How to handle the `onKeyPress` event in ReactJS?
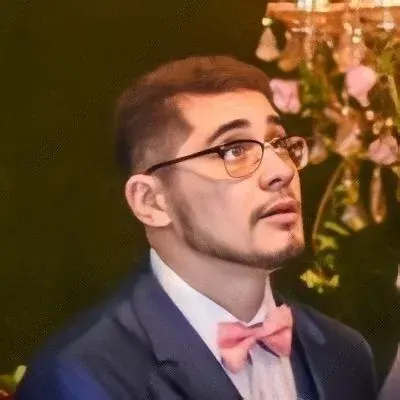
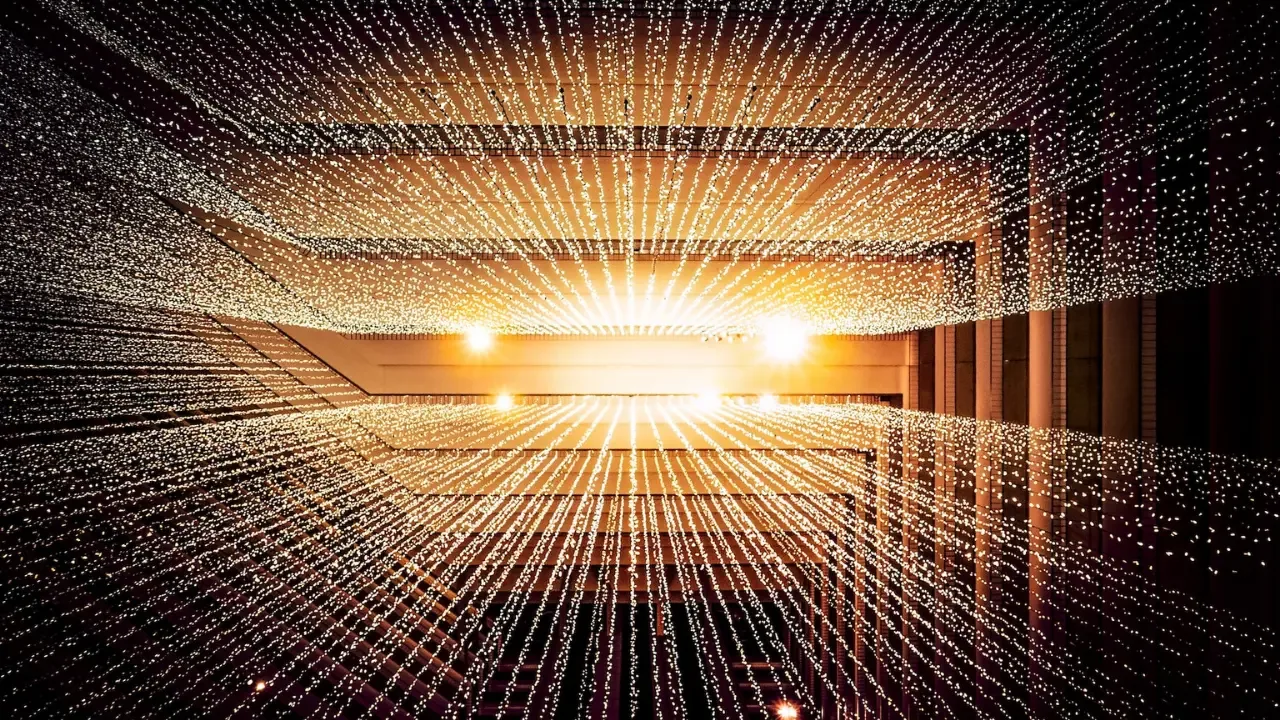
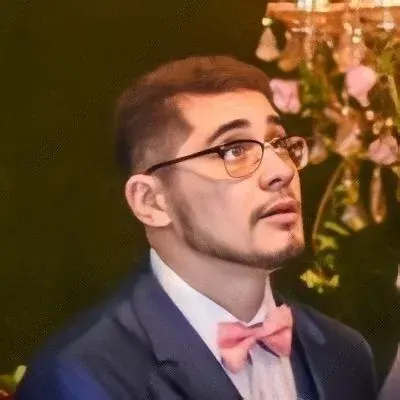
🔛🔠How to handle the onKeyPress
event in ReactJS? 🔠🔛
Hey there tech enthusiasts! 👋 Today, we're going to tackle a common issue in ReactJS - how to handle the onKeyPress
event. Specifically, we'll be looking at a scenario where we want to trigger an alert when the user presses the enter key (keyCode = 13). Let's dive right in and find an easy solution! 💪🔍
The first step is to define a function that will handle the event. In our example, we'll call it add
. Inside this function, we'll check if the keyCode is equal to 13 (which represents the enter key). If it is, we'll show an alert saying "Adding...". Here's the initial code snippet to get us started:
var Test = React.createClass({
add: function(event){
if(event.keyCode == 13){
alert('Adding....');
}
},
render: function(){
return(
<div>
<input type="text" id="one" onKeyPress={this.add} />
</div>
);
}
});
React.render(<Test />, document.body);
Now, let's break down what's happening in our code. We have a React component called Test
that contains a function add
. Inside the add
function, we pass in the event
object as a parameter. This object contains information about the event that was triggered, including the keyCode.
Next, we use an if
statement to check if the keyCode
is 13. If it is, we use the alert
function to display the message "Adding...".
In the render
method, we return a JSX snippet that consists of a div
containing an input
element. We attach the onKeyPress
event to the input
element and pass in the add
function as the event handler.
Now, when the user presses any key while the input
element is in focus, the add
function will be triggered. However, the alert will only show up when the enter key (keyCode = 13) is pressed.
👉 Pro Tip: If you want to perform additional actions in addition to showing the alert, you can simply add your code inside the if
statement in the add
function.
And that's it, folks! You now know how to handle the onKeyPress
event in ReactJS and trigger an alert when the enter key is pressed. 🎉💻
But, wait! The journey doesn't end here. There's always more to learn and explore. Share your React experiences and let us know how you're using the onKeyPress
event in your projects. Leave a comment below and join the conversation! 👇✍️
Happy coding! 💻🚀