarize, it's recommended to use []
syntax to initialize an array and set its length in JavaScript. By doing so, you can avoid unexpected errors, improve code readability, and adhere to industry best practices. 😎
So, what are you waiting for? Go ahead and update your code to use the square bracket syntax, unleash your JavaScript skills, and create awesome arrays! 🚀
Have you encountered any other JavaScript issues or have questions? Let us know in the comments below! 👇 We're here to help! 💙
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
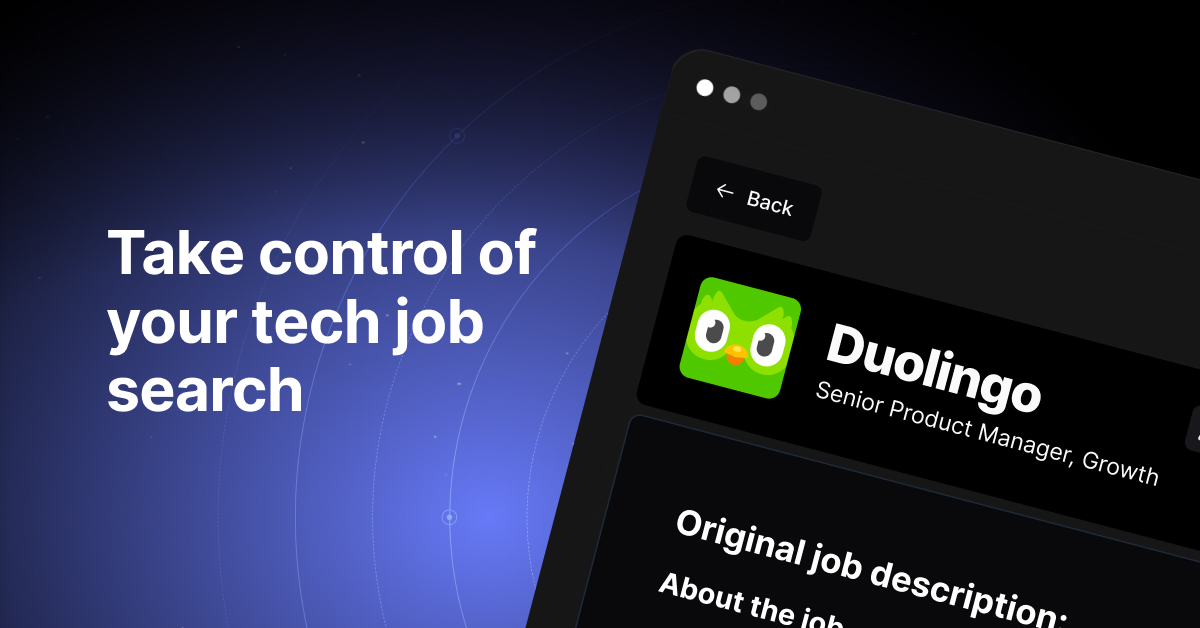