How to get the value of an input field using ReactJS?
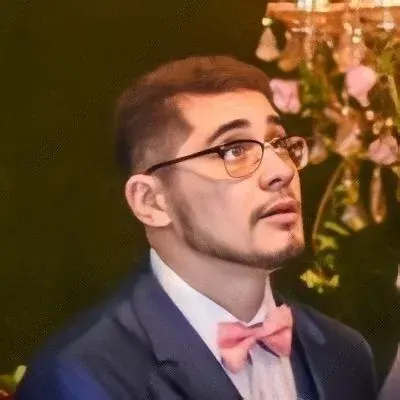
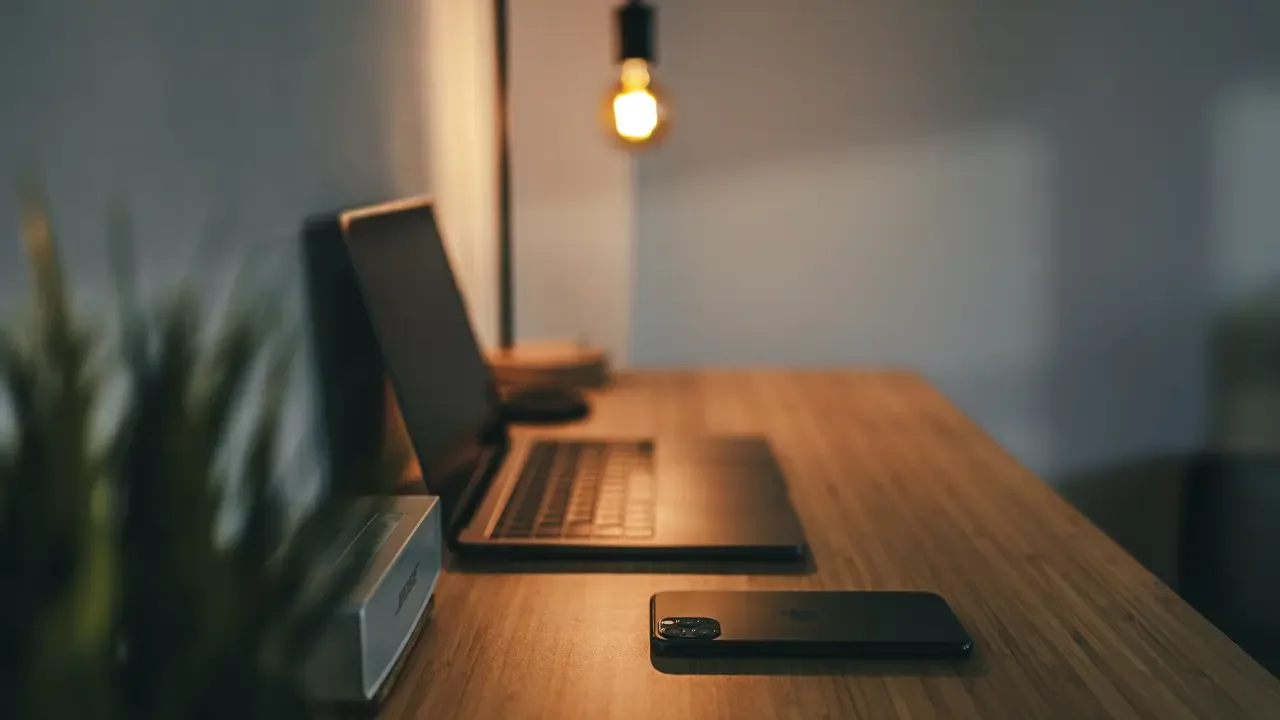
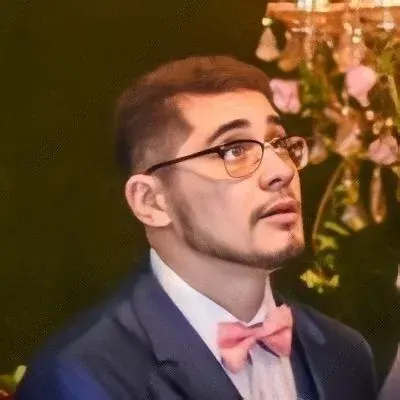
🔥🤔 How to Get the Value of an Input Field using ReactJS? 🔥🤔
Hey there, tech enthusiasts! 👋 In today's blog post, we'll address a common issue many ReactJS developers face: retrieving the value of an input field. 📝
Let's dive right into the code snippet you've shared. 🏊♂️ We have a React component called MyComponent
, which includes a form with an input field and a save button. The goal is to get the value of the input field when the save button is clicked. But wait, there's a problem! 😱
When the onSubmit
function is triggered, the console logs undefined
instead of the expected value. So, what's wrong with the code? Let's find out! 🕵️♀️
The issue lies in how the input field value is being accessed. In the provided code, the input field is assigned a ref using the ref
attribute and an inline callback function. Here's the important part:
<input type="text" className="form-control" ref={(c) => this.title = c} name="title" />
When we try to access this.title
within the onSubmit
function, it should refer to the actual input element, right? Wrong! 🙅♂️
The ref callback function receives the DOM element as an argument (c
in this case), but assigning it to this.title
is just storing a reference to the DOM element, not its value. Hence, when we log this.title
, it is undefined
. 😞
But worry not, fellow developer! 💪 We have a couple of easy solutions to overcome this issue. Let's check them out!
Option 1: Using Controlled Components 🎛️
Controlled components in React are the ones whose values are controlled by React, rather than the DOM. In this case, we can leverage React's state
to keep track of the input field's value. Here's how we can modify our code:
export default class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
title: '' // Initial value of the input field
};
}
handleInputChange(e) {
this.setState({ title: e.target.value });
}
onSubmit(e) {
e.preventDefault();
var title = this.state.title; // Access the value from the state
console.log(title);
}
render() {
return (
// ...
<form className="form-horizontal">
{/* ... */}
<input
type="text"
className="form-control"
value={this.state.title}
onChange={this.handleInputChange}
name="title"
/>
{/* ... */}
</form>
// ...
);
}
}
By binding the value
attribute of the input field to this.state.title
and handling the onChange
event with the handleInputChange
method, we create a controlled component. Now, when the onSubmit
function is triggered, we can access the value of the input field from this.state.title
. 🙌
Option 2: Using Uncontrolled Components with Refs 📝
If you prefer to keep your input field uncontrolled, we can still make a small adjustment to the code to achieve our desired result.
export default class MyComponent extends React.Component {
constructor(props) {
super(props);
this.titleRef = React.createRef();
}
onSubmit(e) {
e.preventDefault();
var title = this.titleRef.current.value; // Access the value directly from the ref
console.log(title);
}
render() {
return (
// ...
<form className="form-horizontal">
{/* ... */}
<input
type="text"
className="form-control"
ref={this.titleRef}
name="title"
/>
{/* ... */}
</form>
// ...
);
}
}
Here, instead of using the inline callback function, we create a ref using React.createRef()
inside the component's constructor. We then assign this ref to the input field using the ref
attribute, and access its value directly with this.titleRef.current.value
inside the onSubmit
function. Problem solved! 🎉
Remember, both options will return the value of the input field correctly when the save button is clicked.
📢 Now it's your turn! 📢
Have you encountered similar issues while working with ReactJS? How did you solve them? Share your experience and let's learn from each other in the comments section below. 👇
That's all for today's blog post, folks! We hope you found this guide helpful in getting the value of an input field using ReactJS. 😊
Until next time, happy coding! 💻✨